filmov
tv
Sorting Algorithms in Python: Intersection of Two Sorted Arrays
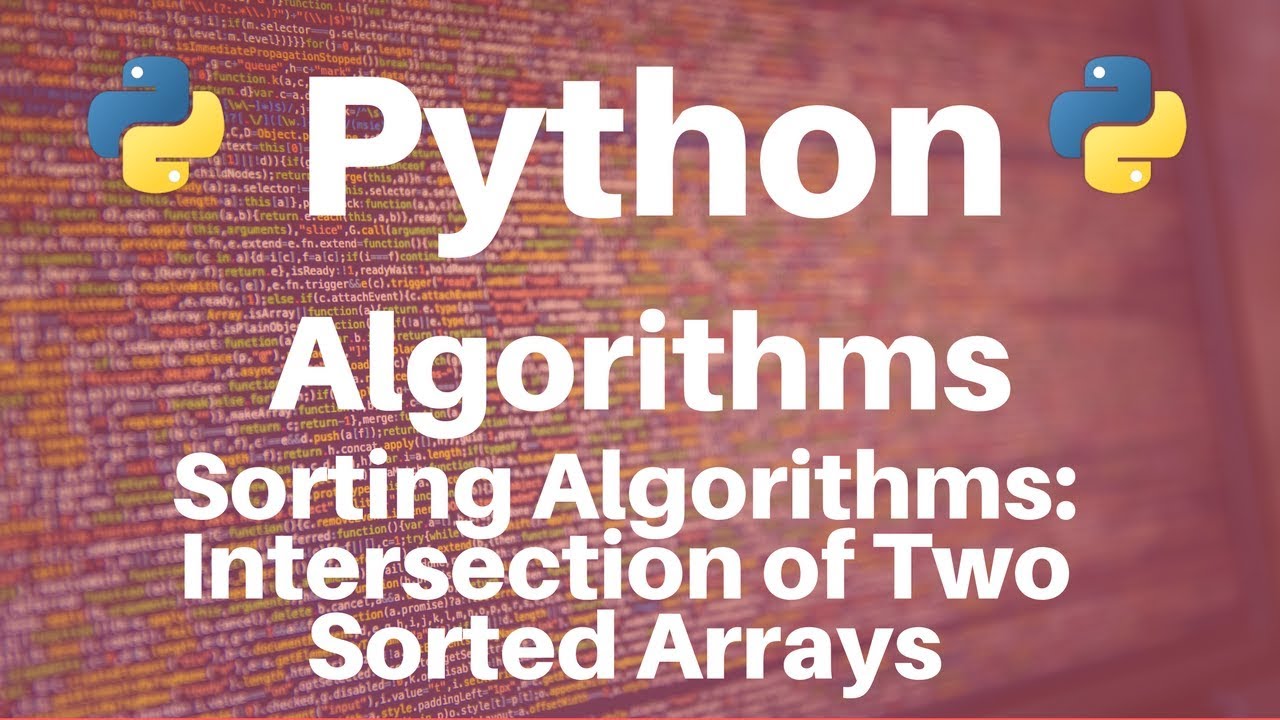
Показать описание
In this video, we will be solving the following problem:
Problem:
Given two arrays, A and B, determine their intersection. That is, what elements are common to A and B?
There is a one-line solution to this in Python that will also work in the event when the arrays are not sorted. However, since we are aware that the arrays A and B are sorted, we can use this to our advantage and solve the problem in a way that leverages this and gives us a slightly better runtime.
The software written in this video is available at:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
Problem:
Given two arrays, A and B, determine their intersection. That is, what elements are common to A and B?
There is a one-line solution to this in Python that will also work in the event when the arrays are not sorted. However, since we are aware that the arrays A and B are sorted, we can use this to our advantage and solve the problem in a way that leverages this and gives us a slightly better runtime.
The software written in this video is available at:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
Sorting Algorithms in Python: Intersection of Two Sorted Arrays
Insertion Sort In Python Explained (With Example And Code)
Bubble Sort in Python | Sorting Algorithms in Python
Sorting algorithms in python intersection of two sorted arrays
Insertion sort in 2 minutes
Insertion Sort | Simply Explained
Watch How Bubble Sort Algorithm Organizes Data in Seconds - Sorting Made Easy!
Learn Insertion Sort in 7 minutes 🧩
Insertion Sort Visualization
Bubble sort in 2 minutes
Insertion Sort In Python
İntersection of two list
Python: Numpy Functions: Sort,Unique,Union,Intersection etc: English
Quick Sort Explained - Algorithms & Data Structures #13
What are Sort,Unique,Union,Intersection etc functions in Python Numpy?:Lesson 11
Intersection method of set in python
That's how you find INTERSECTION of two lists in Python 🆒🆒😁😁
Sorting Algorithms | Bubble Sort, Selection Sort & Insertion Sort | DSA Series by Shradha Ma&apo...
Find the intersection of two lists #pythonprojects #pythonprogramming #pythontutorial #shorts
1 tip to improve your programming skills
Intersection Of Two Sorted Array part-2| DSA |Python-programming
Data Structure Sorting (Bubble, Insertion, Selection, Merge & Quick) | Learn Coding
3 Types of Algorithms Every Programmer Needs to Know
Python 3.7: Set Intersection in Python
Комментарии