filmov
tv
Flutter Dependency Inversion For Beginners | Complete Guide
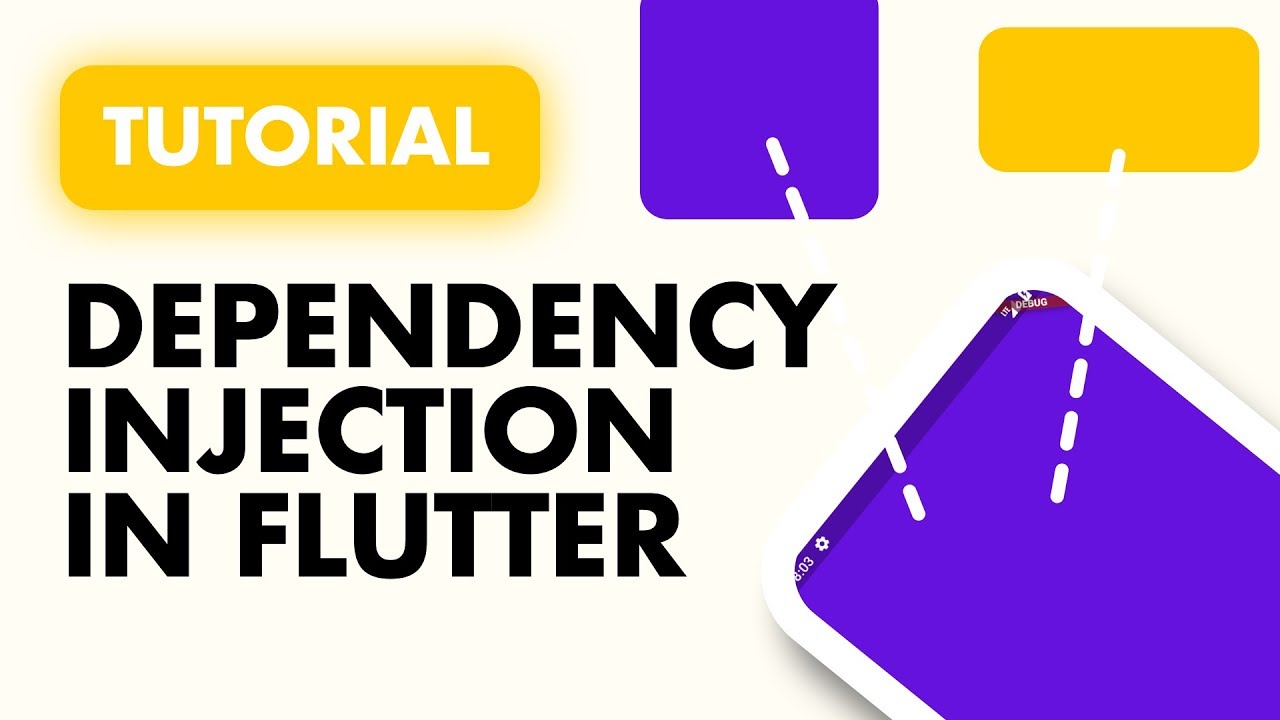
Показать описание
In this Flutter Dependency Injection Tutorial we go through three ways of setting up dependency injection in your code.
Flutter Dependency Inversion For Beginners | Complete Guide
Understanding Dependency Inversion Principle in 3 Minutes !
Dependency Injection in Flutter - You HAVE to Use it !
SOLID Dart / Flutter: Dependency Inversion Principle (DIP)
SOLID principles in Dart - Every PRO Coder Follows This!
Dependency Inversion Principle (DIP) - Raphael Barbosa
Dependency Injection in a Nutshell
What is Dependency Inversion Principle ?
Build A News App - Dependency Injection | PART 7 - Flutter Clean Architecture
Dependency Injection, The Best Pattern
Flutter GetX for Beginners | Dependency Injection
Dependency Inversion with real world example | What is Dependency Inversion?
Flutter Beginner Course | Navigation and Dependency Injection using Code generation
This Is NOT Dependency Injection
Complete Flutter App Course | Flutter Tutorial for Beginners | Dependency Injection (4)
Flutter Generated Dependency Injection – Kiwi Tutorial
Get_It package - Dependency Injection with Service Locator Pattern in Flutter & Dart
Dependency injection in flutter 💉
Flutter Tips #5 | Dependency injection with Gianfranco Papa
Dependency Inversion can make you a better developer
SOLID Principles in Arabic - #5 Dependency inversion
DEPENDENCY INVERSION PRINCIPLE | SOLID PRINCIPLES | LOW LEVEL DESIGN | SYSTEM DESIGN
What is Dependency Injection? | Why | Spring
Dependency injection in Flutter #flutter #dart #flutterdeveloper #firebase #youtubeshorts #code
Комментарии