filmov
tv
Efficiently Returning a Traversed Matrix using Recursion in Python
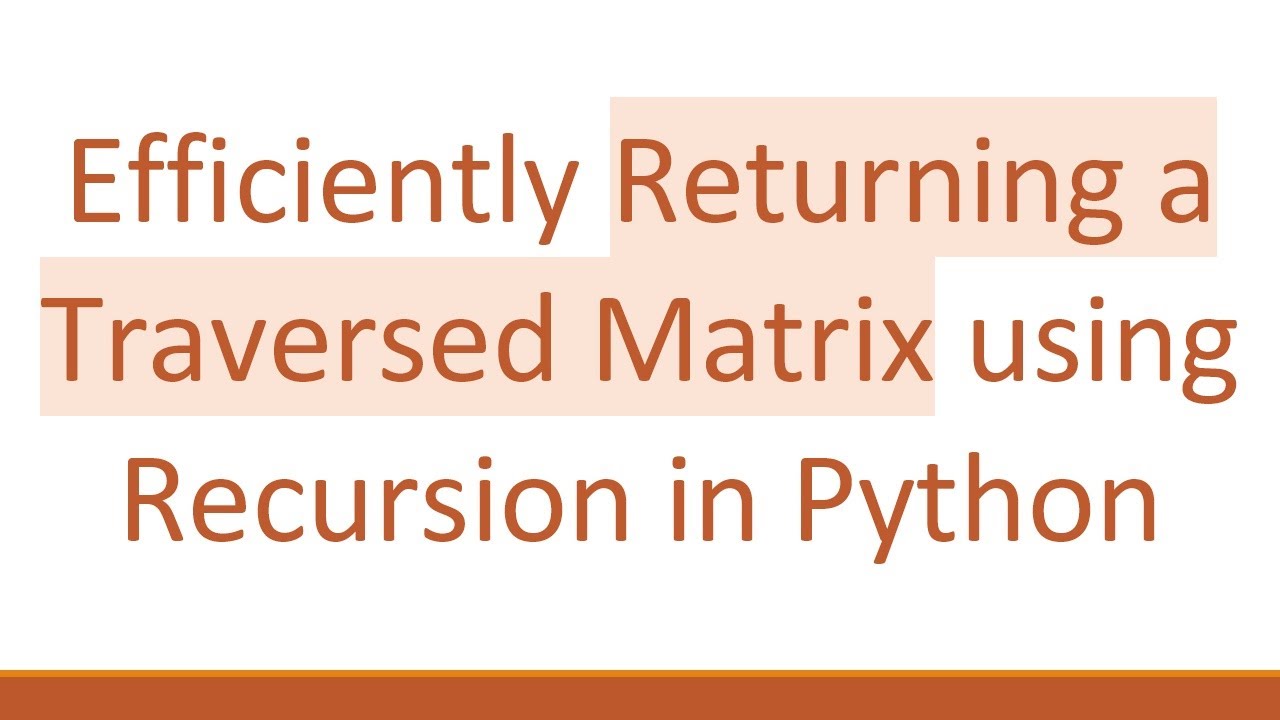
Показать описание
Learn how to traverse a matrix recursively in Python and return the results instead of printing them. Get step-by-step insights and examples!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python - Returning a traversed matrix using recursion
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Recursively Traversing a Matrix in Python
Traversing a matrix to collect or process its elements can be a common task in programming. While you can loop through a matrix using traditional iteration methods, recursion can be a fascinating and elegant way to achieve the same results.
In this guide, we'll show you how to traverse a matrix recursively and return the elements in a flattened structure. This will not only help you understand recursion better but also enhance your coding skills in Python.
Understanding the Problem
Let's start with a simple example. Suppose we have the following matrix:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to traverse this matrix and return the integers in a flat list format like this:
[[See Video to Reveal this Text or Code Snippet]]
While we could do this using loops, the challenge is to achieve it with recursion.
The Recursive Approach
In order to solve this problem using recursion, we need to create a method that utilizes a helper function to navigate through each element of the matrix. Here’s how we can break it down:
Step 1: Define the Main Function
We'll start by defining the main function that will call the helper function. It will initialize some important parameters:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create the Helper Function
The helper function does the actual work of traversing the matrix. It should take additional arguments for the current indices and an accumulator list to hold the matrix elements.
Here’s an outline of the helper function:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Putting it All Together
Now, let’s see how the entire code looks:
[[See Video to Reveal this Text or Code Snippet]]
Output
When you run the above code, you'll get the desired output:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In this post, we explored how to traverse a matrix recursively in Python and return the elements as a flattened list. By breaking down the problem into smaller parts and using a helper function, we were able to achieve a recursive solution effectively.
Using recursion not only keeps the code concise but also improves your problem-solving skills! Give it a try with different matrix sizes and elements to solidify your understanding.
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python - Returning a traversed matrix using recursion
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Recursively Traversing a Matrix in Python
Traversing a matrix to collect or process its elements can be a common task in programming. While you can loop through a matrix using traditional iteration methods, recursion can be a fascinating and elegant way to achieve the same results.
In this guide, we'll show you how to traverse a matrix recursively and return the elements in a flattened structure. This will not only help you understand recursion better but also enhance your coding skills in Python.
Understanding the Problem
Let's start with a simple example. Suppose we have the following matrix:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to traverse this matrix and return the integers in a flat list format like this:
[[See Video to Reveal this Text or Code Snippet]]
While we could do this using loops, the challenge is to achieve it with recursion.
The Recursive Approach
In order to solve this problem using recursion, we need to create a method that utilizes a helper function to navigate through each element of the matrix. Here’s how we can break it down:
Step 1: Define the Main Function
We'll start by defining the main function that will call the helper function. It will initialize some important parameters:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create the Helper Function
The helper function does the actual work of traversing the matrix. It should take additional arguments for the current indices and an accumulator list to hold the matrix elements.
Here’s an outline of the helper function:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Putting it All Together
Now, let’s see how the entire code looks:
[[See Video to Reveal this Text or Code Snippet]]
Output
When you run the above code, you'll get the desired output:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In this post, we explored how to traverse a matrix recursively in Python and return the elements as a flattened list. By breaking down the problem into smaller parts and using a helper function, we were able to achieve a recursive solution effectively.
Using recursion not only keeps the code concise but also improves your problem-solving skills! Give it a try with different matrix sizes and elements to solidify your understanding.
Happy coding!