filmov
tv
LeetCode Python Solutions: 283. Move Zeroes #coding #python
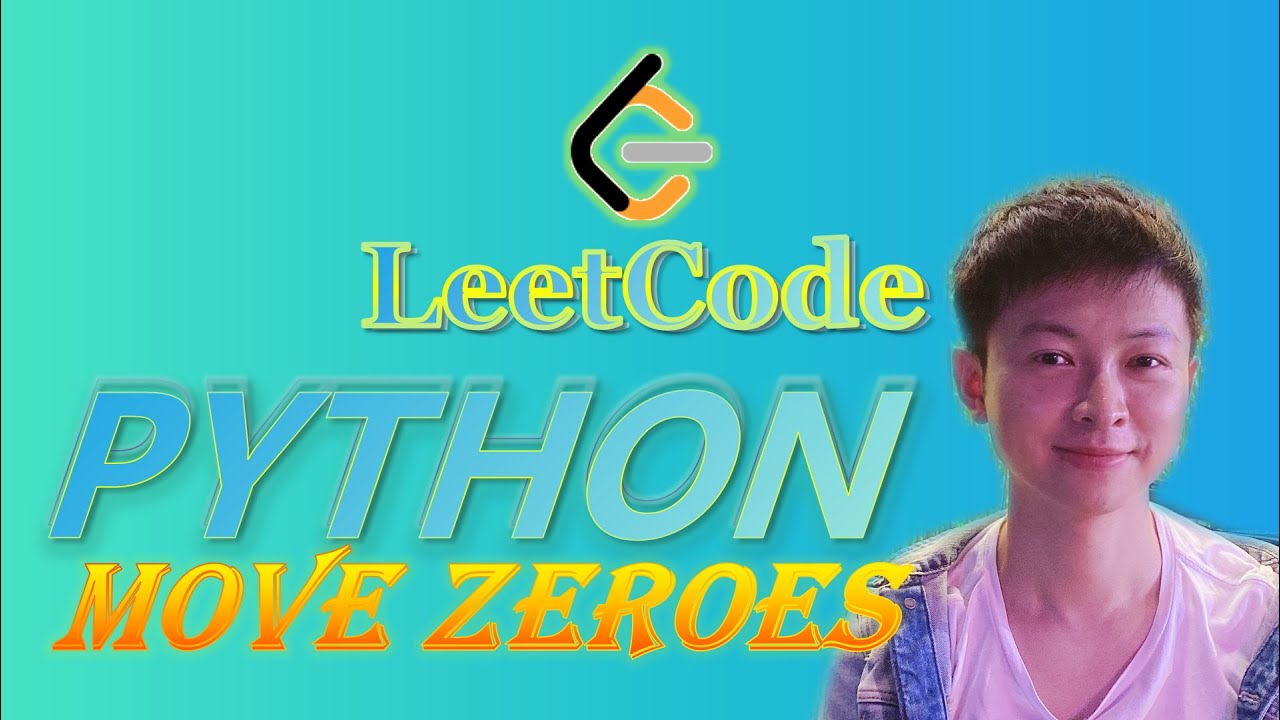
Показать описание
ZeroStress LeetCode Python Solutions: 283. Move Zeroes #coding #python #leetcode
Hello everyone, and welcome to this tutorial video on solving the 'Move Zeroes' LeetCode problem. In this video, we will explore an efficient approach to rearranging an array by moving all the zeroes to the end while maintaining the relative order of the non-zero elements.
I'm about to present to you a solution that utilizes a simple and intuitive algorithm. We will be using the power of pointers to efficiently
swap the elements within the array, allowing us to achieve the desired result in-place, without making a copy of the array.
Also, we will break down the problem step by step, explaining the logic, syntax, and mechanisms behind each line of code. By the end of this tutorial, you'll have a clear understanding of the solution and be able to implement it yourself.
Whether you're a beginner starting your coding journey or an experienced programmer looking to enhance your problem-solving skills, this video is for you. The 'Move Zeroes' problem is a common interview question, and understanding its solution will not only sharpen your programming abilities but also prepare you for similar challenges in the future.
So, without further ado, let's dive into the solution and unravel the intricacies of the 'Move Zeroes' problem. Please join me as we explore the fascinating world of algorithms and data manipulation to solve this coding challenge together.
How exciting, let's get started!
00:00 Code
02:21 Main
11:00 End
Hello everyone, and welcome to this tutorial video on solving the 'Move Zeroes' LeetCode problem. In this video, we will explore an efficient approach to rearranging an array by moving all the zeroes to the end while maintaining the relative order of the non-zero elements.
I'm about to present to you a solution that utilizes a simple and intuitive algorithm. We will be using the power of pointers to efficiently
swap the elements within the array, allowing us to achieve the desired result in-place, without making a copy of the array.
Also, we will break down the problem step by step, explaining the logic, syntax, and mechanisms behind each line of code. By the end of this tutorial, you'll have a clear understanding of the solution and be able to implement it yourself.
Whether you're a beginner starting your coding journey or an experienced programmer looking to enhance your problem-solving skills, this video is for you. The 'Move Zeroes' problem is a common interview question, and understanding its solution will not only sharpen your programming abilities but also prepare you for similar challenges in the future.
So, without further ado, let's dive into the solution and unravel the intricacies of the 'Move Zeroes' problem. Please join me as we explore the fascinating world of algorithms and data manipulation to solve this coding challenge together.
How exciting, let's get started!
00:00 Code
02:21 Main
11:00 End
Комментарии