filmov
tv
132 Python Lab 1
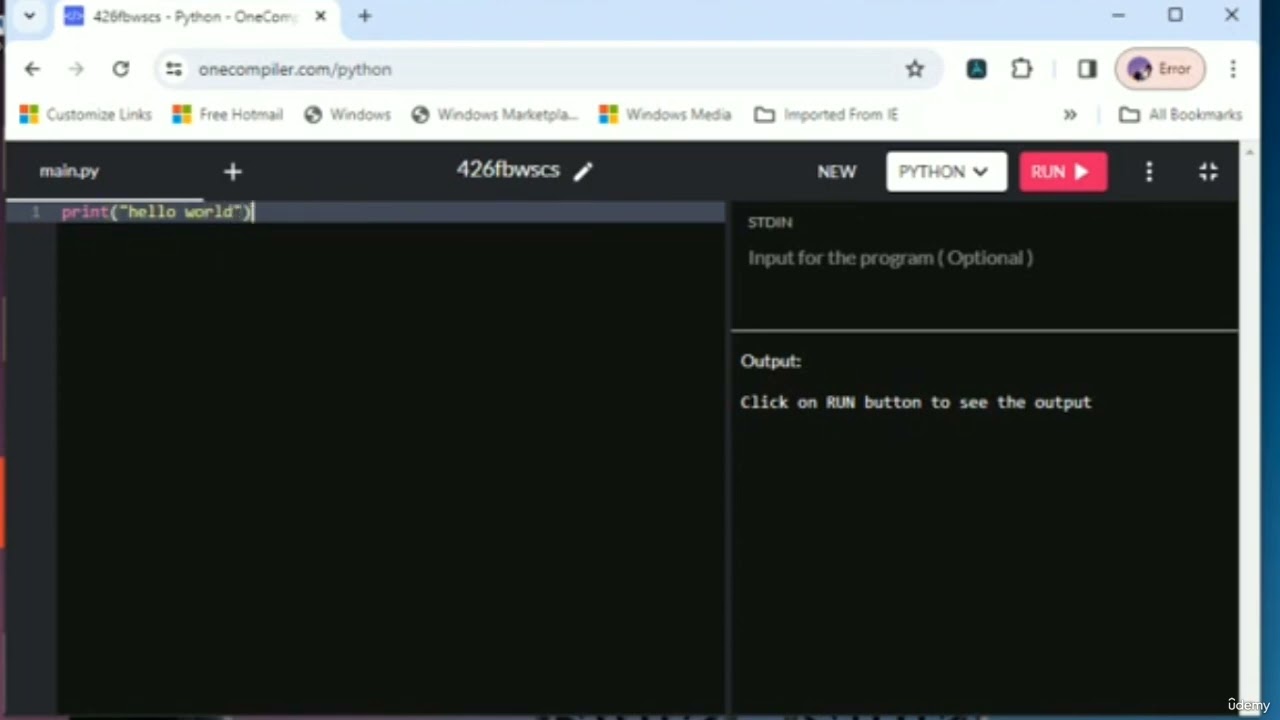
Показать описание
**Python Lab 1** typically refers to an introductory exercise or practical session designed to help students or learners get familiar with the basics of Python programming. This lab is usually part of the curriculum for beginners who are just starting to learn Python. It focuses on introducing fundamental concepts such as syntax, variables, data types, and basic input/output operations.
### Common Topics Covered in Python Lab 1:
1. **Introduction to Python Syntax:**
Learning how to write simple Python statements, understanding indentation, and exploring the basic structure of a Python program.
2. **Variables and Data Types:**
Understanding how to declare and assign values to variables, and working with different data types like integers, floats, strings, and booleans.
3. **Basic Input/Output:**
Using the `input()` function to get user input and the `print()` function to display output.
4. **Basic Operators:**
Performing arithmetic operations (addition, subtraction, multiplication, division), comparison operations (equal to, greater than, etc.), and logical operations (AND, OR).
5. **Simple Expressions and Statements:**
Writing expressions and using them in simple programs to compute values, concatenate strings, or check conditions.
### Example Exercise:
A common exercise might be asking students to write a simple program to add two numbers input by the user and print the result.
```python
# Python Lab 1: Simple addition program
# Get user input
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
# Add the numbers
sum = num1 + num2
# Print the result
print("The sum of", num1, "and", num2, "is", sum)
```
### Objective:
The goal of **Python Lab 1** is to give learners hands-on experience with the core concepts of Python and build confidence in writing basic programs.
### Common Topics Covered in Python Lab 1:
1. **Introduction to Python Syntax:**
Learning how to write simple Python statements, understanding indentation, and exploring the basic structure of a Python program.
2. **Variables and Data Types:**
Understanding how to declare and assign values to variables, and working with different data types like integers, floats, strings, and booleans.
3. **Basic Input/Output:**
Using the `input()` function to get user input and the `print()` function to display output.
4. **Basic Operators:**
Performing arithmetic operations (addition, subtraction, multiplication, division), comparison operations (equal to, greater than, etc.), and logical operations (AND, OR).
5. **Simple Expressions and Statements:**
Writing expressions and using them in simple programs to compute values, concatenate strings, or check conditions.
### Example Exercise:
A common exercise might be asking students to write a simple program to add two numbers input by the user and print the result.
```python
# Python Lab 1: Simple addition program
# Get user input
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
# Add the numbers
sum = num1 + num2
# Print the result
print("The sum of", num1, "and", num2, "is", sum)
```
### Objective:
The goal of **Python Lab 1** is to give learners hands-on experience with the core concepts of Python and build confidence in writing basic programs.