filmov
tv
How to Check if a Python List Contains All Elements from Another List with Duplicates Not Ignored
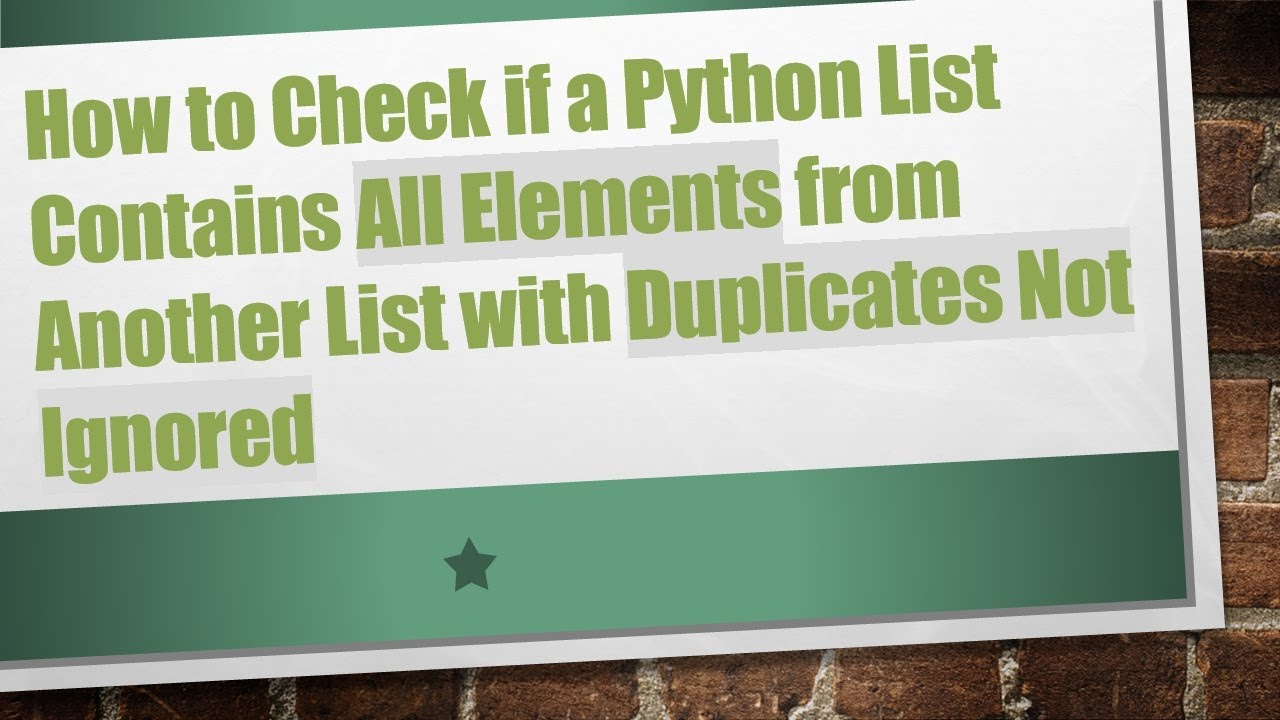
Показать описание
Learn how to effectively verify if all elements from one Python list are contained in another without ignoring duplicates. This guide offers code examples and clear explanations.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to Check if Python List Contains Elements of Another List Duplicates Not Ignored
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Check if a Python List Contains All Elements from Another List with Duplicates Not Ignored
In Python programming, list manipulation is one of the common tasks developers face. One intriguing problem is verifying whether a smaller list contains all elements of a larger list, with a particular focus on duplicity. In this post, we'll walk through two cases illustrating the challenge of checking list contents, along with a clear solution using Python's collections.Counter class.
Understanding the Problem
Consider the following scenarios:
Case 1
List 1: [97, 97, 196]
List 2: [97, 97, 101, 103, 196]
Here, the output should be True because all elements in List 1 are present in List 2, including the required duplicate of 97.
Case 2
List 1: [97, 97, 196]
List 2: [97, 101, 103, 196]
In this case, the output should be False. Although both lists contain 196, List 2 lacks the second occurrence of 97 from List 1.
This creates the need for a robust solution that effectively compares not just presence but also counts of elements.
Solution: Using collections.Counter
The most effective way to tackle this problem is by using the Counter class from Python's collections module. Counter enables us to count how many times each element appears in a list, which can help us verify whether one list contains all the elements of another, including their duplicates.
Code Implementation
Here's an example of how to implement this solution in Python:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Counting Elements: We initialize two Counter objects, one for each list. This allows us to easily retrieve the count of each element.
Checking Presence: The first if condition checks whether each element from list1 exists in list2. If not, we return False.
Verifying Counts: The second if condition ensures that the count of each element is sufficient in list2. If list2 has fewer occurrences than list1, returns False.
Returning Result: If all elements pass the checks, the function returns True, indicating that List 1's requirements are fulfilled in List 2.
Conclusion
By employing Python's collections.Counter, we can efficiently solve the problem of checking whether a smaller list contains all elements of a larger list, without ignoring duplicates. This approach not only simplifies the code but also enhances its readability and correctness.
Feel free to apply this technique to your own projects and streamline your list handling in Python!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to Check if Python List Contains Elements of Another List Duplicates Not Ignored
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Check if a Python List Contains All Elements from Another List with Duplicates Not Ignored
In Python programming, list manipulation is one of the common tasks developers face. One intriguing problem is verifying whether a smaller list contains all elements of a larger list, with a particular focus on duplicity. In this post, we'll walk through two cases illustrating the challenge of checking list contents, along with a clear solution using Python's collections.Counter class.
Understanding the Problem
Consider the following scenarios:
Case 1
List 1: [97, 97, 196]
List 2: [97, 97, 101, 103, 196]
Here, the output should be True because all elements in List 1 are present in List 2, including the required duplicate of 97.
Case 2
List 1: [97, 97, 196]
List 2: [97, 101, 103, 196]
In this case, the output should be False. Although both lists contain 196, List 2 lacks the second occurrence of 97 from List 1.
This creates the need for a robust solution that effectively compares not just presence but also counts of elements.
Solution: Using collections.Counter
The most effective way to tackle this problem is by using the Counter class from Python's collections module. Counter enables us to count how many times each element appears in a list, which can help us verify whether one list contains all the elements of another, including their duplicates.
Code Implementation
Here's an example of how to implement this solution in Python:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Counting Elements: We initialize two Counter objects, one for each list. This allows us to easily retrieve the count of each element.
Checking Presence: The first if condition checks whether each element from list1 exists in list2. If not, we return False.
Verifying Counts: The second if condition ensures that the count of each element is sufficient in list2. If list2 has fewer occurrences than list1, returns False.
Returning Result: If all elements pass the checks, the function returns True, indicating that List 1's requirements are fulfilled in List 2.
Conclusion
By employing Python's collections.Counter, we can efficiently solve the problem of checking whether a smaller list contains all elements of a larger list, without ignoring duplicates. This approach not only simplifies the code but also enhances its readability and correctness.
Feel free to apply this technique to your own projects and streamline your list handling in Python!