filmov
tv
Java: Rounding Numbers (Math.round(), DecimalFormat & printf)
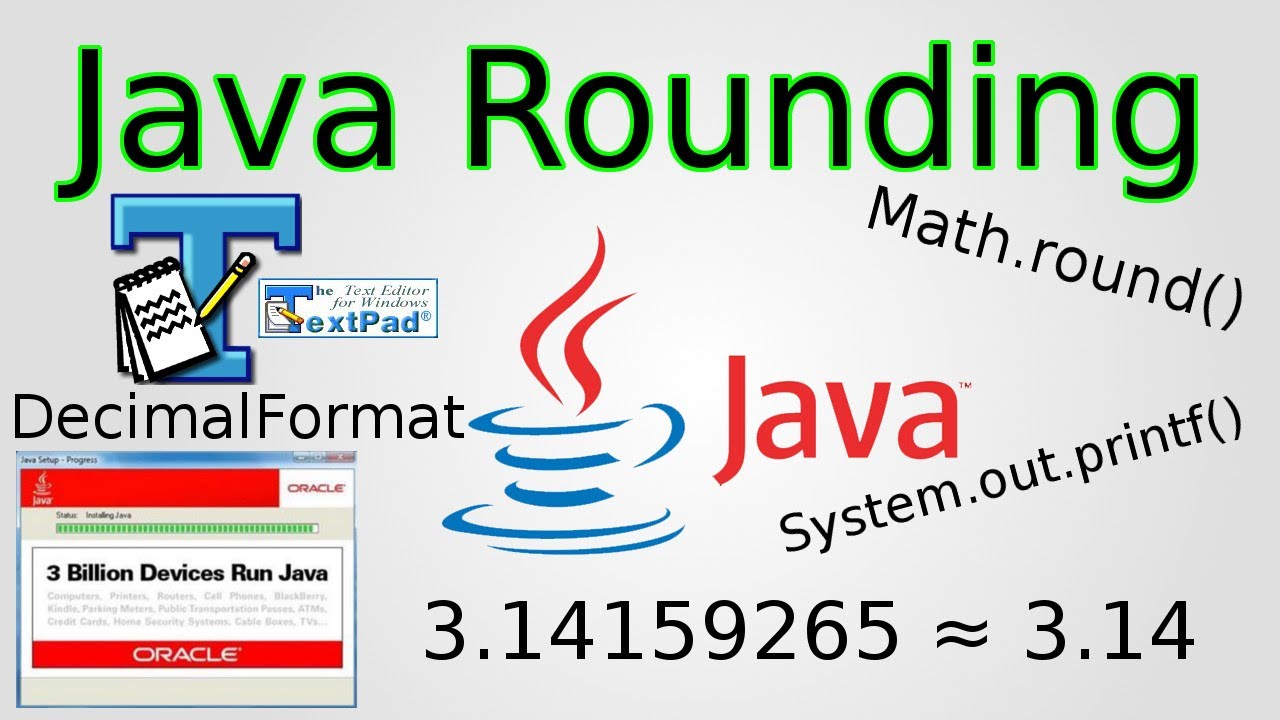
Показать описание
Java enables you to do almost anything, especially tasks involving numbers. But sometimes complicated calculations give you an answer with way too many decimals.
Most practical applications only require a few decimals. Here are 3 ways to round number in Java:
Java's Math class is inherently included in every program you create so there is not need for an import statement. The round() method takes a number as an argument and rounds that number to the nearest integer. On it's own this isn't spectacularly helpful but you can combine it with an easy little trick.
NOTE: The printf method doesn't change the actual contents of the variable. After using printf, the variable still has all the decimals.
3) Using DecimalFormat
Then create a reference variable to a DecimalFormat object: DecimalFormat dFormatter and set it equal to: new DecimalFormat(); In the parentheses, place "0.00" or "#.##" to round to 2 decimals. Add more zeros of ##'s after the period to change the number of decimals.
DecimalFormat formats variables as strings, so if you want to use a number for calculation you have to parse it back to double or float.
Most practical applications only require a few decimals. Here are 3 ways to round number in Java:
Java's Math class is inherently included in every program you create so there is not need for an import statement. The round() method takes a number as an argument and rounds that number to the nearest integer. On it's own this isn't spectacularly helpful but you can combine it with an easy little trick.
NOTE: The printf method doesn't change the actual contents of the variable. After using printf, the variable still has all the decimals.
3) Using DecimalFormat
Then create a reference variable to a DecimalFormat object: DecimalFormat dFormatter and set it equal to: new DecimalFormat(); In the parentheses, place "0.00" or "#.##" to round to 2 decimals. Add more zeros of ##'s after the period to change the number of decimals.
DecimalFormat formats variables as strings, so if you want to use a number for calculation you have to parse it back to double or float.
Комментарии