filmov
tv
Create a Simple Chatbot Using ChatGPT API in React Native
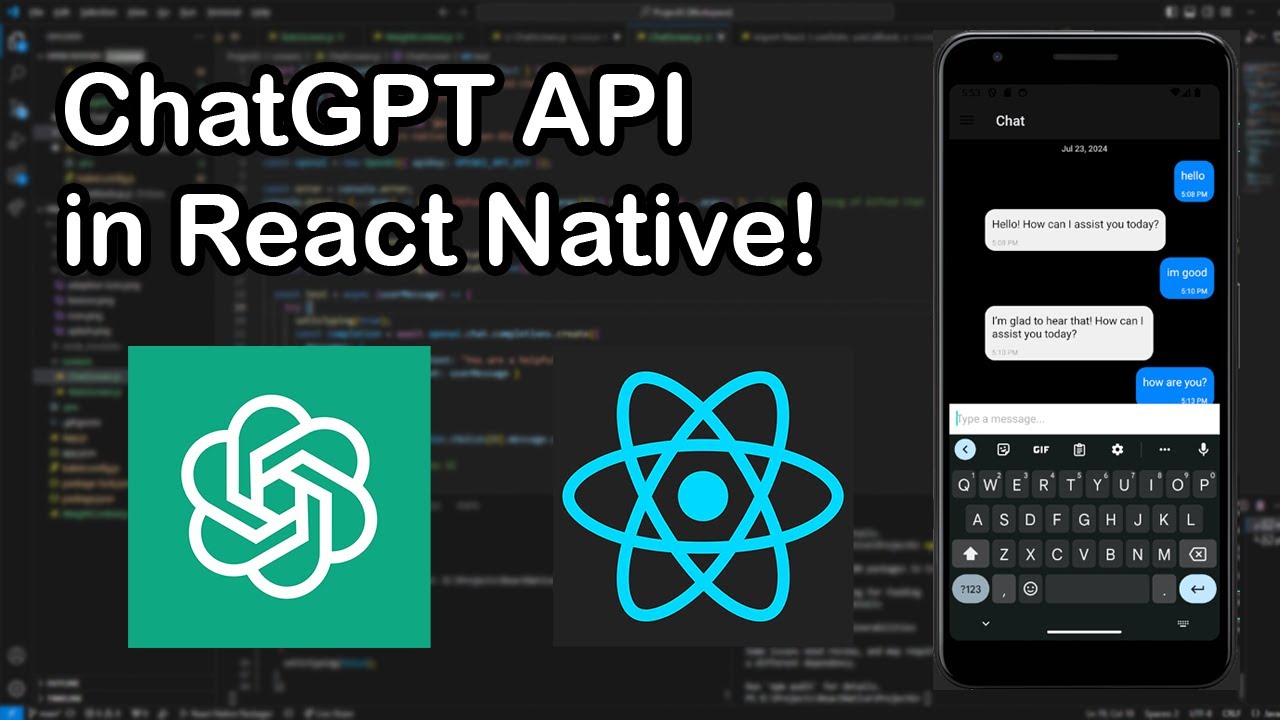
Показать описание
0:00 - Tutorial
6:00 - Bonus: Using Markdown
6:58 - Bonus: Typing indicator
----------------------------------------------------------------
npm install react-native-gifted-chat --save
npm install react-native-uuid
npm install openai --save
npm install react-native-dotenv --save
Optional:
npm install react-native-markdown-display
add:
plugins: ['module:react-native-dotenv'],
like so:
return {
presets: ['babel-preset-expo'],
plugins: ['module:react-native-dotenv'],
};
};
To get your API Key:
The code will be in the comments.
6:00 - Bonus: Using Markdown
6:58 - Bonus: Typing indicator
----------------------------------------------------------------
npm install react-native-gifted-chat --save
npm install react-native-uuid
npm install openai --save
npm install react-native-dotenv --save
Optional:
npm install react-native-markdown-display
add:
plugins: ['module:react-native-dotenv'],
like so:
return {
presets: ['babel-preset-expo'],
plugins: ['module:react-native-dotenv'],
};
};
To get your API Key:
The code will be in the comments.
Create a Python GPT Chatbot - In Under 4 Minutes
Build a Chatbot with AI in 5 minutes
Create a Simple Chatbot using Hugging Face
How to create an accurate Chat Bot Response System in Python Tutorial (2021)
How To Build A Chat Bot That Learns From The User In Python Tutorial
Build a Large Language Model AI Chatbot using Retrieval Augmented Generation
Build an AI Chatbot on your Custom Data 🔥
Create a basic chatbot with Dialogflow
Build an AI Chatbot using Dify AI and Streamlit
How to Build AI ChatBot with Custom Knowledge Base in 10 mins
Build & Integrate your own custom chatbot to a website (Python & JavaScript)
Chatbot using Python, NLP, and Data Science | Build Your Own Chatbot | Intellipaat
Python Chatbot Tutorial | How to Create Chatbot Using Python | Python For Beginners | Simplilearn
Build AI Chat Bot using ChatGPT API 👉 gpt-3.5-turbo 👈 #chatgpt #openai
ChatGPT / BARD API - Build a FREE Chatbot in Python w/ Google Colab
Python Chat Bot Tutorial - Chatbot with Deep Learning (Part 1)
Introduction to Chatbots | NLP Tutorial | S3 E1
Make a Simple Chatbot Using Python Tkinter GUI | Chatbot Tutorial | Syntax Error Pratiksha Jain
ChatGPT in Python for Beginners - Build A Chatbot
How to create an accurate Chat Bot Response System in Python Tutorial (2022)
Build your own chatbot using Python | Python Tutorial for Beginners in 2022 | Great Learning
Build your own RAG (retrieval augmented generation) AI Chatbot using Python | Simple walkthrough
Intelligent AI Chatbot in Python
Learn How to Build a Chatbot with Flask - Step-by-Step Tutorial with HTML, CSS, and JavaScript
Комментарии