filmov
tv
Exploring Alternatives to InnerHTML in JavaScript
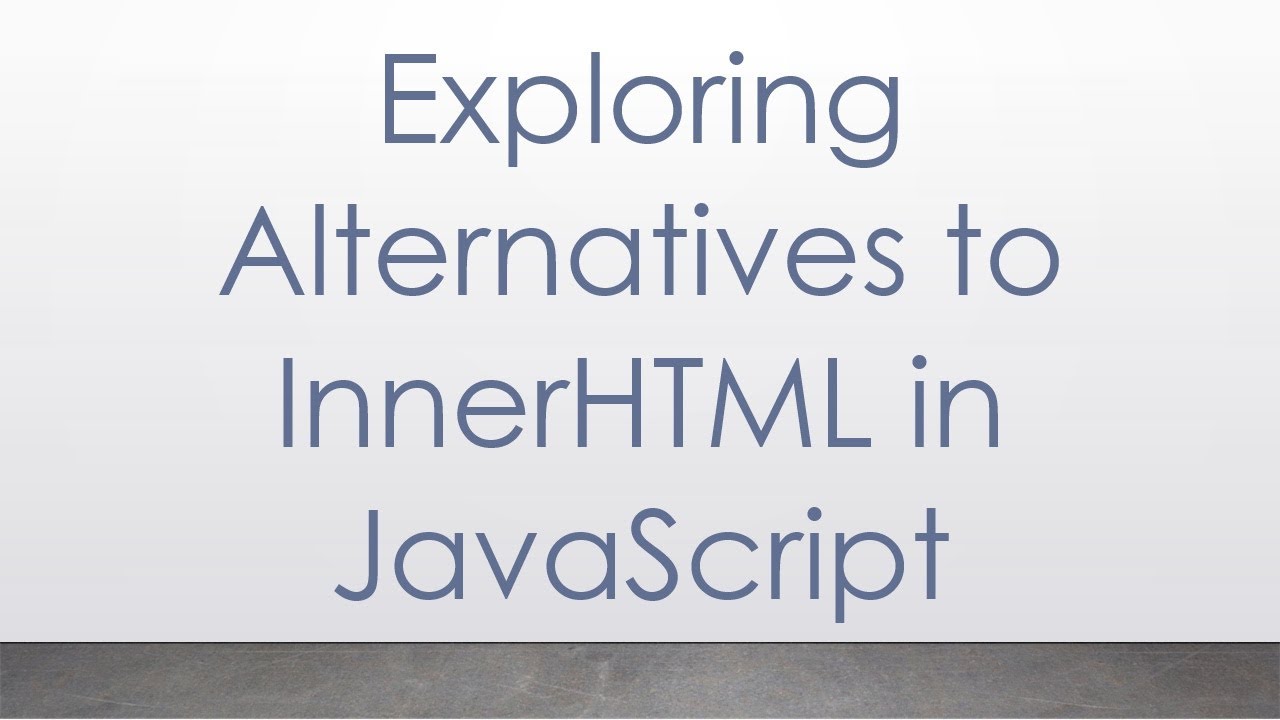
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Discover efficient and safe alternatives to using `innerHTML` in JavaScript for updating the DOM. Learn best practices for working with elements and improving web app performance and security.
---
When it comes to manipulating the DOM in JavaScript, one common method developers often use is innerHTML. While it's a straightforward and powerful way to update page content, it has its drawbacks, such as security vulnerabilities and potential performance issues. Let's explore some alternatives to innerHTML that can help you build more secure and efficient web applications.
The Drawbacks of Using innerHTML
Before diving into alternatives, it's important to understand why innerHTML may not always be the best choice:
Security Risks:
Cross-Site Scripting (XSS): Directly setting innerHTML can introduce XSS vulnerabilities if the content is not properly sanitized.
Performance Issues:
Reflows and Repaints: Setting innerHTML causes the browser to re-parse and re-render the entire content of an element, which can be inefficient especially for large documents.
Alternative Methods
textContent
If you're working with text and don't need to include HTML tags, textContent is a safer and more efficient alternative:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Security: It automatically escapes any HTML tags, preventing malicious script execution.
Performance: Faster than innerHTML as it does not parse and render HTML.
createElement and appendChild
For more complex HTML content, consider creating and appending new elements manually:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Granular Control: Allows more precise manipulation of the DOM.
Performance: Minimizes reflows by updating smaller parts of the DOM incrementally.
Template Literals with DOM Manipulation
You can use template literals and a combination of createElement, and appendChild to structure and insert HTML content:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Separation of Concerns: Keeps HTML structure clear and separate from JavaScript logic.
Security: You can sanitize parts if needed before inserting into the DOM.
If you prefer to insert HTML directly but need better performance and security control, insertAdjacentHTML can be a good middle ground:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Performance: More efficient than innerHTML as it doesn’t re-render the entire content.
Flexibility: Allows insertion at various positions relative to the target element.
Conclusion
While innerHTML is a convenient way to manipulate the DOM, considering its drawbacks, especially related to security and performance, makes it worthwhile to explore alternatives. Methods like textContent, createElement, and appendChild, as well as insertAdjacentHTML, offer safer and more efficient ways to update web content. By incorporating these techniques, you can build more robust, secure, and performant applications.
Adopting best practices in DOM manipulation not only enhances user experience but also fortifies your applications against common vulnerabilities.
---
Summary: Discover efficient and safe alternatives to using `innerHTML` in JavaScript for updating the DOM. Learn best practices for working with elements and improving web app performance and security.
---
When it comes to manipulating the DOM in JavaScript, one common method developers often use is innerHTML. While it's a straightforward and powerful way to update page content, it has its drawbacks, such as security vulnerabilities and potential performance issues. Let's explore some alternatives to innerHTML that can help you build more secure and efficient web applications.
The Drawbacks of Using innerHTML
Before diving into alternatives, it's important to understand why innerHTML may not always be the best choice:
Security Risks:
Cross-Site Scripting (XSS): Directly setting innerHTML can introduce XSS vulnerabilities if the content is not properly sanitized.
Performance Issues:
Reflows and Repaints: Setting innerHTML causes the browser to re-parse and re-render the entire content of an element, which can be inefficient especially for large documents.
Alternative Methods
textContent
If you're working with text and don't need to include HTML tags, textContent is a safer and more efficient alternative:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Security: It automatically escapes any HTML tags, preventing malicious script execution.
Performance: Faster than innerHTML as it does not parse and render HTML.
createElement and appendChild
For more complex HTML content, consider creating and appending new elements manually:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Granular Control: Allows more precise manipulation of the DOM.
Performance: Minimizes reflows by updating smaller parts of the DOM incrementally.
Template Literals with DOM Manipulation
You can use template literals and a combination of createElement, and appendChild to structure and insert HTML content:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Separation of Concerns: Keeps HTML structure clear and separate from JavaScript logic.
Security: You can sanitize parts if needed before inserting into the DOM.
If you prefer to insert HTML directly but need better performance and security control, insertAdjacentHTML can be a good middle ground:
[[See Video to Reveal this Text or Code Snippet]]
Benefits:
Performance: More efficient than innerHTML as it doesn’t re-render the entire content.
Flexibility: Allows insertion at various positions relative to the target element.
Conclusion
While innerHTML is a convenient way to manipulate the DOM, considering its drawbacks, especially related to security and performance, makes it worthwhile to explore alternatives. Methods like textContent, createElement, and appendChild, as well as insertAdjacentHTML, offer safer and more efficient ways to update web content. By incorporating these techniques, you can build more robust, secure, and performant applications.
Adopting best practices in DOM manipulation not only enhances user experience but also fortifies your applications against common vulnerabilities.