filmov
tv
Understanding Python Class Construction: __init__ vs. fromDict
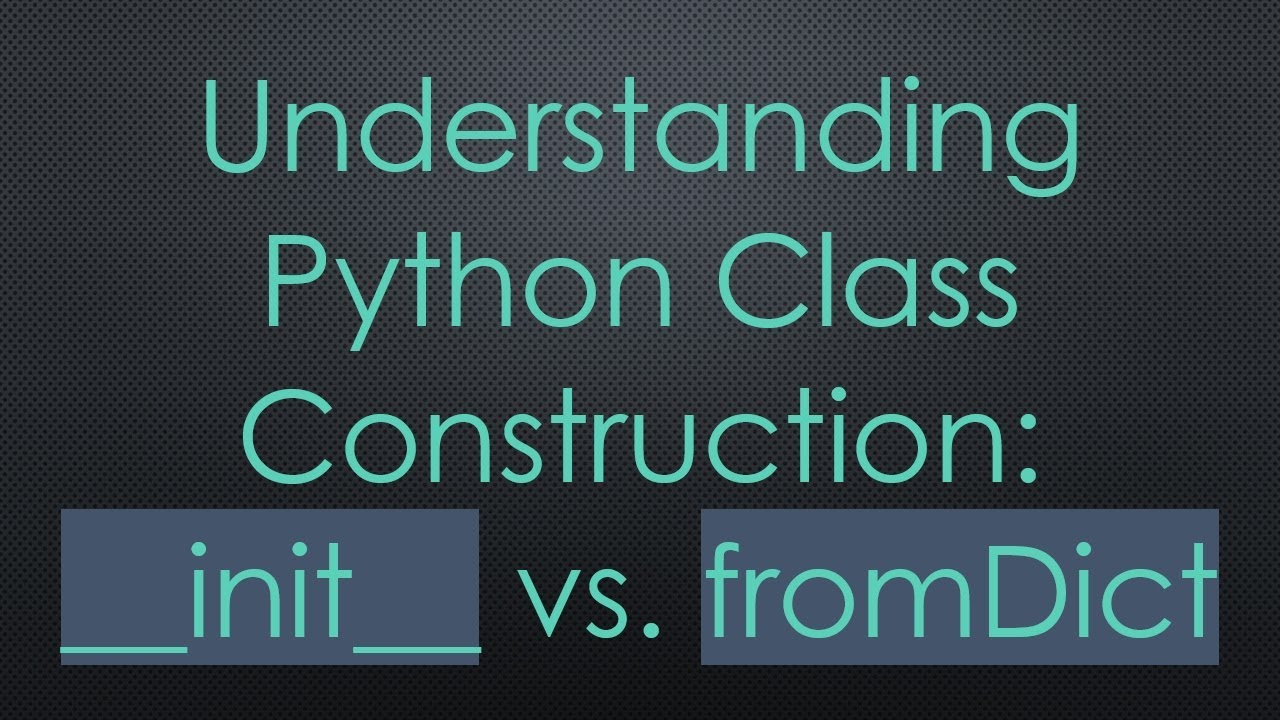
Показать описание
Discover the differences between Python's `__init__` and `fromDict` methods in class construction. Learn how to create class instances from dictionaries effectively without errors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python Class construction: why is my class constructed with __init__ different than the class constructed from dict using setattr?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python Class Construction: __init__ vs. fromDict
When working with Python classes, you may encounter situations where you want to create instances using both the traditional __init__ method and a dynamic construction method like fromDict. However, you might experience unexpected behavior or errors when trying to use these methods interchangeably. In this post, we will dive into the issue of class construction in Python and clarify why it happens and how to solve it effectively.
The Problem
You have a class A initialized with an __init__ method. Your goal is to create instances of A using both the __init__ method directly and dynamically using a method called fromDict. However, you encounter an error:
When an instance is created directly with a = A('Bar'), you can call a.aFunction() and it works fine.
But when you use _a = A.fromDict({'instanceVar': 'Bar'}) or __a = A.fromDict({}), calling _a.aFunction() or __a.aFunction() raises a TypeError indicating that a required positional argument, self, is missing.
What Went Wrong?
The root of the issue lies in how the fromDict method is defined. Instead of creating an instance of the class, it inadvertently tries to add attributes to the class itself. Let’s break down the original fromDict method:
[[See Video to Reveal this Text or Code Snippet]]
By returning the class itself rather than an instance of the class, the calls to other methods, such as aFunction(), do not have access to an instance object—resulting in the missing self error.
The Solution
To resolve this issue, the fromDict method should be modified to create an instance of the class properly. Here’s an updated version of the code that accomplishes this:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Changes
Setting attributes: The loop that sets the attributes iterates over the dictionary items and applies them to the instance (obj), not the class itself.
Returning the object: Finally, instead of returning cls, we return the newly created instance obj.
Advanced Usage: Including Additional Attributes
For more complex scenarios where you wish to handle additional attributes more gracefully, you might consider extending the __init__ method to accept variable keyword arguments (**kwargs). Here’s how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding how to correctly construct a class instance using different methods in Python is essential for effective programming. By clearly distinguishing between class-level attributes and instance-level attributes, you can avoid common pitfalls such as the TypeError we explored. Make sure to always create instances when needed and properly utilize the __init__ method for initialization. This practice will lead you to write more robust Python code.
With these insights into class construction, you can now confidently create class instances using either the __init__ method or a dynamic approach with fromDict. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python Class construction: why is my class constructed with __init__ different than the class constructed from dict using setattr?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python Class Construction: __init__ vs. fromDict
When working with Python classes, you may encounter situations where you want to create instances using both the traditional __init__ method and a dynamic construction method like fromDict. However, you might experience unexpected behavior or errors when trying to use these methods interchangeably. In this post, we will dive into the issue of class construction in Python and clarify why it happens and how to solve it effectively.
The Problem
You have a class A initialized with an __init__ method. Your goal is to create instances of A using both the __init__ method directly and dynamically using a method called fromDict. However, you encounter an error:
When an instance is created directly with a = A('Bar'), you can call a.aFunction() and it works fine.
But when you use _a = A.fromDict({'instanceVar': 'Bar'}) or __a = A.fromDict({}), calling _a.aFunction() or __a.aFunction() raises a TypeError indicating that a required positional argument, self, is missing.
What Went Wrong?
The root of the issue lies in how the fromDict method is defined. Instead of creating an instance of the class, it inadvertently tries to add attributes to the class itself. Let’s break down the original fromDict method:
[[See Video to Reveal this Text or Code Snippet]]
By returning the class itself rather than an instance of the class, the calls to other methods, such as aFunction(), do not have access to an instance object—resulting in the missing self error.
The Solution
To resolve this issue, the fromDict method should be modified to create an instance of the class properly. Here’s an updated version of the code that accomplishes this:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Changes
Setting attributes: The loop that sets the attributes iterates over the dictionary items and applies them to the instance (obj), not the class itself.
Returning the object: Finally, instead of returning cls, we return the newly created instance obj.
Advanced Usage: Including Additional Attributes
For more complex scenarios where you wish to handle additional attributes more gracefully, you might consider extending the __init__ method to accept variable keyword arguments (**kwargs). Here’s how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding how to correctly construct a class instance using different methods in Python is essential for effective programming. By clearly distinguishing between class-level attributes and instance-level attributes, you can avoid common pitfalls such as the TypeError we explored. Make sure to always create instances when needed and properly utilize the __init__ method for initialization. This practice will lead you to write more robust Python code.
With these insights into class construction, you can now confidently create class instances using either the __init__ method or a dynamic approach with fromDict. Happy coding!