filmov
tv
Solving Python dataclasses Circular Parsing Issues with Marshmallow
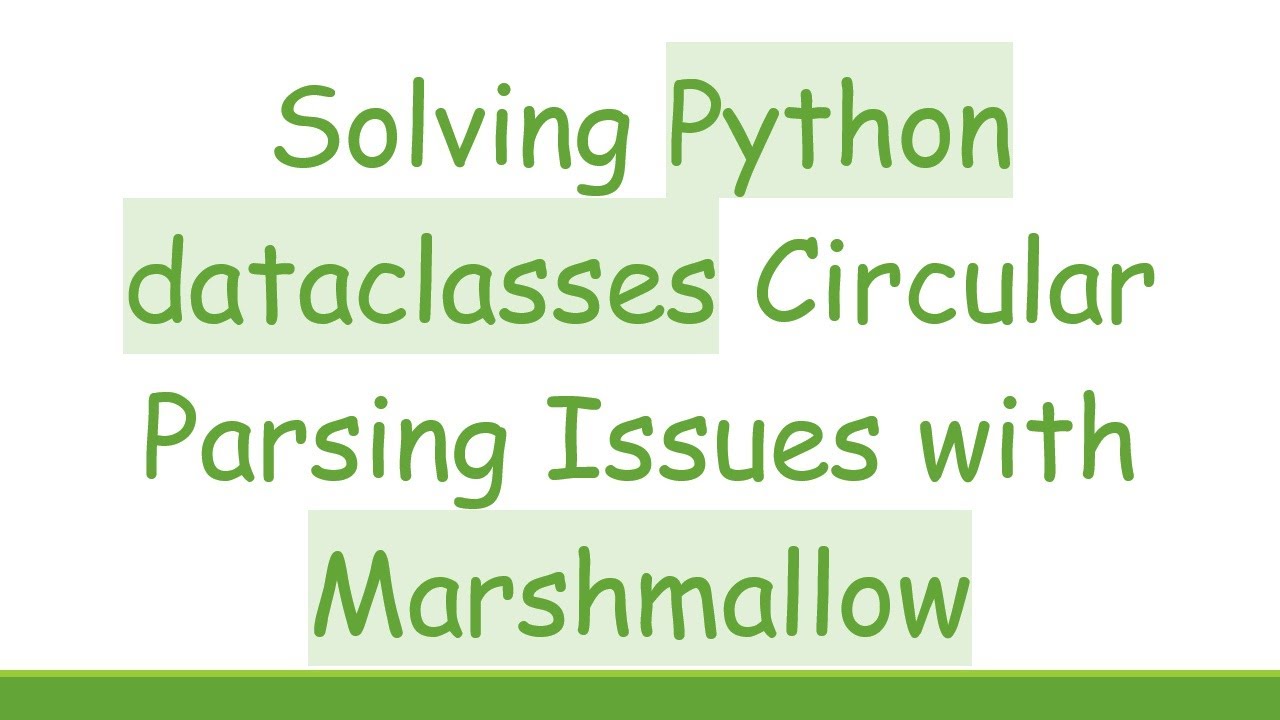
Показать описание
Learn how to handle circular parsing in Python dataclasses using Marshmallow and dataclasses_json projects with a custom decoder for your JSON data structures.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python dataclasses circular parsing with marshmallow
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Navigating Circular Parsing in Python Dataclasses with Marshmallow
As you work with JSON data structures, especially complex ones that may contain circular references, you might find yourself struggling to represent them neatly using Python's dataclasses. One common issue is when nested data structures fail to parse correctly. In this post, we will explore a solution to this problem by configuring a custom decoder for our dataclass fields using the dataclasses_json library.
Understanding the Problem
Consider the following scenario where you are trying to create a dataclass to represent a circular JSON structure. The structure might look something like this:
[[See Video to Reveal this Text or Code Snippet]]
When you attempt to parse this JSON data into a dataclass using the from_dict method, you might find that its nested components do not get transformed into instances of your defined dataclass, leading to unexpected behavior.
Example of the Problem
Here’s an example of the code you might be using, decorated with -dataclass_json:
[[See Video to Reveal this Text or Code Snippet]]
And when you call:
[[See Video to Reveal this Text or Code Snippet]]
You find that type contains basic string and dict types for nested objects instead of SchemaType instances.
Finding the Solution
The main issue here arises from the fact that dataclasses_json doesn't automatically recognize or decode mixed types within a list properly. To address this, we can utilize a custom decoder function that will handle the transformation of items appropriately.
Implementing the Custom Decoder
Here’s how you can implement the custom decoder using the config function from dataclasses_json:
Define the Decoder Function: Create a schemaTypeDecoder function that will manage the transformation of input data based on its type.
[[See Video to Reveal this Text or Code Snippet]]
Apply the Decoder to Your Dataclass Field: Use the field() function along with metadata=config(decoder=schemaTypeDecoder) to link your decoder with the type field of the SchemaType dataclass.
[[See Video to Reveal this Text or Code Snippet]]
Complete Working Example
Putting this all together, you now have a working dataclass that can manage circular structures correctly, thanks to your custom decoding logic.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
With your new custom decoder in place, the type field in the SchemaType will correctly parse nested structures into the specified dataclass instances rather than basic strings and dictionaries. This approach effectively handles the complexity of circular data representations, enabling you to leverage the full power of Python's dataclasses along with marshmallow and dataclasses_json.
Wrapping Up
If you find yourself dealing with complex JSON structures in your projects, utilizing custom decoders like this one can save you from parsing headaches, making your code cleaner and more efficient. Feel free to give this solution a try in your data parsing tasks!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python dataclasses circular parsing with marshmallow
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Navigating Circular Parsing in Python Dataclasses with Marshmallow
As you work with JSON data structures, especially complex ones that may contain circular references, you might find yourself struggling to represent them neatly using Python's dataclasses. One common issue is when nested data structures fail to parse correctly. In this post, we will explore a solution to this problem by configuring a custom decoder for our dataclass fields using the dataclasses_json library.
Understanding the Problem
Consider the following scenario where you are trying to create a dataclass to represent a circular JSON structure. The structure might look something like this:
[[See Video to Reveal this Text or Code Snippet]]
When you attempt to parse this JSON data into a dataclass using the from_dict method, you might find that its nested components do not get transformed into instances of your defined dataclass, leading to unexpected behavior.
Example of the Problem
Here’s an example of the code you might be using, decorated with -dataclass_json:
[[See Video to Reveal this Text or Code Snippet]]
And when you call:
[[See Video to Reveal this Text or Code Snippet]]
You find that type contains basic string and dict types for nested objects instead of SchemaType instances.
Finding the Solution
The main issue here arises from the fact that dataclasses_json doesn't automatically recognize or decode mixed types within a list properly. To address this, we can utilize a custom decoder function that will handle the transformation of items appropriately.
Implementing the Custom Decoder
Here’s how you can implement the custom decoder using the config function from dataclasses_json:
Define the Decoder Function: Create a schemaTypeDecoder function that will manage the transformation of input data based on its type.
[[See Video to Reveal this Text or Code Snippet]]
Apply the Decoder to Your Dataclass Field: Use the field() function along with metadata=config(decoder=schemaTypeDecoder) to link your decoder with the type field of the SchemaType dataclass.
[[See Video to Reveal this Text or Code Snippet]]
Complete Working Example
Putting this all together, you now have a working dataclass that can manage circular structures correctly, thanks to your custom decoding logic.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
With your new custom decoder in place, the type field in the SchemaType will correctly parse nested structures into the specified dataclass instances rather than basic strings and dictionaries. This approach effectively handles the complexity of circular data representations, enabling you to leverage the full power of Python's dataclasses along with marshmallow and dataclasses_json.
Wrapping Up
If you find yourself dealing with complex JSON structures in your projects, utilizing custom decoders like this one can save you from parsing headaches, making your code cleaner and more efficient. Feel free to give this solution a try in your data parsing tasks!