filmov
tv
Java Game Programming Wizard Top Down Shooter Part 7
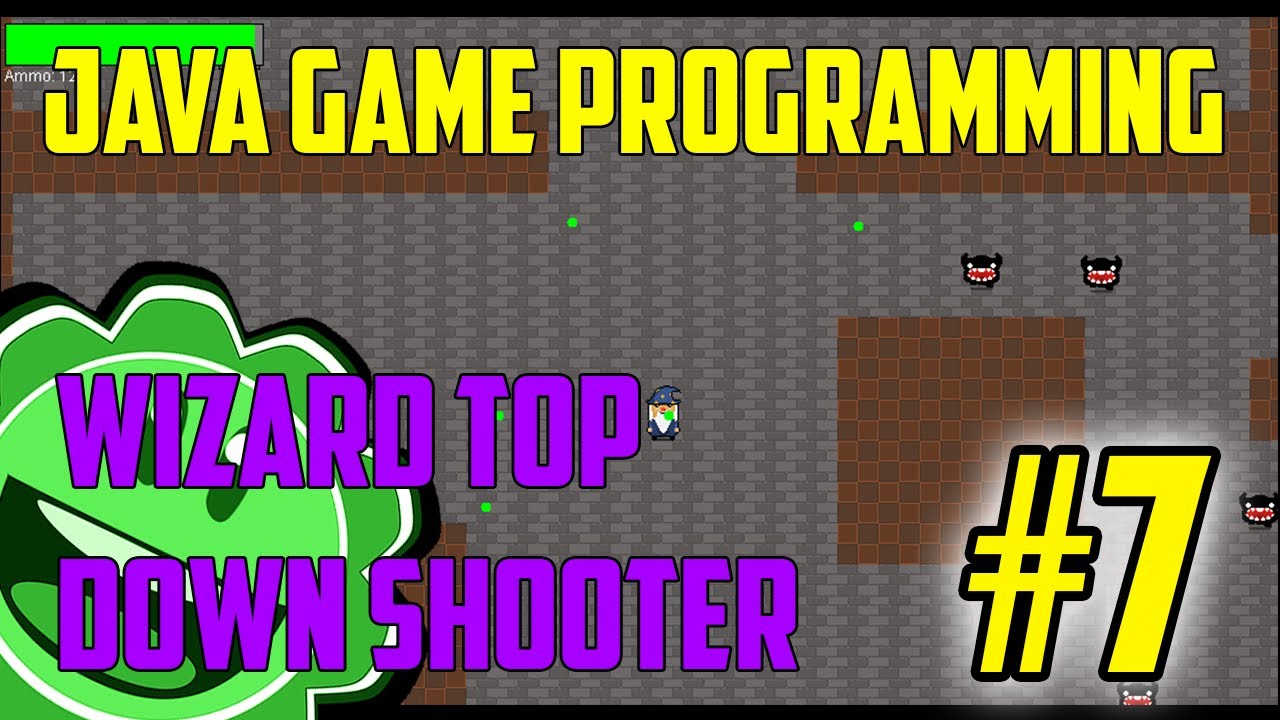
Показать описание
• Course Selection •
• Individual Game Design Courses •
Have fun learning!
Java Game Programming Wizard Top Down Shooter Part 1
Java Game Programming Wizard Top Down Shooter Part 2
Java Game Programming Wizard Top Down Shooter Part 5
Java Game Programming Wizard Top Down Shooter Part 3
Java Game Programming Wizard Top Down Shooter Part 9
Java Game Programming Wizard Top Down Shooter Part 4
Java Game Programming Wizard Top Down Shooter Part 10
Java Game Programming Wizard Top Down Shooter Part 8
Java Game Programming Wizard Top Down Shooter Part 11
Java Game Programming Wizard Top Down Shooter Part 12
Java Game Programming Wizard Top Down Shooter Part 13
Java Game Programming Wizard Top Down Shooter Part 14
Java Game Programming Wizard Top Down Shooter Part 7
Java Game Programming Wizard Top Down Shooter Part 6
3 Months of Game Programming in 20 Minutes
The HARDEST part about programming 🤦♂️ #code #programming #technology #tech #software #developer...
Code Wizard: Java Edition trailer
Satisfying ascii animation with C 😉 - The doughnut shaped code that generates a spinning 🍩
Serious Game - Word Wizard [Java]
IQ TEST
Normal People VS Programmers #coding #python #programming #easy #funny #short
I Tricked ChatGPT to Think 9 + 10 = 21
Create a Spiderman using python coding |python programer| #tech #python #coding
I Learned C++ In 24 Hours
Комментарии