filmov
tv
Graph -14: Check if Directed Graph has Cycle (Using InDegree/BFS)
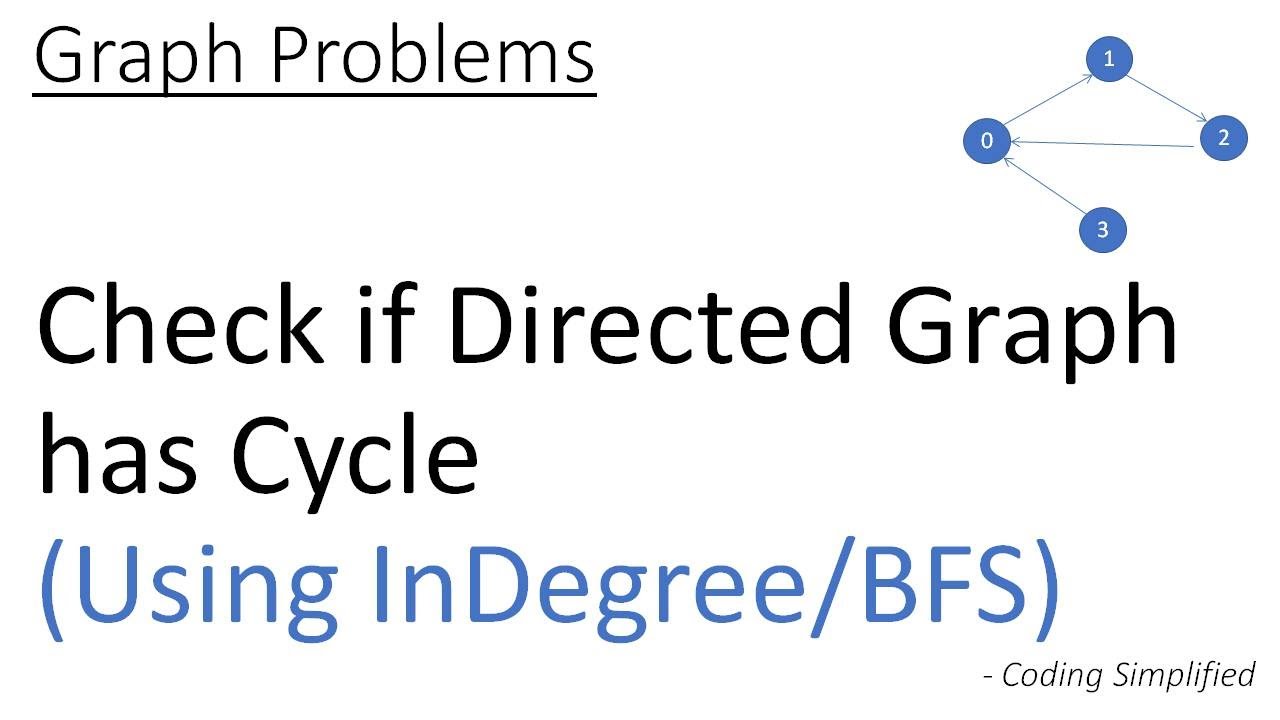
Показать описание
Solution:
- We'll achieve this via Indegree approach. We'll create hashmap, where key wil be index & value will be indegree for this index
- Now iterate the map & add all keys in queue for which indegree is 0
- Now poll the index form queue & get all children for this index, For each children decrease the indegree value by 1. Here if indegree becomes 0, add this in queue
- Every time you remove the item from queue, increase the visitedCount by 1
- Do this until queue is not empty
- At last if total nodes is not equal to visitedNodes, there exists a cycle in Graph
CHECK OUT CODING SIMPLIFIED
★☆★ VIEW THE BLOG POST: ★☆★
I started my YouTube channel, Coding Simplified, during Dec of 2015.
Since then, I've published over 400+ videos.
★☆★ SUBSCRIBE TO ME ON YOUTUBE: ★☆★
★☆★ Send us mail at: ★☆★
- We'll achieve this via Indegree approach. We'll create hashmap, where key wil be index & value will be indegree for this index
- Now iterate the map & add all keys in queue for which indegree is 0
- Now poll the index form queue & get all children for this index, For each children decrease the indegree value by 1. Here if indegree becomes 0, add this in queue
- Every time you remove the item from queue, increase the visitedCount by 1
- Do this until queue is not empty
- At last if total nodes is not equal to visitedNodes, there exists a cycle in Graph
CHECK OUT CODING SIMPLIFIED
★☆★ VIEW THE BLOG POST: ★☆★
I started my YouTube channel, Coding Simplified, during Dec of 2015.
Since then, I've published over 400+ videos.
★☆★ SUBSCRIBE TO ME ON YOUTUBE: ★☆★
★☆★ Send us mail at: ★☆★
Graph -14: Check if Directed Graph has Cycle (Using InDegree/BFS)
Detect cycle in a directed graph
Graph - 8: Check if Directed Graph is Strongly Connected
Detect Cycle in Directed Graph Algorithm
Detect Cycle In Directed Graph | Graphs Interview Prep
Graph -13: Check if Directed Graph has Cycle (Using DFS)
Topological Sort Algorithm | Graph Theory
Detect Cycle in Directed Graph | Cycle Detection Algorithm
Graph Theory, Lecture 35: Algebraic flows I: fundamentals
Detect cycle in a directed graph | Python Code | Graph Interview Problem
Detect cycle in directed graph | Competitive Programming | Graph | Geeksforgeeks
Detect Cycle in a Directed graph in C++
Cycle in Directed Graph #InterviewBit || DFS + BFS Kahn's Algorithm
Detect Cycle in Undirected Graph | Using DFS | Cycle detection in Graph | DSA-One Course #77
Coding Fundamentals: Course Schedule - Detect Cycle in Directed Graph (DFS)
5.13 Graph Data Structure : Detect Cycle in directed Graph (with coding)
G-11. Detect a Cycle in an Undirected Graph using BFS | C++ | Java
Graph Concepts & Qns - 6 (Flipkart, Amazon, Microsoft...) : Detect Cycle in Directed Graph using...
Detect a Cycle in Directed Graph
graph 12 cycle detection using BFS
[Algorithms] Negative weight edges and negative weight cycle in a directed graph
Topological Sort and Detect Cycle in a Directed Graph | leetcode course schedule ii | Java solution
Graph - 7: Check if Undirected Graph is Connected
Detect cycle in a directed graph || Geeks for Geeks
Комментарии