filmov
tv
Word Search II - Backtracking Trie - Leetcode 212 - Python
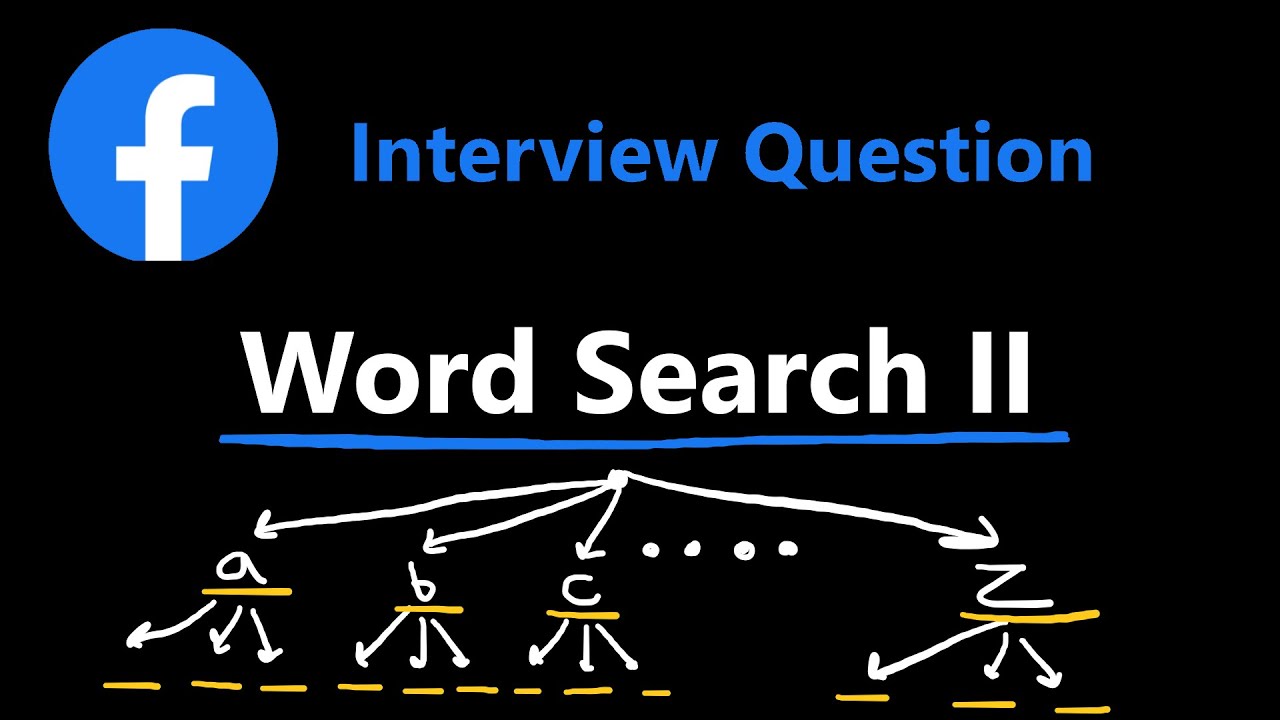
Показать описание
0:00 - Read the problem
5:06 - Drawing Explanation
12:32 - Coding Explanation
leetcode 212
#trie #prefix #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Word Search II - Backtracking Trie - Leetcode 212 - Python
Word Search - Backtracking - Leetcode 79 - Python
[Java] Leetcode 212. Word Search II [Backtracking #12]
Word Search II | DFS + Map | DFS + TRIE | Leetcode #212
Leetcode #212: Word Search II (DFS + Bactracking + Trie) | Code Explained
Word Search II - LeetCode 212 - JavaScript
🚀 Master LeetCode 212: Word Search II - Efficient Trie and Backtracking Solution 🌟 | Hack Code
LeetCode 212. Word Search II Explanation and Solution
Word Search II | Day 30 | Trie Data Structure [ June LeetCoding Challenge ] [ Leetcode #212] [ 2020]
Word Search 2 | Backtracking and Trie Data Structure | Leetcode 212
𝗪𝗼𝗿𝗱 𝗦𝗲𝗮𝗿𝗰𝗵 | 𝗟𝗲𝗲𝘁𝗰𝗼𝗱𝗲 𝟳𝟵 | 𝗦𝗶𝗺𝗽𝗹𝗲 𝗩𝗶𝘀𝘂𝗮𝗹𝗶𝘇𝗮𝘁𝗶𝗼𝗻 | 𝗗𝗙𝗦 | 𝗕𝗮𝗰𝗸𝗧𝗿𝗮𝗰𝗸𝗶𝗻𝗴 | 𝗣𝗵𝗮𝗻𝗶 𝗧𝗵𝗮𝘁𝗶𝗰𝗵𝗮𝗿𝗹𝗮...
Word Search II | Leetcode 212 | Leetcode Hard | Live coding session 🔥🔥🔥
Word Search 2 (LeetCode 212 - Hard)
Word Search II
Word Search II | Leetcode 212 | Trie | Backtracking | DSA | Explanation from Basics
Word Search II | LeetCode 212 | Java | DFS + TRIE | @LearnOverflow
Leetcode - Word Search II (Python)
79. Word Search | Recursion & Backtracking | 489. Robot Room Cleaner | 2 Approaches
Word Search ii | LeetCode 212 | C++, Java, Python
Word Search II - LeetCode 212 - Python
212. Word Search II - Day 5/30 Leetcode November Challenge
Readable LeetCode Solution Word Search II Depth First Search
Word Search II | word search ii leetcode | word search 2 leetcode | leetcode 212
Word Search II | LeetCode | Solution Explained in Detail
Комментарии