filmov
tv
PYTHON WEEK-4 GRADED ASSIGNMENT
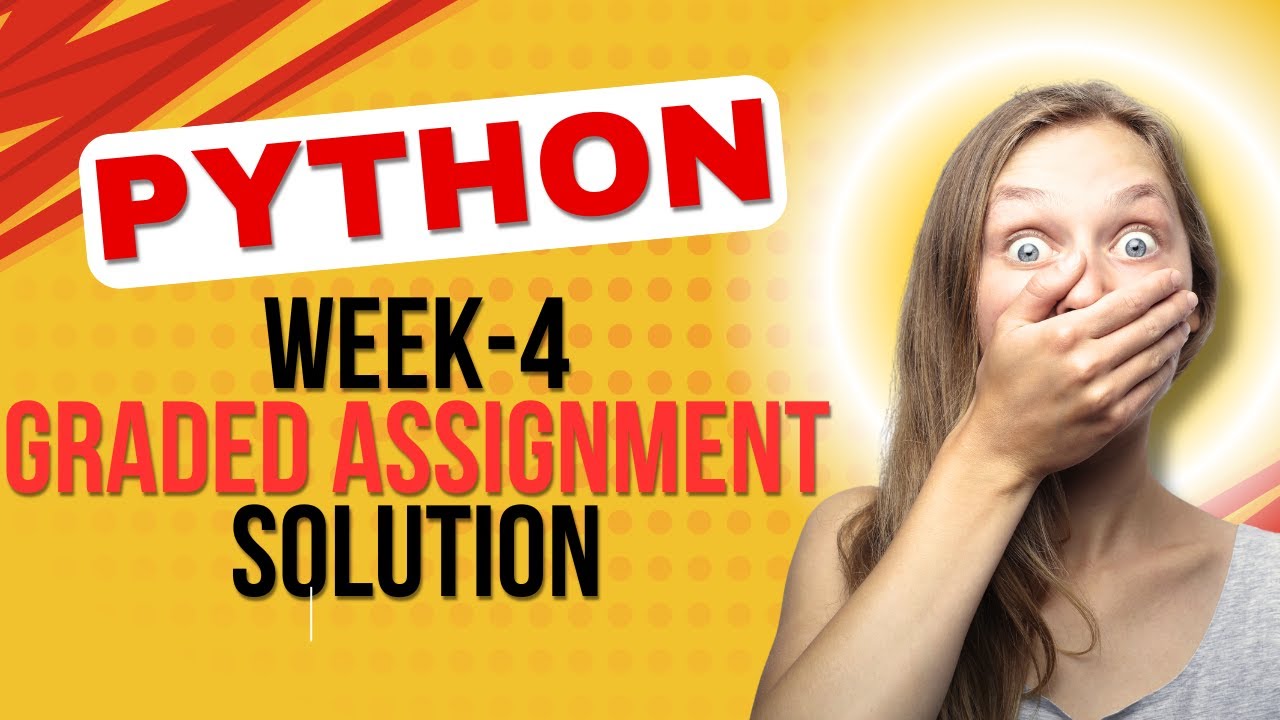
Показать описание
PYTHON WEEK-4 GRADED ASSIGNMENT
PYTHON | WEEK-4 | GRADED ASSIGNMENT | IITM
PYTHON WEEK-4 GRADED ASSIGNMENT
Week 4 Python Graded Solution | Foundational Level | IITM BS Data Science Degree
Python Week 4 Graded Assignment (IITM)
Python GA Solutions Week 4 | IITM BS Data Science | Graded Assignment Solution
Python Week 4 Graded Assignment
iitm python Graded Assignment Week 4 solutions
Python Week 4 Graded Assignment Solution // IITM BS Online Degree Program || Foundation
PROGRAMMING IN PYTHON || WEEK - 4 || GRADED ASSIGNMENT SOLUTION || IITM ONLINE BSC DEGREE COURSE
Python Week 4 Graded Assignment // IITM BS Online Degree Program || Foundation #python #gasolution
Python G.A. Week-4 IITM Bs degree Data Science #python#iitm#iit#datascience#video#ytshorts
PYTHON | WEEK-4 GRPA | 1,2,3,4,5 IITM
iitm Week 4 Python graded assignment #iitm #iitmadrasonlinedegree
Python Week 4 All GrPa's Solutions | IITM BS Data Science | Python
all subject week 4 graded assignments solutions
Coursera - Python Functions, Files, and Dictionaries - week 4 Graded Assessment 1 Solution
using database with python week 4 Many student in many cousre
IIT MADRAS Python WEEK 4 Grpa Assignment solution
Week 4 Graded Assignment Solution | DBMS,PDSA,JAVA,MAD 1,MAD 2,MLF,MLP,MLT,SC | IIT Madras BS | 4K
Coursera - Python Functions, Files, and Dictionaries - week 4 Graded Assessment 2 Solution
Python week-4 GrPA (Graded Programming Assignment) IITM
IITM PDSA GRADED ASSIGNMENT WEEK 4 SOLUTIONS
Week 4 Graded Assignment Solution | DBMS,PDSA,JAVA,MAD 1,MAD 2,MLF,MLP,MLT,SC | IIT Madras BS | 4K
Week 4 Graded Assignment Solution | Maths,Stats,CT,Python,English | IIT Madras BSc Data Science |4K
Комментарии