filmov
tv
What Are Identity & Membership Operators in Python | EP-37 Python Membership & Identity Operators
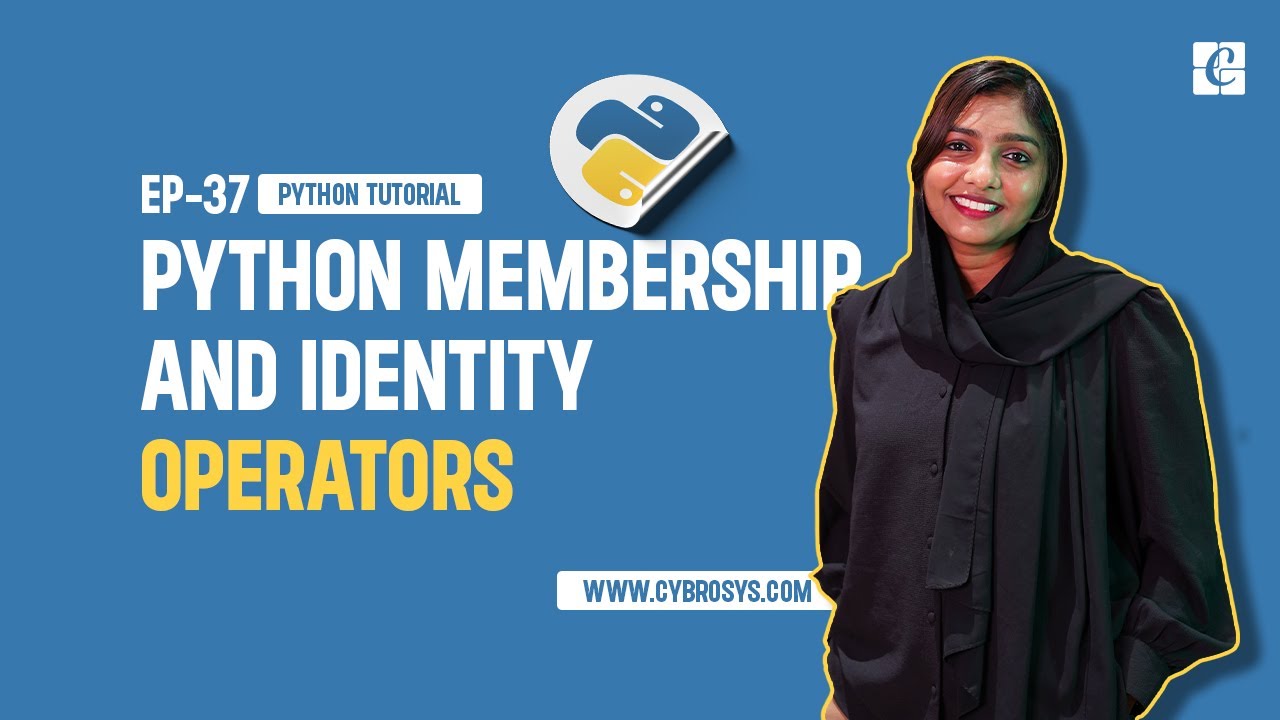
Показать описание
Python Membership and Identity Operators
There is a large set of Python operators that can be used on different datatypes.
Sometimes we need to know if a value belongs to a particular set.
Python Membership Operators
The Python membership operators test for the membership of an object in a sequence, such as strings, lists, or tuples. Python offers two membership operators to check or validate the membership of a value. They are as follows:
Python IN Operator
The in operator is used to check if a character/substring/element exists in a sequence or not.Returns True if the value exists in a sequence, else returns False.
syntax:value in sequence
Company = "cybrosys"
print("e" in Company)
Python NOT IN Operator
Returns False if the value exists in a sequence, else returns True"""
list_1 =[1,2,3,4]
print(2 not in list_1,"not_in")
An alternative to Membership ‘in’ operator is the contains() function.
This function is part of the Operator module in Python. The function take two arguments, the first is the sequence and the second is the value that is to be checked.
import operator
Python Identity Operators
The Python Identity Operators are used to compare the objects if both the objects are actually of the same data type and share the same memory location. There are different
identity operators such as:
1. is Operator
2. is not Operator
Python IS Operator
Returns True if both objects refers to same memory location, else returns False
syntax:obj1 is obj2
num1=5
num2=5
num3=8
print(id(num1))
print(id(num2))
print(id(num3))
print(num1 is num2)
print(num2 is num3)
Python IS NOT Operator
Returns False if both object refers to same memory location, else returns True
syntax: obj1 is not obj2
num1=5
num2=5
num3=8
print(id(num1))
print(id(num2))
print(id(num3))
print(num1 is not num2)
print(num2 is not num3)
Difference between ‘==’ and ‘is’ Operator
While comparing objects in Pyhton, the users often gets confused between the Equality operator and Identity ‘is’ operator. The equality operator is used to compare the value of two variables, whereas the identities operator is used to compare the memory location
of two variables.
num = 2
num1 = 2
using 'is' and '==' operators
print(num is num1)
print(num == num1)
#PythonTutorial #PythonOperators #IdentityOperators #MembershipOperators #LearnPython #PythonProgramming #PythonForBeginners #CodingInPython #PythonTips #PythonTutorials #PythonCoding #ProgrammingTips #Python #LearnPython #Coding #Programming #TechTips
Connect With Us:
—————————————
There is a large set of Python operators that can be used on different datatypes.
Sometimes we need to know if a value belongs to a particular set.
Python Membership Operators
The Python membership operators test for the membership of an object in a sequence, such as strings, lists, or tuples. Python offers two membership operators to check or validate the membership of a value. They are as follows:
Python IN Operator
The in operator is used to check if a character/substring/element exists in a sequence or not.Returns True if the value exists in a sequence, else returns False.
syntax:value in sequence
Company = "cybrosys"
print("e" in Company)
Python NOT IN Operator
Returns False if the value exists in a sequence, else returns True"""
list_1 =[1,2,3,4]
print(2 not in list_1,"not_in")
An alternative to Membership ‘in’ operator is the contains() function.
This function is part of the Operator module in Python. The function take two arguments, the first is the sequence and the second is the value that is to be checked.
import operator
Python Identity Operators
The Python Identity Operators are used to compare the objects if both the objects are actually of the same data type and share the same memory location. There are different
identity operators such as:
1. is Operator
2. is not Operator
Python IS Operator
Returns True if both objects refers to same memory location, else returns False
syntax:obj1 is obj2
num1=5
num2=5
num3=8
print(id(num1))
print(id(num2))
print(id(num3))
print(num1 is num2)
print(num2 is num3)
Python IS NOT Operator
Returns False if both object refers to same memory location, else returns True
syntax: obj1 is not obj2
num1=5
num2=5
num3=8
print(id(num1))
print(id(num2))
print(id(num3))
print(num1 is not num2)
print(num2 is not num3)
Difference between ‘==’ and ‘is’ Operator
While comparing objects in Pyhton, the users often gets confused between the Equality operator and Identity ‘is’ operator. The equality operator is used to compare the value of two variables, whereas the identities operator is used to compare the memory location
of two variables.
num = 2
num1 = 2
using 'is' and '==' operators
print(num is num1)
print(num == num1)
#PythonTutorial #PythonOperators #IdentityOperators #MembershipOperators #LearnPython #PythonProgramming #PythonForBeginners #CodingInPython #PythonTips #PythonTutorials #PythonCoding #ProgrammingTips #Python #LearnPython #Coding #Programming #TechTips
Connect With Us:
—————————————