filmov
tv
Definitive Guide to React Component Design and the key to avoiding tech debt
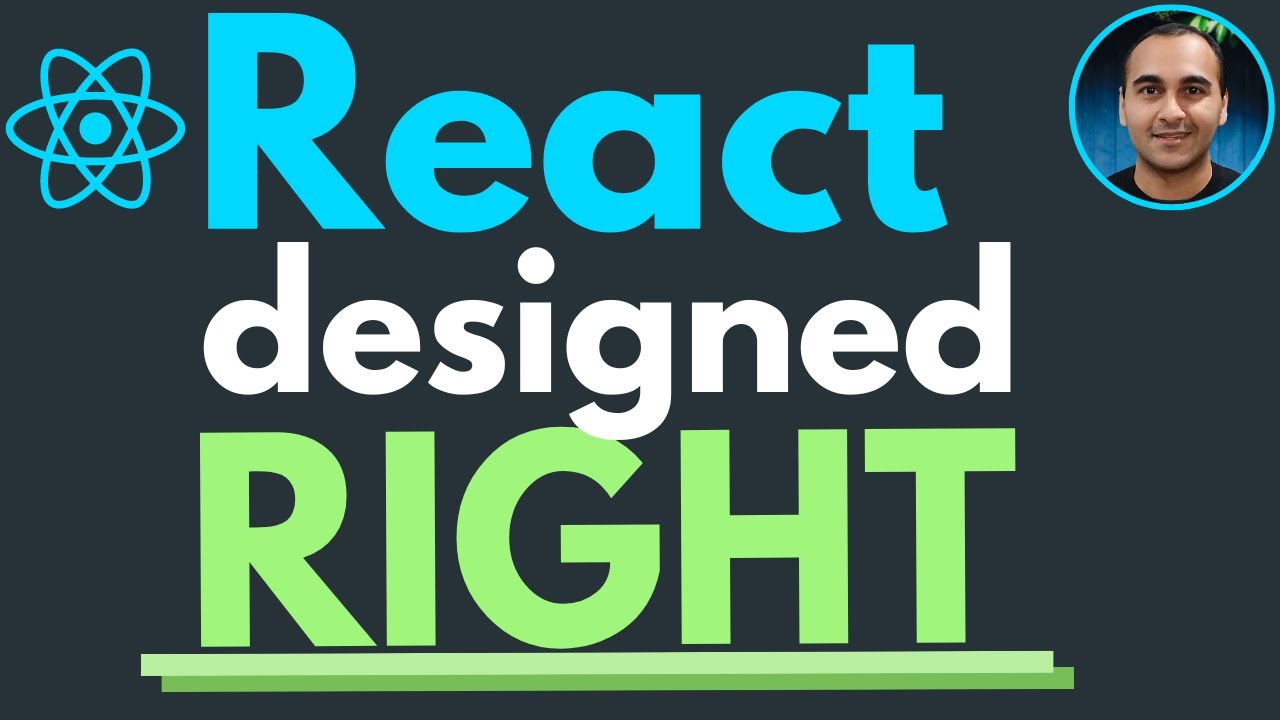
Показать описание
Instead of building up tons of tech debt with React, I'll teach you how to build better, more-flexible React components using "children-first" design principles! It's a combination of compound components (a lost art) and context.
Through years and years of coding day and night, I bring to you, a Definitive Guide to React Component Design; a set of skills and principles you could use for coding even outside of React.
Children-first components:
This talk is about avoiding tech debt to make you a better developer.
Other similar terms are "React Clean Architecture", "React Design Patterns", "React Best Practices", and "Junior vs Senior code".
Through years and years of coding day and night, I bring to you, a Definitive Guide to React Component Design; a set of skills and principles you could use for coding even outside of React.
Children-first components:
This talk is about avoiding tech debt to make you a better developer.
Other similar terms are "React Clean Architecture", "React Design Patterns", "React Best Practices", and "Junior vs Senior code".
Definitive Guide to React Component Design and the key to avoiding tech debt
Creating High-Quality React Components: Best Practices for Reusability
React Tutorial for Beginners
Folder structure in React - Complete Guide
React JS Explained In 10 Minutes
React in 100 Seconds
10 React Antipatterns to Avoid - Code This, Not That!
How To Master React In 2024 (Complete Roadmap)
1 - Setting Up Expo for TikTok Clone with React Native | Step-by-Step Guide
React Full Course for free ⚛️ (2024)
React Forms: the SIMPLEST way
Why you should look into these React component styling options!
Figma to React Component in Five Minutes
What is React Component? A Beginner's Guide
10 React Hooks Explained // Plus Build your own from Scratch
React Styled Components - The Complete Guide
React Components Explained - React MicroBytes (2020)
Typescript for React Components From Beginners to Masters
React JS Full Course 2023 | Build an App and Master React in 1 Hour
Learn React Hooks: useContext - Simply Explained!
Mastering React Context 2023: The Complete Guide!
React Component Lifecycle - Hooks / Methods Explained
Un-Suck Your React Components - Composable & Compound Components
Best way to learn React!
Комментарии