filmov
tv
Graph Valid Tree - Leetcode 261 - Python
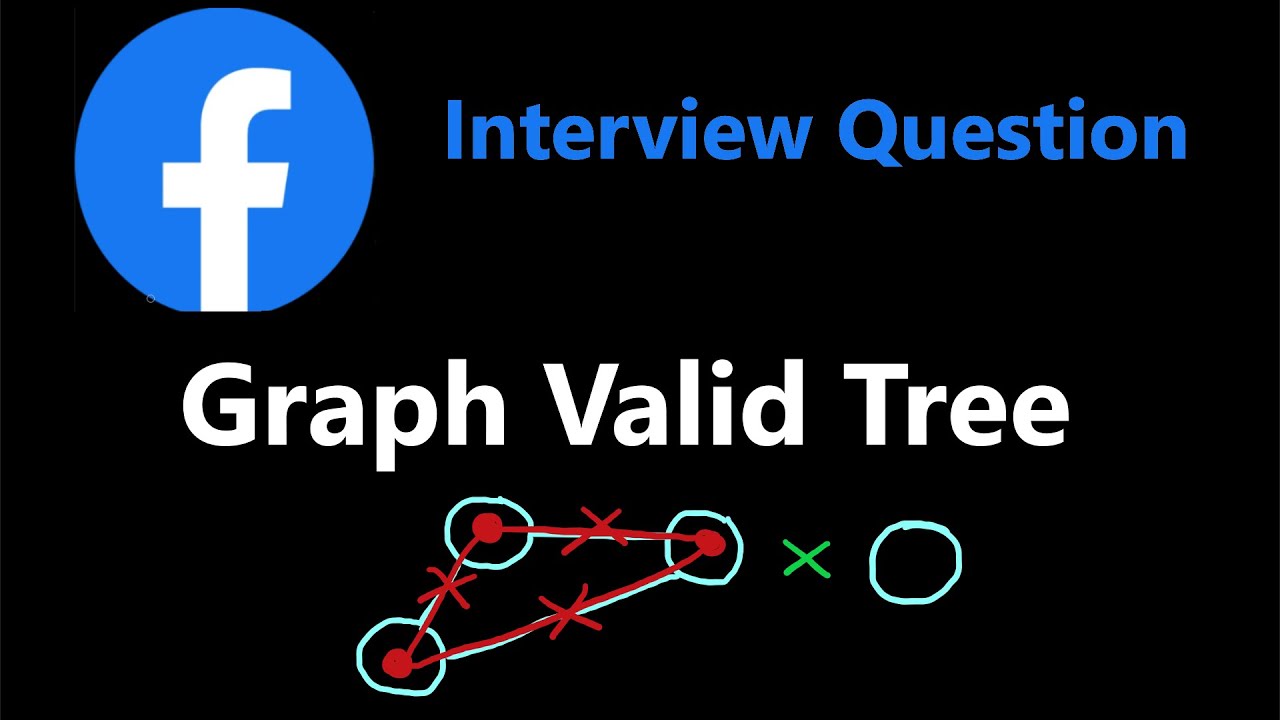
Показать описание
0:00 - Read the problem
2:45 - Drawing Explanation
10:06 - Coding Explanation
leetcode 261
#graph #tree #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Graph Valid Tree - Leetcode 261 - Python
Graph Valid Tree Using Union Find
261. Graph Valid Tree (Leetcode Medium)
LeetCode 261. Graph Valid Tree
Graph Valid Tree | LeetCode #261 (Google)
Graph Valid Tree - LeetCode 261 - JavaScript
Graph Valid Tree - Leetcode 261 - Python
LeetCode: 261. Graph Valid Tree (Golang & Java)
Graph Valid Tree - Leetcode 261- Blind 75 Explained - Graphs - Python
[Java] Leetcode 261. Graph Valid Tree [Union Find #2]
Graph Valid Tree || Leetcode
Leetcode 261. Graph Valid Tree | Cycle in Graph | DFS & Union-find
LEETCODE 261 GRAPH VALID TREE | leetcode graph
LEETCODE 261 (JAVASCRIPT) | GRAPH VALID TREE
Leetcode 261. Graph Valid Tree - Union-Find method
Leetcode Blind 75 C++: Graph Valid Tree
Check If Given Graph Is Tree Or Not
LeetCode 261. Graph Valid Tree | TechZoo Python
BFS Template : Graph Valid Trees ( Leetcode-261 )
LeetCode 261 | Graph Valid Tree | Union Find | Java
Graph Valid Tree - Leetcode 261 - Python
Leetcode 261. Graph Valid Tree (union find)
Graph Valid Tree | LeetCode Medium | Educative.io Day 116 | Graphs
LeetCode Graph Valid Tree - Python
Комментарии