filmov
tv
Fixing the TypeError in Python: A Guide to Class Method Usage
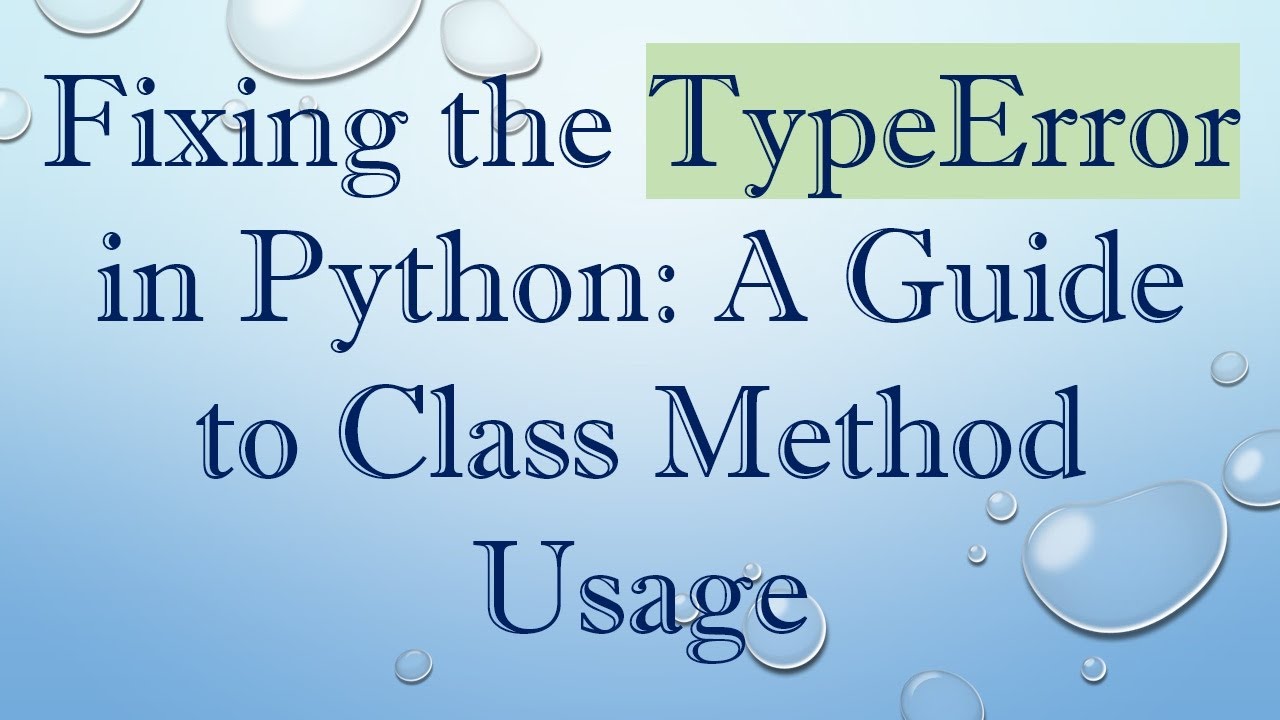
Показать описание
Learn how to resolve the `TypeError: Menu() missing 1 required positional argument: 'self'` in Python by correctly using class instances and methods.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: simple python menu - missing Self
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing the TypeError in Python: A Guide to Class Method Usage
When working with classes in Python, one common issue developers encounter is the TypeError: Menu() missing 1 required positional argument: 'self'. This error usually occurs when attempting to call a method from a class without properly instantiating that class. In this guide, we'll break down this problem and provide a straightforward solution.
The Problem: Understanding the TypeError
The error message can be confusing at first. Here’s what it means:
TypeError: This is a built-in error in Python that signals an operation or function is applied to an object of inappropriate type.
missing 1 required positional argument: 'self': This part indicates that when calling the method Menu(), Python expects the instance of the class (referred to as self) as the first argument.
Why This Happens
In Python, when you define a method within a class, it requires an instance of the class to be called. The instance is represented by the self parameter. If you try to call a method directly without referencing an instance of the class, you'll face the TypeError.
The Solution: Creating an Instance of the Class
To resolve this issue, you need to create an instance of your SupermarketDAO class and then call its Menu method. Here’s how to do it step-by-step:
Step 1: Create an Instance of the Class
In your code, instead of calling self.Menu() directly inside the __init__ method, follow this structure:
[[See Video to Reveal this Text or Code Snippet]]
Here, you create an instance called supermarket, which correctly initializes the __init__ method.
Step 2: Calling the Menu Method
To call the Menu method, you simply use the instance you created:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Here’s how the full implementation should look with the improvements made:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In conclusion, the key to resolving the TypeError: Menu() missing 1 required positional argument: 'self' lies in ensuring you create an instance of your class before calling its methods. By following the steps outlined in this guide, you can effectively manage and utilize class methods while avoiding common errors. Keep coding and happy developing!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: simple python menu - missing Self
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing the TypeError in Python: A Guide to Class Method Usage
When working with classes in Python, one common issue developers encounter is the TypeError: Menu() missing 1 required positional argument: 'self'. This error usually occurs when attempting to call a method from a class without properly instantiating that class. In this guide, we'll break down this problem and provide a straightforward solution.
The Problem: Understanding the TypeError
The error message can be confusing at first. Here’s what it means:
TypeError: This is a built-in error in Python that signals an operation or function is applied to an object of inappropriate type.
missing 1 required positional argument: 'self': This part indicates that when calling the method Menu(), Python expects the instance of the class (referred to as self) as the first argument.
Why This Happens
In Python, when you define a method within a class, it requires an instance of the class to be called. The instance is represented by the self parameter. If you try to call a method directly without referencing an instance of the class, you'll face the TypeError.
The Solution: Creating an Instance of the Class
To resolve this issue, you need to create an instance of your SupermarketDAO class and then call its Menu method. Here’s how to do it step-by-step:
Step 1: Create an Instance of the Class
In your code, instead of calling self.Menu() directly inside the __init__ method, follow this structure:
[[See Video to Reveal this Text or Code Snippet]]
Here, you create an instance called supermarket, which correctly initializes the __init__ method.
Step 2: Calling the Menu Method
To call the Menu method, you simply use the instance you created:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Here’s how the full implementation should look with the improvements made:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In conclusion, the key to resolving the TypeError: Menu() missing 1 required positional argument: 'self' lies in ensuring you create an instance of your class before calling its methods. By following the steps outlined in this guide, you can effectively manage and utilize class methods while avoiding common errors. Keep coding and happy developing!