filmov
tv
How to Convert a Hex String to int32 with 0x Prefix in C#
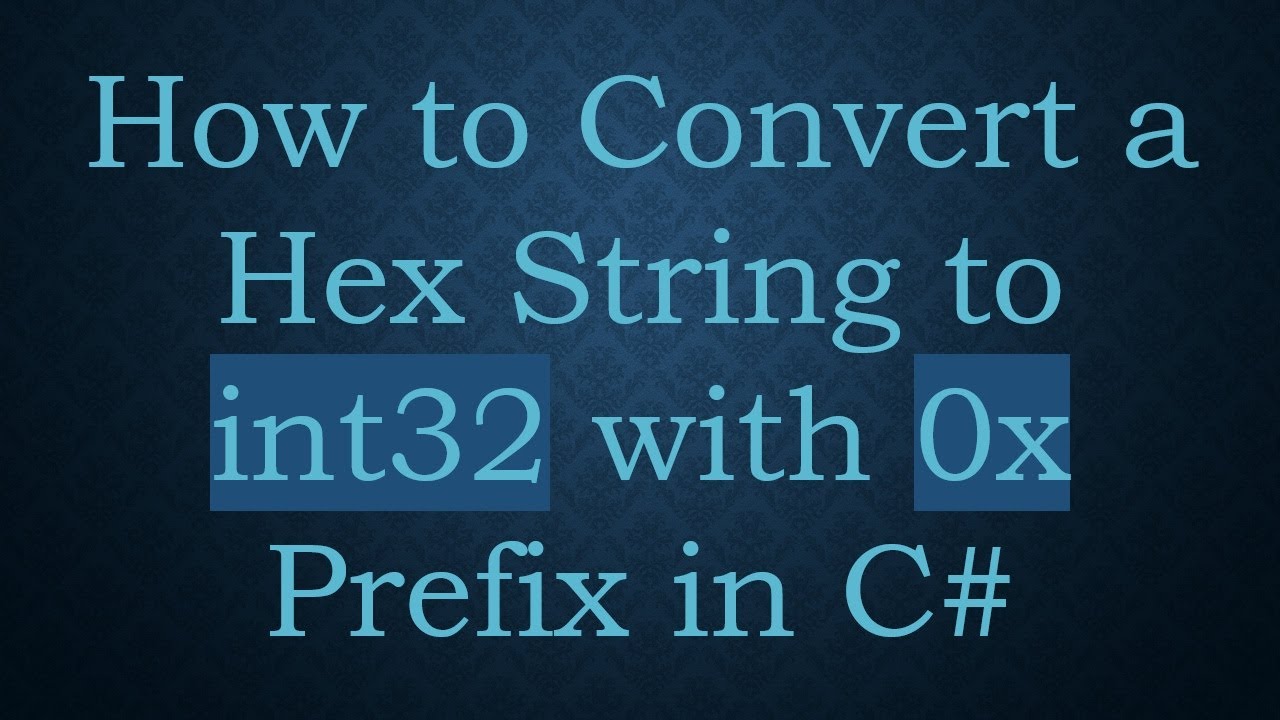
Показать описание
Learn how to easily convert a hex string like "0030FF" to an `int32` integer with a `0x` prefix in C# . You'll get a step-by-step guide to handle hex conversions effectively.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Convert hex string to int32 with 0x (C# )
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Convert a Hex String to int32 with 0x Prefix in C#
Working with hexadecimal values in C# can sometimes be tricky, especially when you want to ensure that you format them correctly as integers. If you've ever found yourself with a hex string such as "0030FF" and wondered how to convert it to an int32 integer with a 0x prefix, you’re not alone!
In this post, we'll break down the steps to achieve this conversion effectively.
The Problem: Hexadecimal to Integer Conversion
You might have a string representation of a hex number, like "0030FF", and you want to convert this into an integer format which looks like 0x0030FF. The challenge here is not just converting the string to an integer, but also formatting it correctly with the 0x prefix.
The Solution: Step-by-Step Conversion
Here’s how you can achieve the required conversion in C# :
Step 1: Prepare Your Hex String
The first part of your task involves specifying the hexadecimal value as a string. In your case, this is straightforward:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Convert Hex String to Integer
You'll use int.Parse() with System.Globalization.NumberStyles.HexNumber to convert the hex string into an int32. Here’s how you do it:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Format the Integer with 0x Prefix
Once you have the integer conversion of the hex string, you can format it to include the 0x prefix. You can accomplish this using an interpolated string in C# to ensure it’s correctly formatted:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Putting it all together, your complete code snippet to convert a hex string to an int32 with a 0x prefix would look like this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
int.Parse(value, System.Globalization.NumberStyles.HexNumber);: This line takes the hex string and converts it to an integer, interpreting it as a hexadecimal number.
$"0x{result:X6}": The X6 format specifier converts the integer back to a hex string, ensuring it's zero-padded to 6 characters, and prepends 0x to the string.
Conclusion
Converting a hex string to an int32 in C# while also adding a 0x prefix is a simple process thanks to the built-in features of the language. With the steps outlined above, you can effectively manage hex conversions and format them as needed.
Now, whether you are working on a small project or a larger application, you can confidently convert hex strings to integers in a way that meets your formatting requirements.
Feel free to reach out if you have any questions or run into issues while implementing this code!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Convert hex string to int32 with 0x (C# )
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Convert a Hex String to int32 with 0x Prefix in C#
Working with hexadecimal values in C# can sometimes be tricky, especially when you want to ensure that you format them correctly as integers. If you've ever found yourself with a hex string such as "0030FF" and wondered how to convert it to an int32 integer with a 0x prefix, you’re not alone!
In this post, we'll break down the steps to achieve this conversion effectively.
The Problem: Hexadecimal to Integer Conversion
You might have a string representation of a hex number, like "0030FF", and you want to convert this into an integer format which looks like 0x0030FF. The challenge here is not just converting the string to an integer, but also formatting it correctly with the 0x prefix.
The Solution: Step-by-Step Conversion
Here’s how you can achieve the required conversion in C# :
Step 1: Prepare Your Hex String
The first part of your task involves specifying the hexadecimal value as a string. In your case, this is straightforward:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Convert Hex String to Integer
You'll use int.Parse() with System.Globalization.NumberStyles.HexNumber to convert the hex string into an int32. Here’s how you do it:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Format the Integer with 0x Prefix
Once you have the integer conversion of the hex string, you can format it to include the 0x prefix. You can accomplish this using an interpolated string in C# to ensure it’s correctly formatted:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Putting it all together, your complete code snippet to convert a hex string to an int32 with a 0x prefix would look like this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
int.Parse(value, System.Globalization.NumberStyles.HexNumber);: This line takes the hex string and converts it to an integer, interpreting it as a hexadecimal number.
$"0x{result:X6}": The X6 format specifier converts the integer back to a hex string, ensuring it's zero-padded to 6 characters, and prepends 0x to the string.
Conclusion
Converting a hex string to an int32 in C# while also adding a 0x prefix is a simple process thanks to the built-in features of the language. With the steps outlined above, you can effectively manage hex conversions and format them as needed.
Now, whether you are working on a small project or a larger application, you can confidently convert hex strings to integers in a way that meets your formatting requirements.
Feel free to reach out if you have any questions or run into issues while implementing this code!