filmov
tv
How to Dynamically Remove DataFrames in Python with Ease
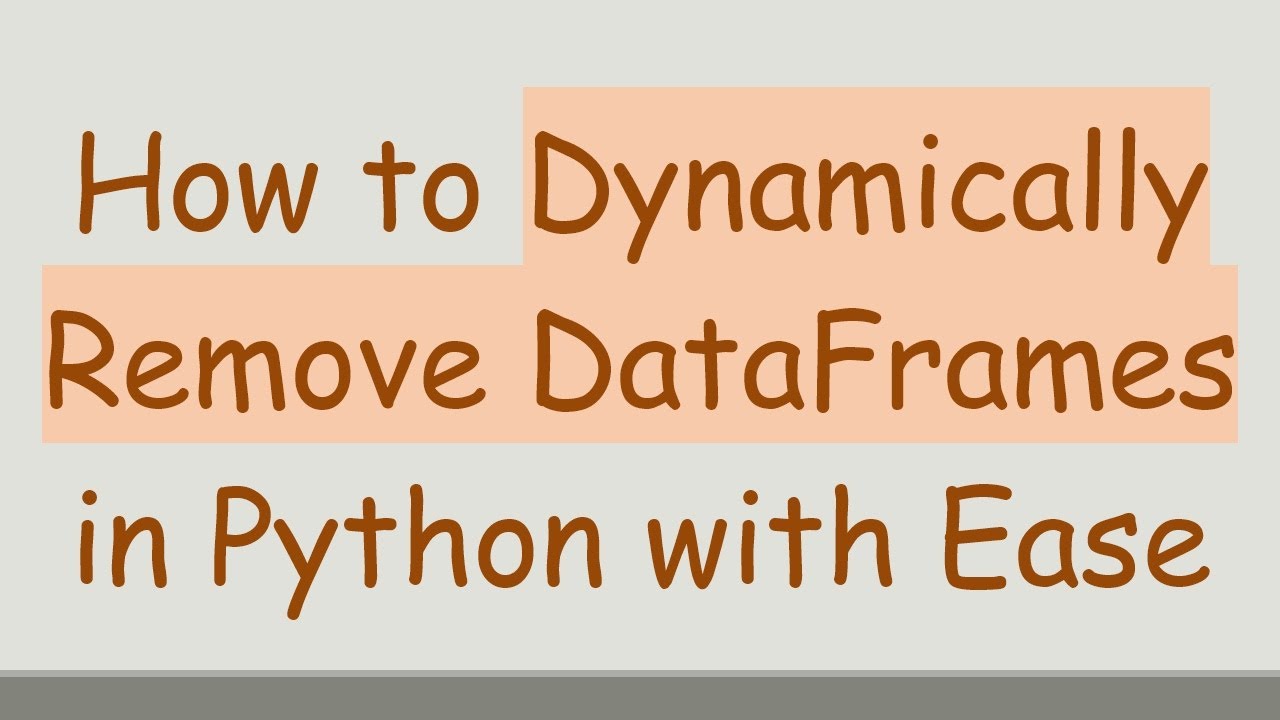
Показать описание
Learn how to efficiently remove DataFrames in Python using loops and the locals() function. This guide offers practical solutions for handling DataFrames in your workspace.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: remove the dataframe dynamically
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Dynamically Remove DataFrames in Python with Ease
Creating and managing DataFrames is a common task in data analysis with Python's pandas library. However, as you work with multiple DataFrames, there may come a time when you want to dynamically remove them. This guide explores the problem of deleting DataFrames from your workspace and provides practical solutions to achieve this efficiently.
The Problem: Removing DataFrames Individually
When you create multiple DataFrames—let's say df1, df2, and df3—you may often find yourself wanting to remove them individually to free up memory or because they are no longer needed. The straightforward approach involves using the del statement, like so:
[[See Video to Reveal this Text or Code Snippet]]
While this method works perfectly, it can quickly become tedious if you have many DataFrames. What's the solution? Let's dive into the dynamic approach.
The Attempted Dynamic Solution
You might consider using a loop to delete DataFrames dynamically based on their names stored in a list or obtained via the locals() function. Here is an initial attempt you might have made:
[[See Video to Reveal this Text or Code Snippet]]
Why This Fails
The above code is intended to find and delete all DataFrames in the current workspace. However, it fails at the last step because del var attempts to delete the variable name var itself rather than the DataFrame referred to by that name. This misunderstanding is common, but there's a better way to achieve your goal.
The Solution: Using locals() Correctly
To properly remove DataFrames dynamically, you'll want to delete the DataFrame objects themselves rather than just their names. Here's how to do it correctly:
Method 1: Simple Loop Through DataFrames
If you know the names of the DataFrames you want to delete, you can use a simple loop:
[[See Video to Reveal this Text or Code Snippet]]
This method is straightforward and clears the DataFrames effectively.
Method 2: Utilizing locals() Correctly
If you want to leverage locals() to dynamically delete all DataFrames present in your current workspace, use this refined loop:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
dir(): This function retrieves a list of names in the current local scope.
locals(): This function returns a dictionary mapping of the current local symbol table.
Deletion: By calling del locals()[var], you are accessing the DataFrame object by its name and deleting it.
Conclusion
Dealing with DataFrames efficiently is an essential skill for any data analyst or data scientist. By using the methods outlined above, you can dynamically remove DataFrames without the hassle of managing them one by one. Whether you prefer a hardcoded list or taking advantage of the Python locals() function, these solutions can help you streamline your coding workflow. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: remove the dataframe dynamically
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Dynamically Remove DataFrames in Python with Ease
Creating and managing DataFrames is a common task in data analysis with Python's pandas library. However, as you work with multiple DataFrames, there may come a time when you want to dynamically remove them. This guide explores the problem of deleting DataFrames from your workspace and provides practical solutions to achieve this efficiently.
The Problem: Removing DataFrames Individually
When you create multiple DataFrames—let's say df1, df2, and df3—you may often find yourself wanting to remove them individually to free up memory or because they are no longer needed. The straightforward approach involves using the del statement, like so:
[[See Video to Reveal this Text or Code Snippet]]
While this method works perfectly, it can quickly become tedious if you have many DataFrames. What's the solution? Let's dive into the dynamic approach.
The Attempted Dynamic Solution
You might consider using a loop to delete DataFrames dynamically based on their names stored in a list or obtained via the locals() function. Here is an initial attempt you might have made:
[[See Video to Reveal this Text or Code Snippet]]
Why This Fails
The above code is intended to find and delete all DataFrames in the current workspace. However, it fails at the last step because del var attempts to delete the variable name var itself rather than the DataFrame referred to by that name. This misunderstanding is common, but there's a better way to achieve your goal.
The Solution: Using locals() Correctly
To properly remove DataFrames dynamically, you'll want to delete the DataFrame objects themselves rather than just their names. Here's how to do it correctly:
Method 1: Simple Loop Through DataFrames
If you know the names of the DataFrames you want to delete, you can use a simple loop:
[[See Video to Reveal this Text or Code Snippet]]
This method is straightforward and clears the DataFrames effectively.
Method 2: Utilizing locals() Correctly
If you want to leverage locals() to dynamically delete all DataFrames present in your current workspace, use this refined loop:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
dir(): This function retrieves a list of names in the current local scope.
locals(): This function returns a dictionary mapping of the current local symbol table.
Deletion: By calling del locals()[var], you are accessing the DataFrame object by its name and deleting it.
Conclusion
Dealing with DataFrames efficiently is an essential skill for any data analyst or data scientist. By using the methods outlined above, you can dynamically remove DataFrames without the hassle of managing them one by one. Whether you prefer a hardcoded list or taking advantage of the Python locals() function, these solutions can help you streamline your coding workflow. Happy coding!