filmov
tv
How to Create a Type Guard for Environment Variables in TypeScript
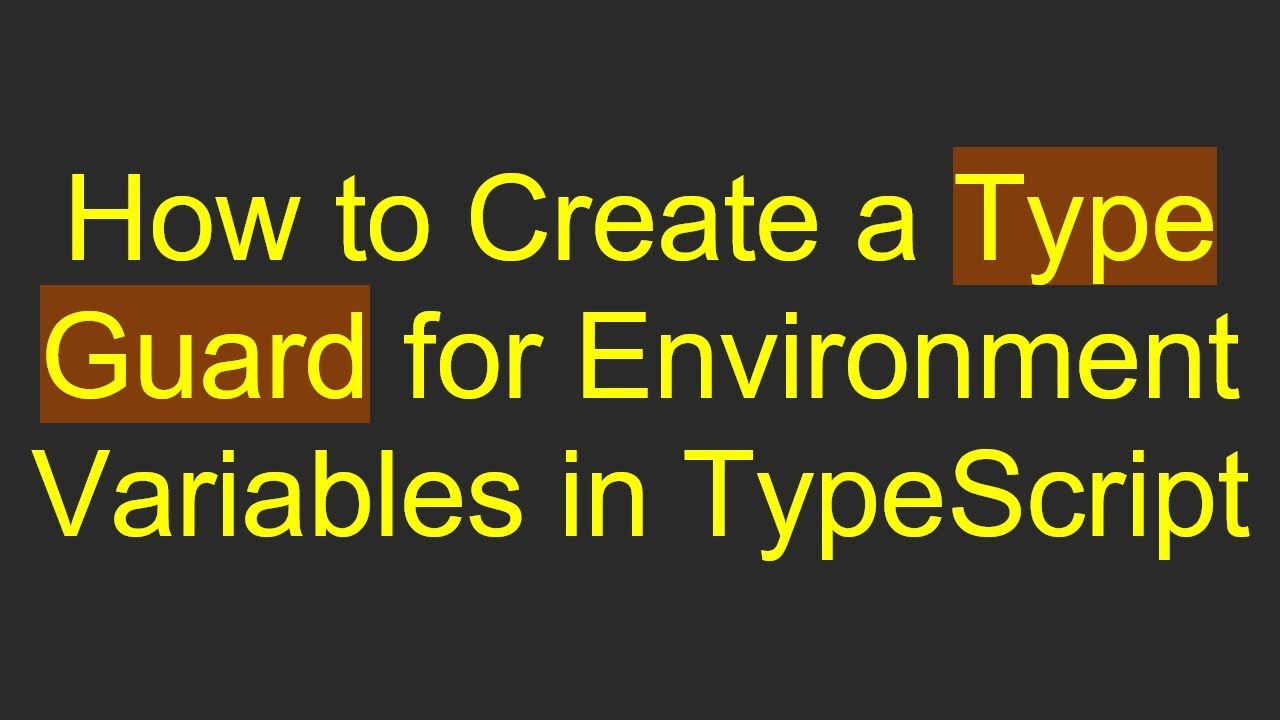
Показать описание
Learn how to effectively implement a `Type Guard` in TypeScript to handle environment variables as keys in a JSON object, ensuring type safety and eliminating runtime errors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Typescript: Type Guard for environment variable as key of JSON object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling Environment Variables in TypeScript with Type Guards
In today’s development environment, managing sensitive data like API keys, database URLs, and other configuration settings is a common task. However, when working with environment variables in TypeScript, you may run into type safety issues, particularly when attempting to use these variables as keys to access data in a JSON object. Here's a guide on how to effectively use Type Guards to ensure that you avoid potential runtime errors.
The Problem
You might find yourself in a situation where TypeScript throws an error like:
[[See Video to Reveal this Text or Code Snippet]]
This often occurs when TypeScript thinks that the environment variable you are using is just a generic string rather than a specific key of your JSON structure. The core of this issue lies in determining whether the environment variable is undefined or if it indeed matches a key defined in your JSON data.
Implementation Breakdown
Here’s how you can effectively solve this issue in TypeScript by implementing a custom Type Guard.
Step 1: Import Your JSON Data
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Define the Type Guard Function
Create a function named doesKeyExist that will serve as your Type Guard. This function checks if the provided input is a valid key in your JSON object.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Use the Type Guard
You will utilize the doesKeyExist function to validate the environment variable before attempting to use it. This will help ensure that you handle the case where the variable might be undefined or not present as a key.
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Access the Data Safely
Now that you've validated the environment variable, you can access your JSON data safely:
[[See Video to Reveal this Text or Code Snippet]]
Complete Implementation
Here is what your complete implementation should look like:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing a custom Type Guard, you can enhance type safety when using environment variables as keys in JSON objects within TypeScript. This not only prevents compile-time errors but also ensures that your application behaves as expected at runtime.
With this approach, you can confidently handle environment variables, mitigate risks, and improve the maintainability of your TypeScript application.
Remember, good practices in type safety can prevent potential issues that might arise from dynamic input, making your code robust and reliable.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Typescript: Type Guard for environment variable as key of JSON object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling Environment Variables in TypeScript with Type Guards
In today’s development environment, managing sensitive data like API keys, database URLs, and other configuration settings is a common task. However, when working with environment variables in TypeScript, you may run into type safety issues, particularly when attempting to use these variables as keys to access data in a JSON object. Here's a guide on how to effectively use Type Guards to ensure that you avoid potential runtime errors.
The Problem
You might find yourself in a situation where TypeScript throws an error like:
[[See Video to Reveal this Text or Code Snippet]]
This often occurs when TypeScript thinks that the environment variable you are using is just a generic string rather than a specific key of your JSON structure. The core of this issue lies in determining whether the environment variable is undefined or if it indeed matches a key defined in your JSON data.
Implementation Breakdown
Here’s how you can effectively solve this issue in TypeScript by implementing a custom Type Guard.
Step 1: Import Your JSON Data
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Define the Type Guard Function
Create a function named doesKeyExist that will serve as your Type Guard. This function checks if the provided input is a valid key in your JSON object.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Use the Type Guard
You will utilize the doesKeyExist function to validate the environment variable before attempting to use it. This will help ensure that you handle the case where the variable might be undefined or not present as a key.
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Access the Data Safely
Now that you've validated the environment variable, you can access your JSON data safely:
[[See Video to Reveal this Text or Code Snippet]]
Complete Implementation
Here is what your complete implementation should look like:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing a custom Type Guard, you can enhance type safety when using environment variables as keys in JSON objects within TypeScript. This not only prevents compile-time errors but also ensures that your application behaves as expected at runtime.
With this approach, you can confidently handle environment variables, mitigate risks, and improve the maintainability of your TypeScript application.
Remember, good practices in type safety can prevent potential issues that might arise from dynamic input, making your code robust and reliable.