filmov
tv
Hello World in C - Introduction to C Programming
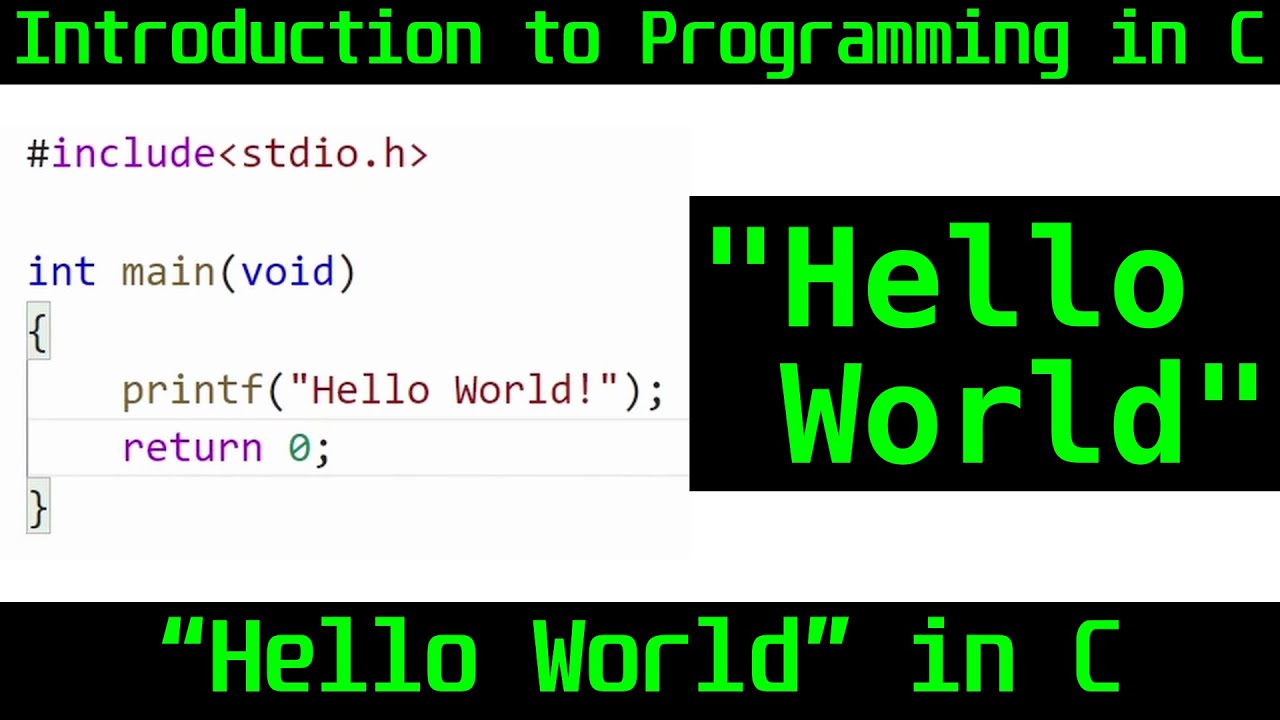
Показать описание
We will write a C program to print "Hello, World!" on the screen.
🔰 Show more for transcript 🔰
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
Any C Program can be divided into 5 basic sections:
1. Header
2. Main
3. Variable Declaration
4. Body
5. Return
At start of our program, we must include header files. Only the functions defined in the included header files can be used in the program. Since in our program we need to print something, we’ll use stdio.h file which defines core input and output functions. We do so using #include with the file name between angled brackets. #include is a preprocessor directory. It will include the file which is given within the angle brackets into the current source file. ‘.h’ extension means it is a header file. If we don’t include the header then our program won’t know the definition of printf and would throw an error.
Operating system (OS) initiates the program execution by invoking the main function. So In C, the execution typically begins with the first line of main().
A function is written in C as return_type function_name ( input / set_of _inputs ) Which here would be int main ( void ).
The int stands for integer because OS expects an integer value from the main function. That integer value represents the status of the program. If there is any error in the program while execution, the main function will return some non-zero value to the operating system. If the program executes successfully without any error, It will return zero. This void is not necessary but to write it is considered technically better as it clearly specifies that main can only be called without any parameter.
C is the block structured programming language where statements are grouped together to achieve specific task using curly braces. printf is a function defined in stdio.h header file. It will print everything to the screen which is given inside the double quotes which in this case is Hello World.
Every statement in C should end with semicolon;.
When we run the program it prints Hello World as output.
▬▬▬▬▬▬▬▬▬♫ ▬▬ ♪▬▬▬▬▬▬▬▬▬
🔰 Show more for transcript 🔰
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
Any C Program can be divided into 5 basic sections:
1. Header
2. Main
3. Variable Declaration
4. Body
5. Return
At start of our program, we must include header files. Only the functions defined in the included header files can be used in the program. Since in our program we need to print something, we’ll use stdio.h file which defines core input and output functions. We do so using #include with the file name between angled brackets. #include is a preprocessor directory. It will include the file which is given within the angle brackets into the current source file. ‘.h’ extension means it is a header file. If we don’t include the header then our program won’t know the definition of printf and would throw an error.
Operating system (OS) initiates the program execution by invoking the main function. So In C, the execution typically begins with the first line of main().
A function is written in C as return_type function_name ( input / set_of _inputs ) Which here would be int main ( void ).
The int stands for integer because OS expects an integer value from the main function. That integer value represents the status of the program. If there is any error in the program while execution, the main function will return some non-zero value to the operating system. If the program executes successfully without any error, It will return zero. This void is not necessary but to write it is considered technically better as it clearly specifies that main can only be called without any parameter.
C is the block structured programming language where statements are grouped together to achieve specific task using curly braces. printf is a function defined in stdio.h header file. It will print everything to the screen which is given inside the double quotes which in this case is Hello World.
Every statement in C should end with semicolon;.
When we run the program it prints Hello World as output.
▬▬▬▬▬▬▬▬▬♫ ▬▬ ♪▬▬▬▬▬▬▬▬▬