filmov
tv
Facebook Coding Interview Question and Answer #1: All Subsets of a Set
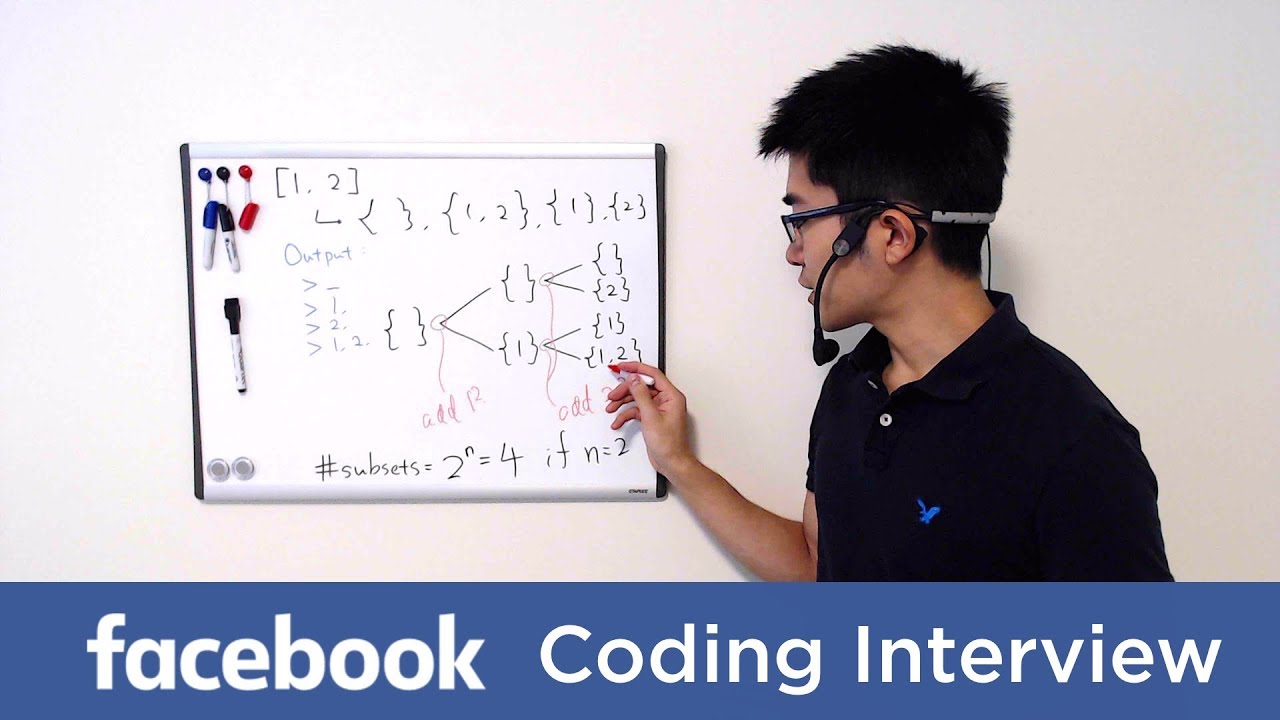
Показать описание
Find and print all subsets of a given set! (Given as an array.)
Practical Guide to LeetCode | How I Passed Facebook’s Coding Interviews
Facebook Coding Interview Question - First and Last Position of X in Sorted Array
Google Coding Interview With A Facebook Software Engineer
Facebook Coding Interview Question - findLongestSubarrayBySum
Facebook Coding Interview Question - How Many Ways to Decode This Message?
Solving My Facebook Phone Interview Question
Facebook Coding Interview Question - sortedSquaredArray
Facebook's favorite interview question
Coding Interviews Be Like
Coding Interview | Software Engineer @ Facebook
My Facebook Interview Experience (software engineer interview)
Facebook Coding Interview Question and Answer #1: All Subsets of a Set
The Facebook Data Science Interview Questions
I gave 127 interviews. Top 5 Algorithms they asked me.
Problem Solving Technique #1 for Coding Interviews with Google, Amazon, Microsoft, Facebook, etc.
ANYONE can Crack Coding Interviews by Doing THIS
Meta (Facebook) Machine Learning Mock Interview: Illegal Items Detection
Cracking the Facebook Coding Interview The Approach
🔴 Google Coding Interview with ex-Facebook ex-Stanford Co-Founder || Software Engineering Interview...
Coding Interview | Software Engineer @ Bloomberg (Part 1)
Facebook SQL Mock Technical Interview: Part 2
Hard SQL Interview Question From FACEBOOK | Data Science Coding Interviews (Popularity Percentage)
Facebook and Microsoft Data Science SQL interview question walkthrough (advanced)
How to Get Hired at META - META Interview Questions and Answers
Комментарии