filmov
tv
How to Use std::array for Returning Arrays in C++ Functions
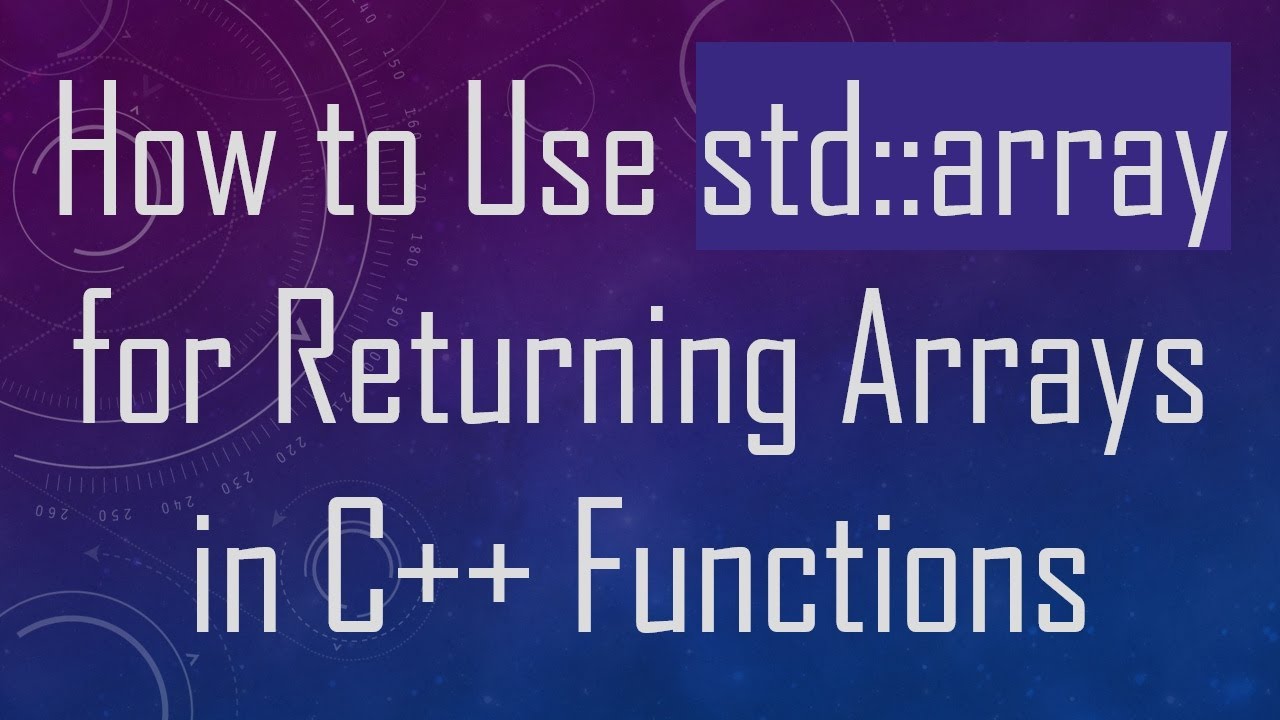
Показать описание
Learn how to return an array of custom structure objects in C++ using `std::array`, keeping your code concise and effective.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Return an array which is iniated in a one liner
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Array Returns in C++ with std::array
In the world of C++, working with arrays can often come with its own set of challenges. One common problem encountered by developers is how to return an array from a function. If you're looking to simplify this process while keeping your code clean and efficient, you’re in the right place! This guide will guide you through returning an array of custom structure objects using the std::array class, eliminating unnecessary complexities.
The Challenge
Let's start by addressing the original problem. The objective is to return an array of a custom structure called timeInDay, which represents times in a day for various weekdays. The challenge was that directly returning a C-style array from a function is not allowed in C++. The user attempted to do so and encountered the following error:
[[See Video to Reveal this Text or Code Snippet]]
Clearly, a different approach is needed to achieve the desired functionality efficiently.
The Solution
Instead of returning a traditional C-style array, there's a simple and modern approach using C++’s Standard Library. Here’s how you can accomplish this with std::array, which ensures type safety and better memory management.
Step 1: Define Your Structure
First, you need to define your timeInDay structure that includes the hours and minutes for the time representation. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the Function Using std::array
Next, instead of trying to return a C-style array, you can utilize std::array. This not only simplifies the syntax but also ensures that you can return your array safely.
Here's an illustration:
[[See Video to Reveal this Text or Code Snippet]]
Why std::array?
Type Safety: Unlike C-style arrays, std::array is type-safe and its size is part of the type.
No Manual Memory Management: With std::array, there is no need to worry about memory allocation and deallocation.
Simplified Syntax: You can initialize it inline, making your code cleaner and more maintainable.
Conclusion
By using std::array, you successfully simplify the return of arrays from functions in C++. This modern approach minimizes errors and makes your code more robust. If your requirements evolve to needing a dynamic size array, consider using std::vector for even greater flexibility.
So, next time you find yourself facing the tricky issue of returning an array in C++, remember this streamlined approach using std::array to keep your coding elegant and error-free!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Return an array which is iniated in a one liner
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Array Returns in C++ with std::array
In the world of C++, working with arrays can often come with its own set of challenges. One common problem encountered by developers is how to return an array from a function. If you're looking to simplify this process while keeping your code clean and efficient, you’re in the right place! This guide will guide you through returning an array of custom structure objects using the std::array class, eliminating unnecessary complexities.
The Challenge
Let's start by addressing the original problem. The objective is to return an array of a custom structure called timeInDay, which represents times in a day for various weekdays. The challenge was that directly returning a C-style array from a function is not allowed in C++. The user attempted to do so and encountered the following error:
[[See Video to Reveal this Text or Code Snippet]]
Clearly, a different approach is needed to achieve the desired functionality efficiently.
The Solution
Instead of returning a traditional C-style array, there's a simple and modern approach using C++’s Standard Library. Here’s how you can accomplish this with std::array, which ensures type safety and better memory management.
Step 1: Define Your Structure
First, you need to define your timeInDay structure that includes the hours and minutes for the time representation. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the Function Using std::array
Next, instead of trying to return a C-style array, you can utilize std::array. This not only simplifies the syntax but also ensures that you can return your array safely.
Here's an illustration:
[[See Video to Reveal this Text or Code Snippet]]
Why std::array?
Type Safety: Unlike C-style arrays, std::array is type-safe and its size is part of the type.
No Manual Memory Management: With std::array, there is no need to worry about memory allocation and deallocation.
Simplified Syntax: You can initialize it inline, making your code cleaner and more maintainable.
Conclusion
By using std::array, you successfully simplify the return of arrays from functions in C++. This modern approach minimizes errors and makes your code more robust. If your requirements evolve to needing a dynamic size array, consider using std::vector for even greater flexibility.
So, next time you find yourself facing the tricky issue of returning an array in C++, remember this streamlined approach using std::array to keep your coding elegant and error-free!