filmov
tv
ESP32 - Read DHT11 Temperature and humidity and publish to MQTT Broker
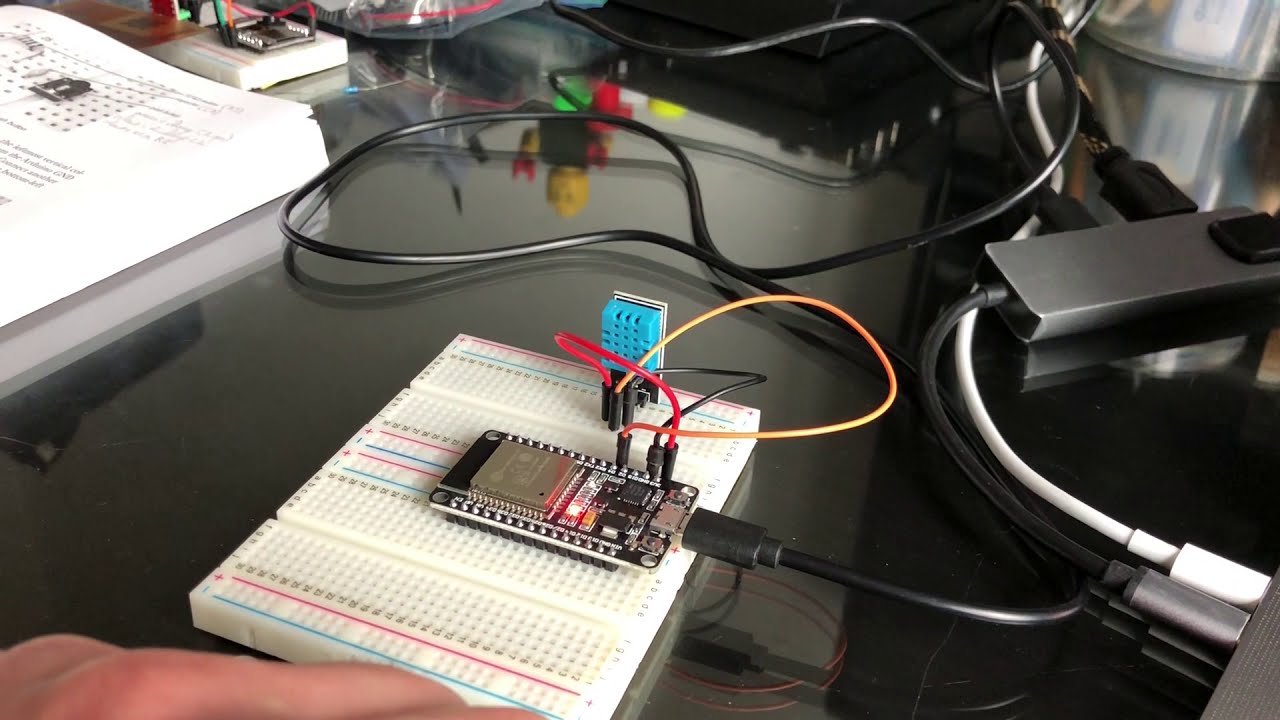
Показать описание
ESP32 - Read DHT11 Temperature and humidity and publish to MQTT Broker
ESP32 Tutorial - DHT11/DHT22 (Temperature and Humidity Sensor)
How to Use ESP32 with DHT11 Temperature and Humidity sensor and Arduino IDE
ESP32 DHT Web Server Project
ESP32 - Read DHT11 Temperature and humidity and publish to MQTT Broker
Temperature and Humidity Monitor Using ESP32 and Blynk IOT | Blynk 2.0 Projects
Reading live temperature and humidity using DHT11 with ESP32 on Ubidots website.
Arduino Tutorial 28 - DHT11 Temperature Sensor with LCD | SunFounder's ESP32 IoT Learning kit
Blynk ESP8266 DHT11 Temperature Sensor
ESP32 Based Webserver for Temperature and Humidity Monitor using DHT11 Sensor
How to use ESP8266 NodeMCU with DHT11 Temperature and Humidity Sensor
ESP32/ESP8266 Plot Sensor Readings in Real Time Charts - Web Server
ESP32: Temperature & Humidity Sensor with LCD Display
ESP32 with DHT11 Sensor | ESP32 Temperature Humidity Sensor | ESP32 Wether Station DIY | ESP32 DHT22
How To Interface ESP32 With DHT11 Temperature/Humidity Sensor & i2c LCD
ESP32 DHT11/DHT22 Asynchronous Web Server (auto updates Temperature and Humidity)
ESP32 with DHT11 Sensor | ESP32 Weather Station | ESP32 Temperature Meter | DHT11 with ESP32 | IOT
ESP32 DHT 11 Temperature & Humidity Reading in Google Sheet #teachmesomething #shorts
Measure Temperature and Humidity WiFi with ESP32 DHT11 and DHT22 - Robojax
Temp-Humidity reading by using ESP32 and DHT11 sensor ||Pramod Pawar|| ECE Vnit
ESP32 + DHT11 temperature & humidity sensor with display on ST7789 and BLE function
ESP32 DHT 11 Temperature and Humidity | 1.3' OLED Display
BT19ECE010_ANUP TEMPERATURE AND HUMIDITY READING WITH ESP32 AND DHT11 SENSOR
04 Easy IoT project w/ Arduino IoT Cloud - ESP32 | DHT11 Humidity & Temperature | Relay & DC...
BLYNK NEW Advanced IOT - Getting DHT11 & Analog Data | Temperature & Humidity Monitoring usi...
Комментарии