filmov
tv
Construct Binary Tree from Inorder and Postorder Traversal - Leetcode 106 - Python
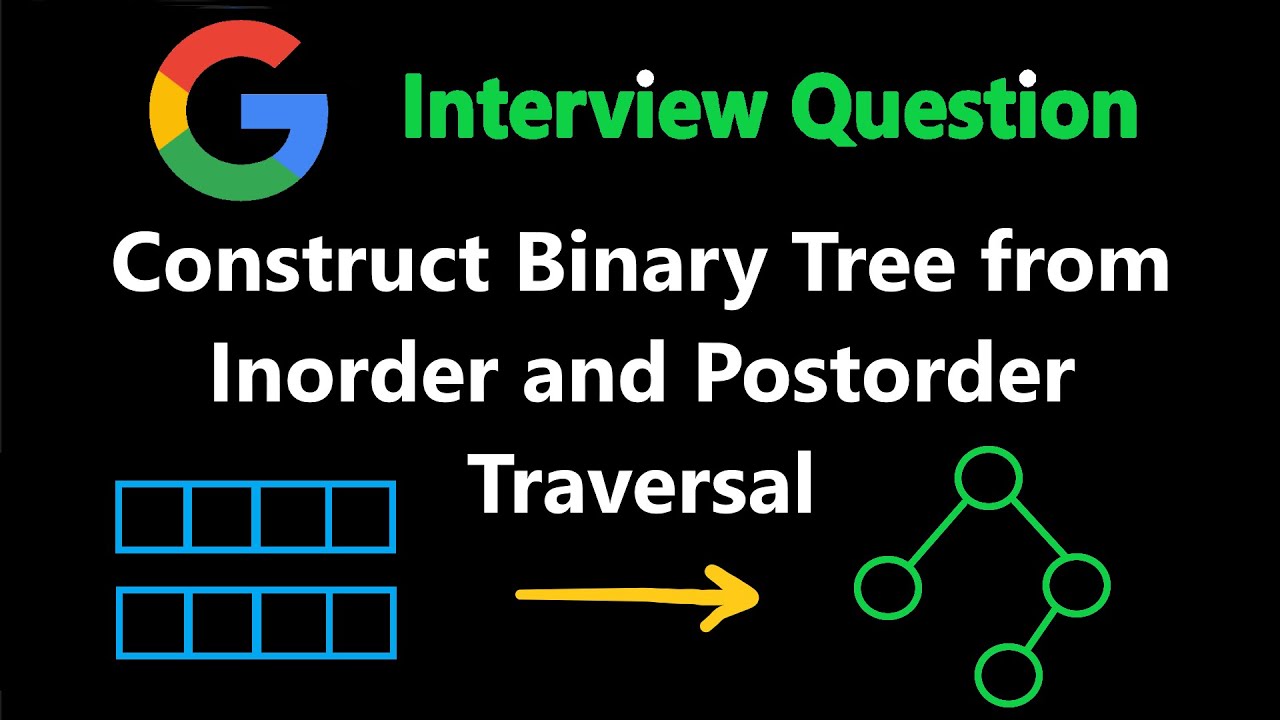
Показать описание
0:00 - Read the problem
0:30 - Drawing Explanation
7:45 - Coding Explanation
leetcode 106
#neetcode #leetcode #python
Construct Binary Tree from Inorder and Preorder Traversal - Leetcode 105 - Python
Construct Binary Tree from Inorder and Postorder Traversal - Leetcode 106 - Python
Leetcode - Construct Binary Tree from Inorder and Postorder Traversal (Python)
Construct Binary Tree From Inorder and Levelorder traversal | Trees
LeetCode 105. Construct Binary Tree from Preorder and Inorder Traversal (Algorithm Explained)
Construct Binary Tree From Preorder And Inorder Traversal - LeetCode 105
simplest way to find preorder given inorder and postorder | construct binary tree
Leetcode - Construct Binary Tree from Preorder and Inorder Traversal (Python)
L34. Construct a Binary Tree from Preorder and Inorder Traversal | C++ | Java
Create Binary Tree from pre-order and in-order traversal (LeetCode 105) | Easiest explanation
construct binary tree from inorder and postorder traversal | HINDI | Niharika Panda
[Java] Leetcode 106. Construct Binary Tree from Inorder and Postorder Traversal [Binary Tree #7]
Constructing binary tree from inorder and preorder traversal || Data Structures
Construct a binary tree from inorder and preorder in Hindi | Simple Shortcut method Data structure
L35. Construct the Binary Tree from Postorder and Inorder Traversal | C++ | Java
LeetCode 106 Construct Binary Tree from Inorder and Postorder Traversal
Construct Special Binary Tree from given Inorder traversal | GeeksforGeeks
Binary Tree Traversal : Inorder | #datastructure #datastructures #viral #shorts #short #shortsvideo
5.9 Construct Binary Tree from Preorder and Postorder traversal | Data Structure Tutorials
construct binary tree from inorder and postorder traversal examples
Learn Tree traversal in 3 minutes 🧗
LeetCode 105. Construct Binary Tree from Preorder and Inorder Traversal [Solution + Code Explained ]
Binary Tree Problems: Construct Tree from Inorder & Preorder | Tree from Postorder and Inorder
Binary Tree from Preorder & Inorder Traversal - Advance Tree Questions
Комментарии