filmov
tv
Mastering Django QuerySets: Techniques to Extract Data Efficiently
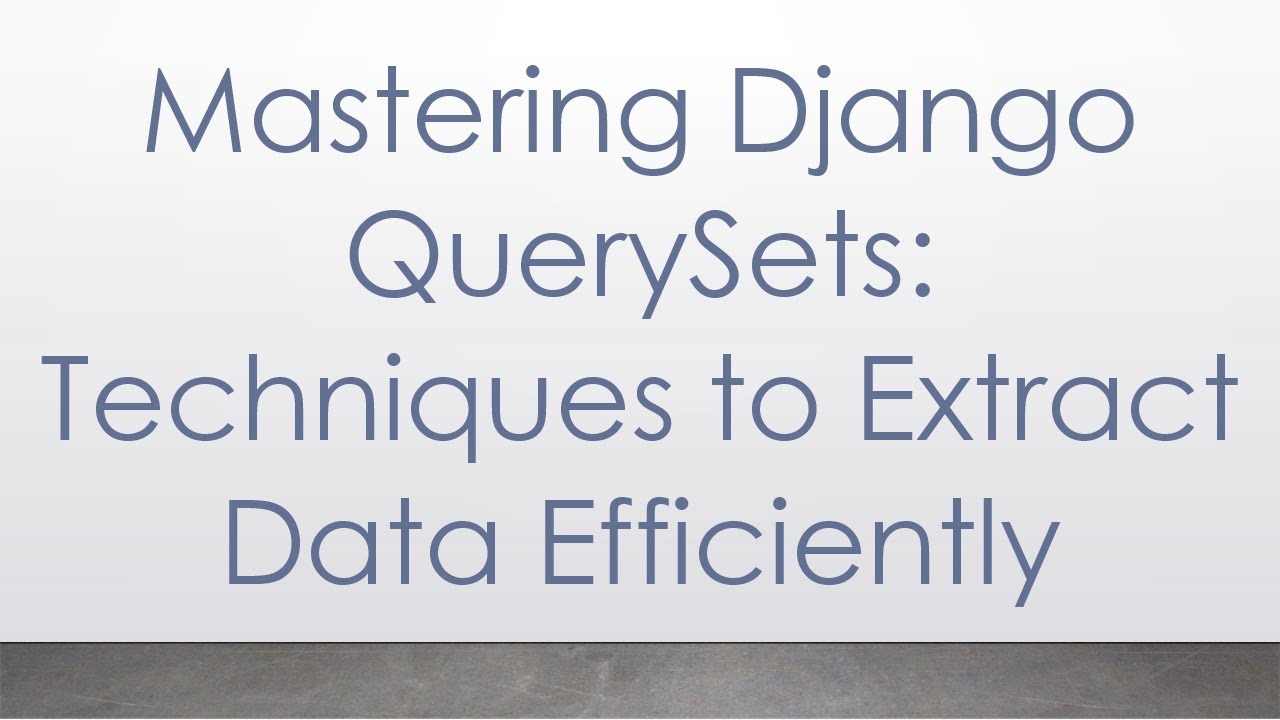
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn various techniques to extract data from QuerySets in Django, including how to get data, handle get_or_none scenarios, and more. Perfect for Python programmers aiming to enhance their Django skills.
---
Mastering Django QuerySets: Techniques to Extract Data Efficiently
How to Extract Data from a QuerySet in Django
A QuerySet in Django represents a collection of database queries. Understanding how to extract data from a QuerySet is crucial for database manipulation in any Django project. Here are some common and effective techniques:
Using .all() and .filter()
Retrieve All Records:
[[See Video to Reveal this Text or Code Snippet]]
The .all() method returns all records of a particular model.
Filter Records:
[[See Video to Reveal this Text or Code Snippet]]
The .filter() method returns a new QuerySet with objects that match the given lookup parameters.
How to Get Data from QuerySet in Django
One of the most frequent operations is fetching a specific object from the database. Here's how you can achieve that:
Using .get()
The .get() method is used to retrieve a single object matching certain lookup parameters.
[[See Video to Reveal this Text or Code Snippet]]
Pros: Ensures you're getting exactly one match.
Cons: Raises a MultipleObjectsReturned exception if more than one object matches the query.
Django QuerySet get_or_none
Unfortunately, Django doesn't provide a built-in get_or_none method, but it’s easy to define your own utility function.
Custom Implementation
Here’s a utility function that mimics get_or_none behavior:
[[See Video to Reveal this Text or Code Snippet]]
Usage:
[[See Video to Reveal this Text or Code Snippet]]
Combining .get() with Other QuerySet Methods
Sometimes, you might need to combine .get() with other QuerySet methods for more complex queries. Here’s an example:
Advanced Filtering:
[[See Video to Reveal this Text or Code Snippet]]
This approach first narrows down the list of objects with .filter() before using .get().
Conclusion
Extracting data from QuerySets in Django is a fundamental skill that involves knowing when and how to use various methods like .all(), .filter(), .get(), and custom utilities like get_or_none. Mastering these techniques ensures you can efficiently and safely handle your database queries, making your Django applications robust and reliable.
Whether you are a seasoned Django developer or just starting, having a solid understanding of these methods will boost your confidence and capability in managing your project's data layer.
Happy Coding! 🐍
---
Summary: Learn various techniques to extract data from QuerySets in Django, including how to get data, handle get_or_none scenarios, and more. Perfect for Python programmers aiming to enhance their Django skills.
---
Mastering Django QuerySets: Techniques to Extract Data Efficiently
How to Extract Data from a QuerySet in Django
A QuerySet in Django represents a collection of database queries. Understanding how to extract data from a QuerySet is crucial for database manipulation in any Django project. Here are some common and effective techniques:
Using .all() and .filter()
Retrieve All Records:
[[See Video to Reveal this Text or Code Snippet]]
The .all() method returns all records of a particular model.
Filter Records:
[[See Video to Reveal this Text or Code Snippet]]
The .filter() method returns a new QuerySet with objects that match the given lookup parameters.
How to Get Data from QuerySet in Django
One of the most frequent operations is fetching a specific object from the database. Here's how you can achieve that:
Using .get()
The .get() method is used to retrieve a single object matching certain lookup parameters.
[[See Video to Reveal this Text or Code Snippet]]
Pros: Ensures you're getting exactly one match.
Cons: Raises a MultipleObjectsReturned exception if more than one object matches the query.
Django QuerySet get_or_none
Unfortunately, Django doesn't provide a built-in get_or_none method, but it’s easy to define your own utility function.
Custom Implementation
Here’s a utility function that mimics get_or_none behavior:
[[See Video to Reveal this Text or Code Snippet]]
Usage:
[[See Video to Reveal this Text or Code Snippet]]
Combining .get() with Other QuerySet Methods
Sometimes, you might need to combine .get() with other QuerySet methods for more complex queries. Here’s an example:
Advanced Filtering:
[[See Video to Reveal this Text or Code Snippet]]
This approach first narrows down the list of objects with .filter() before using .get().
Conclusion
Extracting data from QuerySets in Django is a fundamental skill that involves knowing when and how to use various methods like .all(), .filter(), .get(), and custom utilities like get_or_none. Mastering these techniques ensures you can efficiently and safely handle your database queries, making your Django applications robust and reliable.
Whether you are a seasoned Django developer or just starting, having a solid understanding of these methods will boost your confidence and capability in managing your project's data layer.
Happy Coding! 🐍