filmov
tv
Writing Allocation Free Code in C# - Matt Ellis
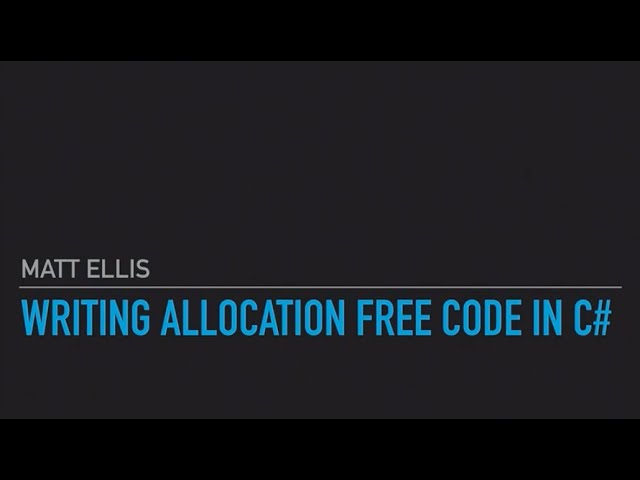
Показать описание
Performance is a feature. We all want our code to run faster, and there are plenty of ways to do this - caching, using a smarter algorithm or simply doing less stuff.
In this session, we’re not going to look at any of that. Instead, we’re going to focus on a recent trend in the C# world - improving performance by reducing memory allocations. We’ll see how recent versions of C# allow using structs without creating lots of copies, and we’ll have a timely reminder on exactly what is the difference between a class and a struct. We’ll also spend some time with the new Span<T> runtime type and find out how that can help work with slices of existing memory, and how it’s already into the types we know and love in the framework. And of course, we’ll take a look at when you should and (more importantly) shouldn’t use these new techniques.
Check out more of our talks in the following links!
NDC Conferences
In this session, we’re not going to look at any of that. Instead, we’re going to focus on a recent trend in the C# world - improving performance by reducing memory allocations. We’ll see how recent versions of C# allow using structs without creating lots of copies, and we’ll have a timely reminder on exactly what is the difference between a class and a struct. We’ll also spend some time with the new Span<T> runtime type and find out how that can help work with slices of existing memory, and how it’s already into the types we know and love in the framework. And of course, we’ll take a look at when you should and (more importantly) shouldn’t use these new techniques.
Check out more of our talks in the following links!
NDC Conferences
Комментарии