filmov
tv
why does inheritance suck?
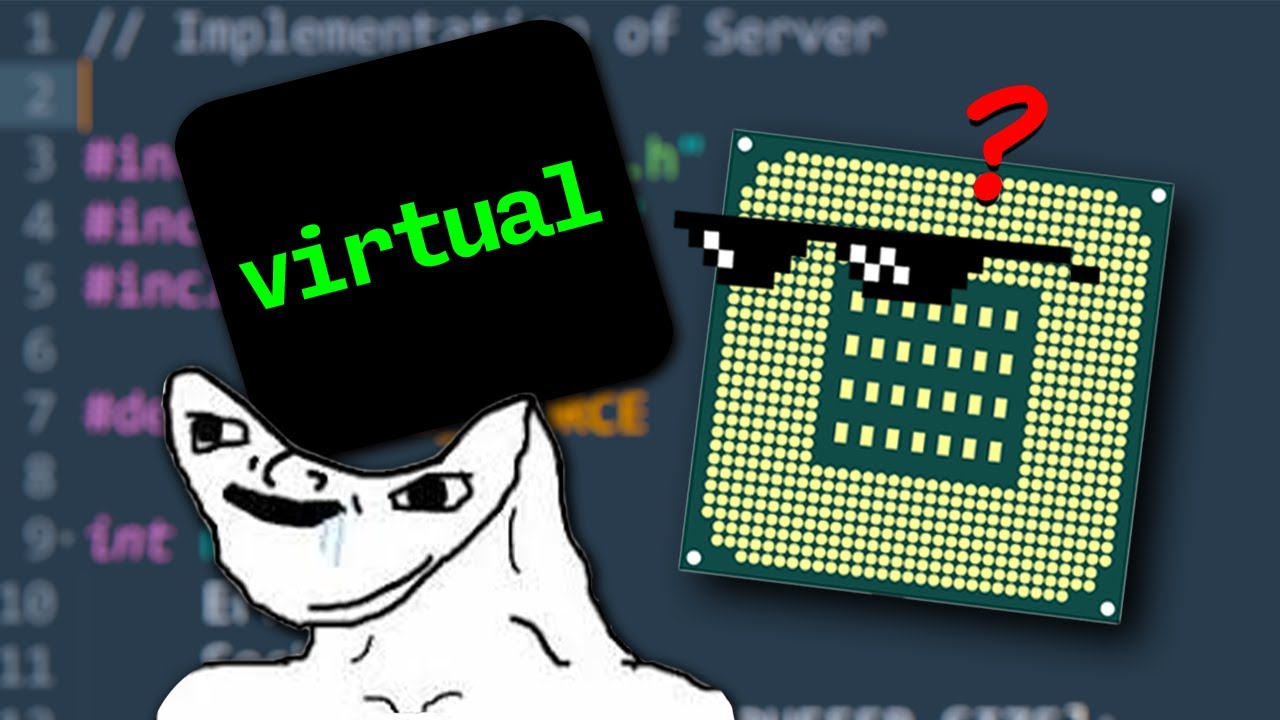
Показать описание
You've probably heard this a few times when talking to your fellow programmer friends. "Gee Billy C++ polymorphism sure is slow, I hope Sally doesn't know that I use it!" But why is it so bad? In this video, we'll do a deep dive on what C++ Polymorphism is, what "virtual" does under the hood, and ultimately why it is SUCH a performance hit compared to languages like C and Rust.
🔥🔥🔥 SOCIALS 🔥🔥🔥
🔥🔥🔥 SOCIALS 🔥🔥🔥
why does inheritance suck?
The Flaws of Inheritance
Prime Reacts: The Flaws of Inheritance
Why OOP inheritance sucks
Epigenetics: Why Inheritance Is Weirder Than We Thought
Is Epigenetic Inheritance Real?
Composition over inheritance. Why Inheritance sucks.
Object-Oriented Programming is Bad
Reacting to Controversial Opinions of Software Engineers
Ep17 - Why Inheritance is bad? OOP Composition vs Inheritance
Avoid Deep Class Inheritance Trees
Farmers should 'suck it up' and pay inheritance tax, argues Matthew Wright
#shorts composition vs inheritance
Young Generations Are Now Poorer Than Their Parents And It's Changing Our Economies
PROOF: Trump's kids are suck ups to get his inheritance
If Eragon Was SO Bad, Why So Successful? | The Inheritance Cycle By Christopher Paolini
Abstraction Can Make Your Code Worse
📏 What Causes Tallness and Shortness
Object-Oriented Programming is Good*
I just inherited 50 MILLION dollars. What should I do with it?
Are many scapegoats left out of inheritance?
Handmade Hero | Getting rid of the OOP mindset
Inheritance #standup #standupcomedy #shorts #youtubeshorts #comedy #jokes #funny #lol
I Inherited $2,500,000 in Stocks!
Комментарии