filmov
tv
Learn JavaScript CLOSURES in 10 minutes! 🔒
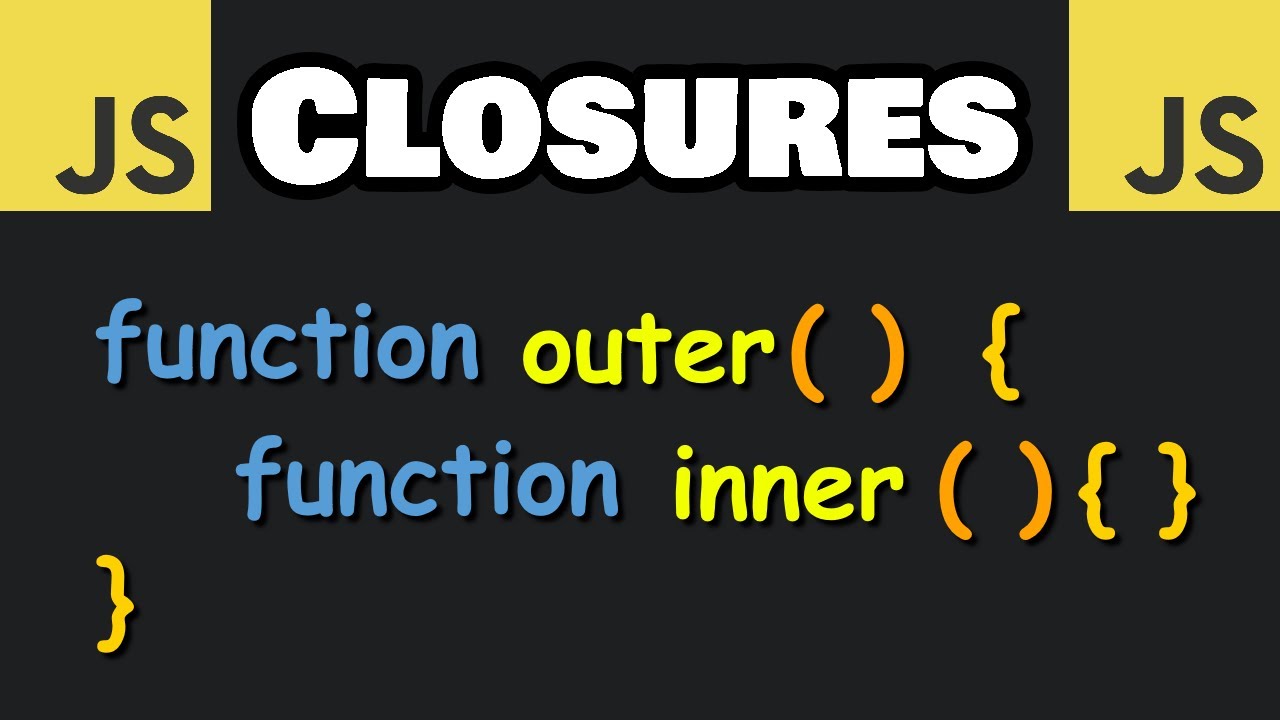
Показать описание
// closure = A function defined inside of another function,
// the inner function has access to the variables
// and scope of the outer function.
// Allow for private variables and state maintenance
// Used frequently in JS frameworks: React, Vue, Angular
00:00:00 intro
00:00:31 example 1
00:02:19 example 2
00:07:00 example 3
00:10:08 conclusion
// ---------- EXAMPLE 1 ----------
function outer(){
const message = "Hello";
function inner(){
}
inner();
}
message = "Goodbye";
outer();
// ---------- EXAMPLE 2 ----------
function createCounter() {
let count = 0;
function increment() {
count++;
}
function getCount() {
return count;
}
return {increment, getCount};
}
const counter = createCounter();
// ---------- EXAMPLE 3 ----------
function createGame(){
let score = 0;
function increaseScore(points){
score += points;
}
function decreaseScore(points){
score -= points;
}
function getScore(){
return score;
}
return {increaseScore, decreaseScore, getScore};
}
const game = createGame();
// the inner function has access to the variables
// and scope of the outer function.
// Allow for private variables and state maintenance
// Used frequently in JS frameworks: React, Vue, Angular
00:00:00 intro
00:00:31 example 1
00:02:19 example 2
00:07:00 example 3
00:10:08 conclusion
// ---------- EXAMPLE 1 ----------
function outer(){
const message = "Hello";
function inner(){
}
inner();
}
message = "Goodbye";
outer();
// ---------- EXAMPLE 2 ----------
function createCounter() {
let count = 0;
function increment() {
count++;
}
function getCount() {
return count;
}
return {increment, getCount};
}
const counter = createCounter();
// ---------- EXAMPLE 3 ----------
function createGame(){
let score = 0;
function increaseScore(points){
score += points;
}
function decreaseScore(points){
score -= points;
}
function getScore(){
return score;
}
return {increaseScore, decreaseScore, getScore};
}
const game = createGame();
Комментарии