filmov
tv
Resolving Blazor Server Issues with Nested Dependency Injection Object Scope
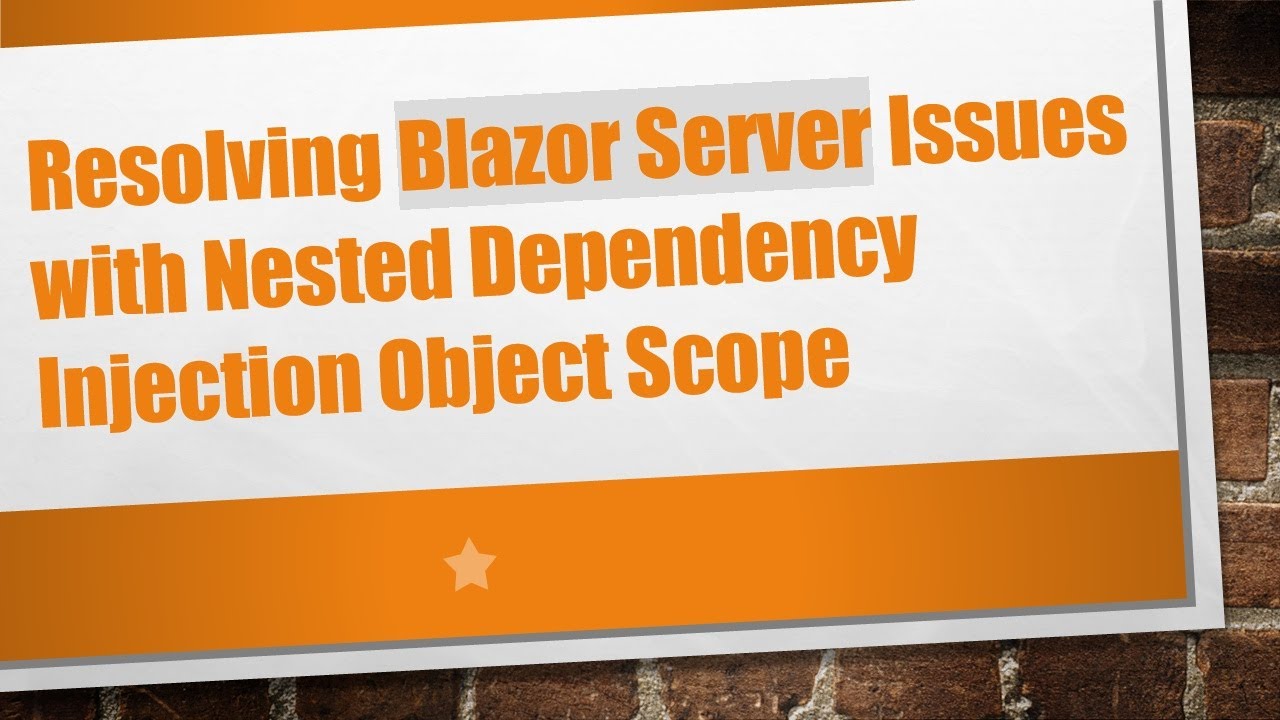
Показать описание
Discover how to effectively manage nested `Dependency Injection` objects' lifetimes in `Blazor Server` applications and avoid common pitfalls related to transient services.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Blazor server issue with nested DI objects' scope
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Managing Dependency Injection in Blazor Server
As a developer working with Blazor, you may find yourself facing issues related to Dependency Injection (DI) and object lifetimes. One common challenge involves managing the scope of nested DI injected objects, especially when those objects implement IDisposable or IAsyncDisposable. This guide will explore how to address these challenges when developing Blazor Server applications.
Understanding the Problem
In your application, you might have a singleton service, DeviceRepositoryGroup, which maintains a dictionary of devices (IMkiiHardwareCommunication). While the singleton itself persists across user sessions, any IMkiiHardwareCommunication instances created as transient services may be disposed when the user's session ends or is refreshed.
Key Points:
Singleton Service: Maintains its instance for the entire application's lifetime.
Transient Service: A new instance of the service is created each time it’s requested. It doesn't keep track of these instances once created.
Session Reset: Refreshing the page (F5) resets the session, leading to the disposal of transient objects.
The disposal of these services leads to a scenario where your device instances become invalid, resulting in disconnections and forcing you to reconnect.
Analyzing the Current Approach
Currently, your code creates IMkiiHardwareCommunication instances inside a loop in a .razor component and adds them to the persistent DeviceRepositoryGroup:
[[See Video to Reveal this Text or Code Snippet]]
Issue:
When the page is refreshed, the original connection is lost because the transient instances are disposed, even though they are still referenced in the DeviceRepositoryGroup.
The Solution: Adjusting Lifetimes and Scope
To resolve these issues, consider the following structured approach:
1. Create Instances When Needed
Instead of relying on transient DI for the IMkiiHardwareCommunication instances that you need to maintain state, create them directly in your service.
2. Implement IDisposable
Implement IDisposable or IAsyncDisposable in DeviceRepositoryGroup to manage the disposal of IMkiiHardwareCommunication instances when they are no longer needed.
Revised Code Example
Here’s how you can implement these concepts:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of this Approach
Instance Control: By creating instances in your service directly, you have control over their lifetime.
Proper Cleanup: Implementing DisposeAsync ensures that all resources are cleaned up appropriately, preventing memory leaks.
Improved Reliability: Your connection status remains intact, as instances are only disposed when managed through the service.
Conclusion
Managing the scope of nested DI objects in Blazor Server can be complex, especially with the nuances of service lifetimes. By creating instances when needed and ensuring proper disposal, you can avoid issues related to transient services and maintain a more stable application.
By applying these principles, you can enhance the robustness of your application and ensure a better user experience. If you encounter further challenges or have specific scenarios in mind, feel free to share!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Blazor server issue with nested DI objects' scope
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Managing Dependency Injection in Blazor Server
As a developer working with Blazor, you may find yourself facing issues related to Dependency Injection (DI) and object lifetimes. One common challenge involves managing the scope of nested DI injected objects, especially when those objects implement IDisposable or IAsyncDisposable. This guide will explore how to address these challenges when developing Blazor Server applications.
Understanding the Problem
In your application, you might have a singleton service, DeviceRepositoryGroup, which maintains a dictionary of devices (IMkiiHardwareCommunication). While the singleton itself persists across user sessions, any IMkiiHardwareCommunication instances created as transient services may be disposed when the user's session ends or is refreshed.
Key Points:
Singleton Service: Maintains its instance for the entire application's lifetime.
Transient Service: A new instance of the service is created each time it’s requested. It doesn't keep track of these instances once created.
Session Reset: Refreshing the page (F5) resets the session, leading to the disposal of transient objects.
The disposal of these services leads to a scenario where your device instances become invalid, resulting in disconnections and forcing you to reconnect.
Analyzing the Current Approach
Currently, your code creates IMkiiHardwareCommunication instances inside a loop in a .razor component and adds them to the persistent DeviceRepositoryGroup:
[[See Video to Reveal this Text or Code Snippet]]
Issue:
When the page is refreshed, the original connection is lost because the transient instances are disposed, even though they are still referenced in the DeviceRepositoryGroup.
The Solution: Adjusting Lifetimes and Scope
To resolve these issues, consider the following structured approach:
1. Create Instances When Needed
Instead of relying on transient DI for the IMkiiHardwareCommunication instances that you need to maintain state, create them directly in your service.
2. Implement IDisposable
Implement IDisposable or IAsyncDisposable in DeviceRepositoryGroup to manage the disposal of IMkiiHardwareCommunication instances when they are no longer needed.
Revised Code Example
Here’s how you can implement these concepts:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of this Approach
Instance Control: By creating instances in your service directly, you have control over their lifetime.
Proper Cleanup: Implementing DisposeAsync ensures that all resources are cleaned up appropriately, preventing memory leaks.
Improved Reliability: Your connection status remains intact, as instances are only disposed when managed through the service.
Conclusion
Managing the scope of nested DI objects in Blazor Server can be complex, especially with the nuances of service lifetimes. By creating instances when needed and ensuring proper disposal, you can avoid issues related to transient services and maintain a more stable application.
By applying these principles, you can enhance the robustness of your application and ensure a better user experience. If you encounter further challenges or have specific scenarios in mind, feel free to share!