filmov
tv
Consume Web APIs Using HttpClient with Post Method | Part 11
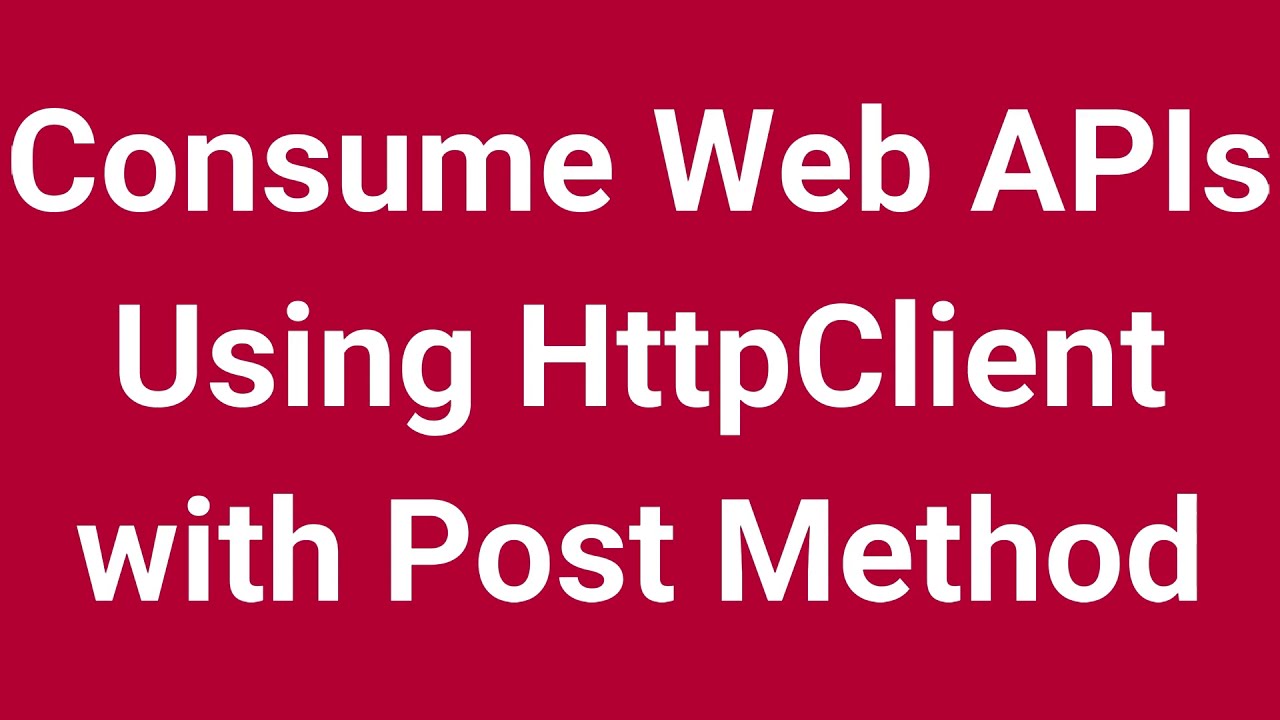
Показать описание
Here we will learn how to Consume Web APIs Using HttpClient with Post Method
Consider we have client web api to post the record.
Method Name: Post
Parameter : object as json format
{
"empid": 120,
"Name": "Hoyam",
"salary": 40000
}
Steps 1: Make a aspx page to consume
Steps: 2 Make a class for JSON
For this We need to make a class like below, then we will assign the value for the new employee to be inserted in the database
public class Employee
{
public int empid { get; set; }
public string Name { get; set; }
public decimal salary { get; set; }
}
protected void Button1_Click(object sender, EventArgs e)
{
try
{
Employee emp = new Employee();
emp.Name = Convert.ToString(txtName.Text);
Consume_WebAPI(emp).Wait();
}
catch (Exception ex)
{
}
}
static async Task Consume_WebAPI(Employee emp)
{
var client = new HttpClient();
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var stringContent = new StringContent(JsonConvert.SerializeObject(emp), Encoding.UTF8, "application/json");
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Ssl3;
var response = await client.PostAsync("api/employee/Add", stringContent).ConfigureAwait(false);
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Ssl3;
response.EnsureSuccessStatusCode();
if (response.IsSuccessStatusCode)
{
dynamic result = await response.Content.ReadAsStringAsync();
}
}
public class Employee
{
public int empid { get; set; }
public string Name { get; set; }
public decimal salary { get; set; }
}
Note:
Since When we are using .Result or .Wait or await this will end up causing a deadlock in our code of aspx page it will give the results in console application due to no context in console application So.
we can use ConfigureAwait(false) in async methods for preventing deadlock
like this:
var response = await client.PostAsync("api/employee/Add", stringContent).ConfigureAwait(false);
We can use ConfigureAwait(false) wherever possible for Don't Block Async Code .
Consider we have client web api to post the record.
Method Name: Post
Parameter : object as json format
{
"empid": 120,
"Name": "Hoyam",
"salary": 40000
}
Steps 1: Make a aspx page to consume
Steps: 2 Make a class for JSON
For this We need to make a class like below, then we will assign the value for the new employee to be inserted in the database
public class Employee
{
public int empid { get; set; }
public string Name { get; set; }
public decimal salary { get; set; }
}
protected void Button1_Click(object sender, EventArgs e)
{
try
{
Employee emp = new Employee();
emp.Name = Convert.ToString(txtName.Text);
Consume_WebAPI(emp).Wait();
}
catch (Exception ex)
{
}
}
static async Task Consume_WebAPI(Employee emp)
{
var client = new HttpClient();
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var stringContent = new StringContent(JsonConvert.SerializeObject(emp), Encoding.UTF8, "application/json");
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Ssl3;
var response = await client.PostAsync("api/employee/Add", stringContent).ConfigureAwait(false);
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Ssl3;
response.EnsureSuccessStatusCode();
if (response.IsSuccessStatusCode)
{
dynamic result = await response.Content.ReadAsStringAsync();
}
}
public class Employee
{
public int empid { get; set; }
public string Name { get; set; }
public decimal salary { get; set; }
}
Note:
Since When we are using .Result or .Wait or await this will end up causing a deadlock in our code of aspx page it will give the results in console application due to no context in console application So.
we can use ConfigureAwait(false) in async methods for preventing deadlock
like this:
var response = await client.PostAsync("api/employee/Add", stringContent).ConfigureAwait(false);
We can use ConfigureAwait(false) wherever possible for Don't Block Async Code .
Комментарии