filmov
tv
LRU cache | EP 22
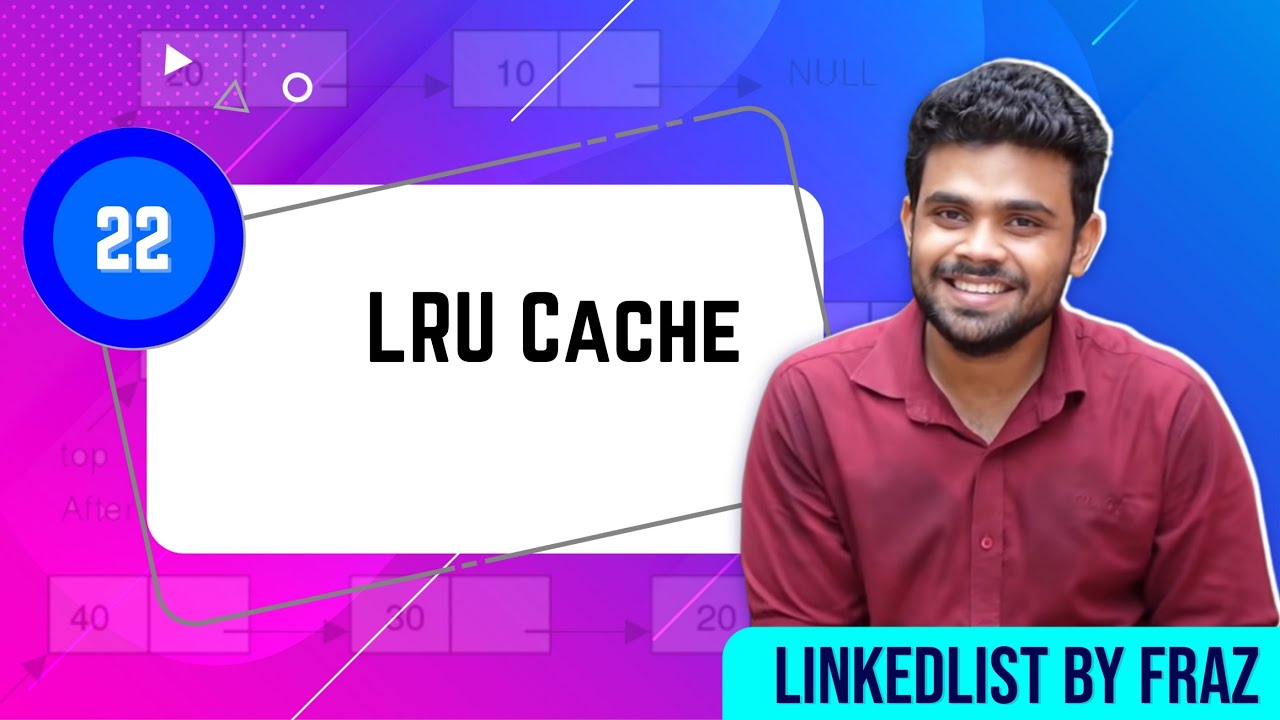
Показать описание
Hi, I am Fraz.
I am Software Engineer working at Curefit and I love teaching.
This is my Channel where I upload content related to Interviews and my personal experiences.
My contact details
LRU cache | EP 22
LRU Cache - Twitch Interview Question - Leetcode 146
ch22 ep#10 Local Cache
What is LRU Cache ? #cache #programming #coding #java #python #datastructures #code #javascript
LRU Cache Replacement Policy - Solved PYQs
LRU Cache Algo and Code Explained
Master LRU Cache with C++ | Efficient Coding with STL Library - 146. LRU Cache
Implement LRU cache with example
What is LRU cache in 10 minutes
LRU Cache - Animation - Solutions - inDepth
LFU Cache - Leetcode 460 - Python
Leetcode 146. LRU Cache - OrderedDict, and review Cache Eviction Policies
What is LRU cache?
lRU cache in Python | How to implement LRU cache that can speed up Function or API Execution
LRU Cache | Brute Force | Optimal | Detailed | Leetcode 146
LRU Cache (Leetcode 146)
Leaking Information Through Cache LRU States in Commercial Processors and Secure Caches (0421)
LRU cache Design
LRU cache | Live Coding with Explanation | Leetcode #146
146. LRU Cache | Leetcode | Python
LRU cache - Amazon interview question |leetcode #146 - python solution
146. LRU Cache | LeetCode
Lecture 22 : An Example of Mapping Techniques and LRU Block Replacement Policy
LRU Cache Implementation (Doubly LinkedList, Hashing)
Комментарии