filmov
tv
SELENIUM : What is an implicit wait in Selenium - SDET Automation Testing Interview
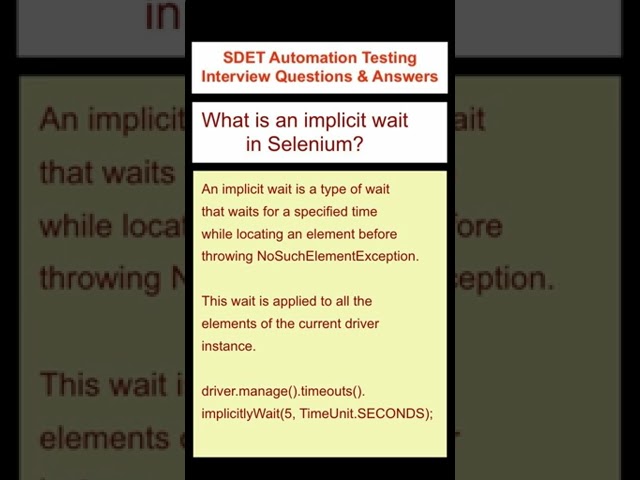
Показать описание
SELENIUM : What is an implicit wait in Selenium
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
An implicit wait in Selenium WebDriver is a wait time that is applied globally to all elements in a test script. It is a type of wait that is used to set a maximum time limit for the WebDriver to wait for a certain element to become available or clickable before throwing an exception.
The implicit wait is applied using the manage().timeouts().implicitlyWait() method of the WebDriver interface. The method takes an argument of type Duration or long and sets the implicit wait time for the WebDriver.
Here's an example code snippet that demonstrates how to set an implicit wait in Selenium WebDriver using Java:
public class ImplicitWaitExample {
public static void main(String[] args) {
// Create an instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Set the implicit wait time to 10 seconds
// Navigate to the desired URL
// Find the element with the ID "myElement"
// Perform an action on the element
// Close the browser
}
}
Then, we create an instance of the ChromeDriver and set the implicit wait time to 10 seconds using the implicitlyWait() method of the WebDriver.Options interface.
Next, we navigate to the desired URL using the get() method and find the element with the ID "myElement" using the findElement() method of the WebDriver interface and the By class.
We then perform an action on the element, in this case, clicking it using the click() method of the WebElement interface.
Finally, we close the browser using the quit() method of the WebDriver interface.
Note that the implicit wait time should be set to a reasonable value that is long enough to allow for the slowest loading element to load, but not too long that it slows down the test unnecessarily.
It is also important to note that the implicit wait time is applied globally to all elements, so it may cause a delay in the test script if it is set too high.
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
An implicit wait in Selenium WebDriver is a wait time that is applied globally to all elements in a test script. It is a type of wait that is used to set a maximum time limit for the WebDriver to wait for a certain element to become available or clickable before throwing an exception.
The implicit wait is applied using the manage().timeouts().implicitlyWait() method of the WebDriver interface. The method takes an argument of type Duration or long and sets the implicit wait time for the WebDriver.
Here's an example code snippet that demonstrates how to set an implicit wait in Selenium WebDriver using Java:
public class ImplicitWaitExample {
public static void main(String[] args) {
// Create an instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Set the implicit wait time to 10 seconds
// Navigate to the desired URL
// Find the element with the ID "myElement"
// Perform an action on the element
// Close the browser
}
}
Then, we create an instance of the ChromeDriver and set the implicit wait time to 10 seconds using the implicitlyWait() method of the WebDriver.Options interface.
Next, we navigate to the desired URL using the get() method and find the element with the ID "myElement" using the findElement() method of the WebDriver interface and the By class.
We then perform an action on the element, in this case, clicking it using the click() method of the WebElement interface.
Finally, we close the browser using the quit() method of the WebDriver interface.
Note that the implicit wait time should be set to a reasonable value that is long enough to allow for the slowest loading element to load, but not too long that it slows down the test unnecessarily.
It is also important to note that the implicit wait time is applied globally to all elements, so it may cause a delay in the test script if it is set too high.
Комментарии