filmov
tv
Don't throw exceptions in C#. Do this instead
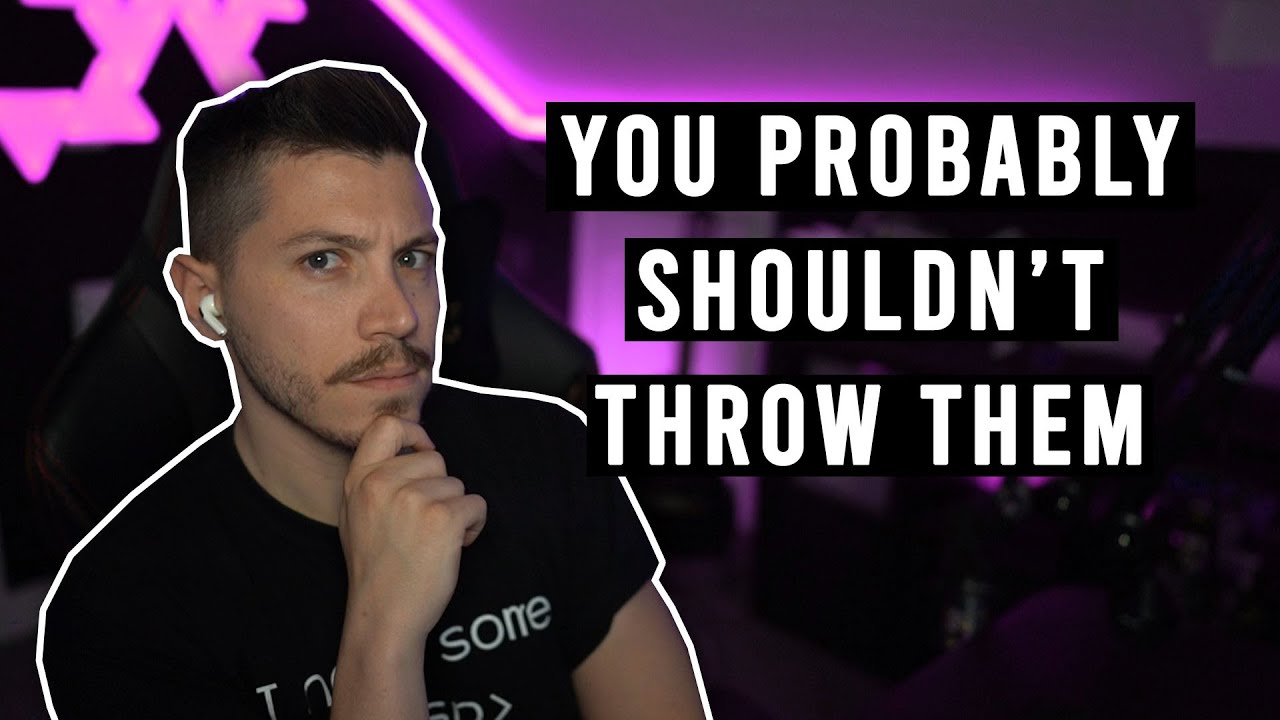
Показать описание
Hello everybody I'm Nick and in this video I will show you how you can potentially replace some of your exception throwing in your C# application with a differnent way of handling bad state. It is a concept that is native in other languages like Rust and F#, and it will eventually make its way into C# too but for now, here is the workaround.
Don't forget to comment, like and subscribe :)
Social Media:
#csharp #dotnet
Don't throw exceptions in C#. Do this instead
Never Re-Throw Exceptions in C# Do This Instead - DevChatter #csharp #programming #tutorial
You're Throwing Exceptions Wrong! Don't Throw Away The Trace!
What If We Didn't Throw Exceptions In #csharp #dotnet
'Don't Throw Exceptions, Use A Result Pattern' is BAD ADVICE!
The WRONG Way To Throw an IOException
When To Throw Exceptions?
Handling Exceptions in C# - When to catch them, where to catch them, and how to catch them
Master Kotlin Basics: Exceptions 🚀
Exception Handling in C#: Try, Catch, Finally, and Throw Explained
Rachel's C++ Course - Exceptions, Try / Catch / Throw
Nomadic life: Azita's mother-in-law plans to kick her out of the house
C++ : function doesn't throw bad_alloc exception
When To Validate and When To Throw Exceptions?
Throw as a savior for Developers | Throw vs Throw Ex | Awesome Codes
Exception Handling in C++ Programming
C# Tutorial for Beginners #30 - throw Keyword
C# : Error Handling Should I throw exception? Or handle at the source?
Exception Handling in C# .Net made easy! | Try Catch Finally | Throw | Throw ex | Codelligent
C# - Part 42 - Exception Handling | Try | Catch | Finally | Throw - Tutorial For Beginners
Learn Finance C++, Lesson 31, Interlude for Exceptions, throw, try, and catch
Exception Handling using Throw-ReThrow Statement In C#
WAP c++ of all possible exception use a try block to throw it and a catch block| #exception #viral
【ENG SUB】💕She married a stranger in order to raise money, unexpectedly he's a CEO,true love com...
Комментарии