filmov
tv
Understanding the Difference Between Function Declarations and Function Expressions in JavaScript
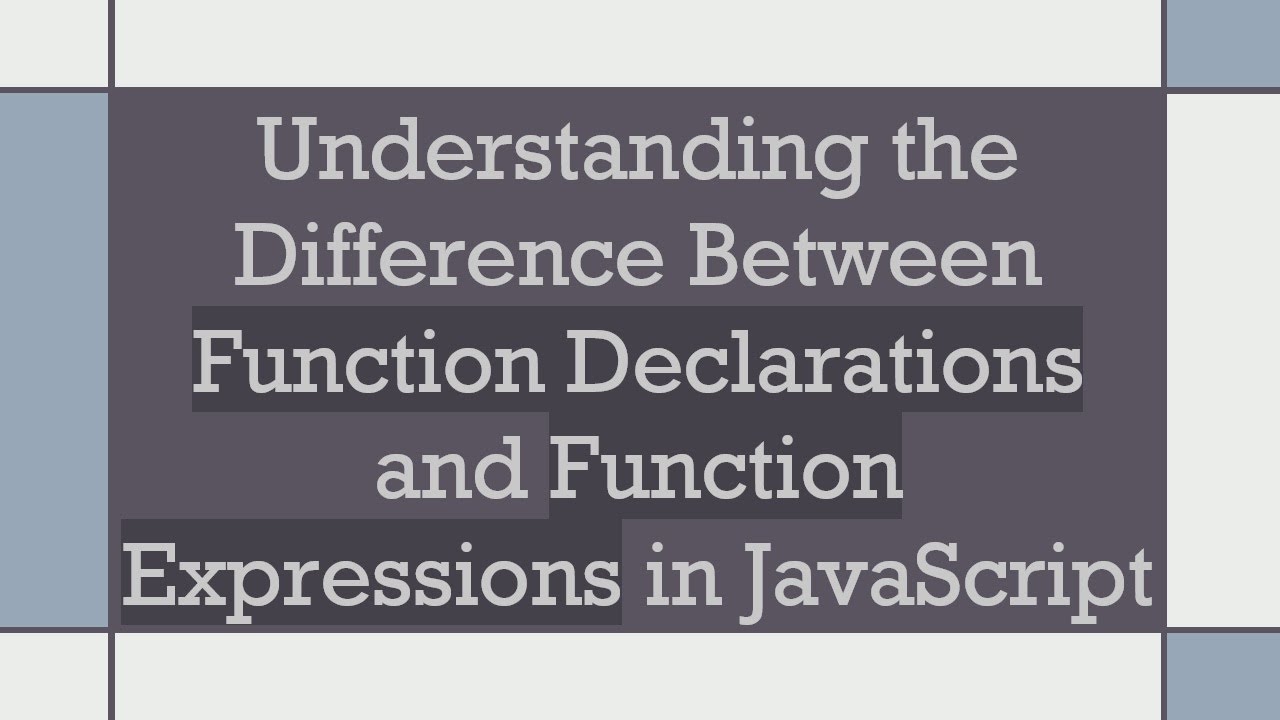
Показать описание
Learn the fundamental distinctions between function declarations and function expressions in JavaScript, and how each can influence your code structure and behavior.
---
Understanding the Difference Between Function Declarations and Function Expressions in JavaScript
When working with JavaScript, understanding how to declare functions is essential for effective coding. Functions are one of the core building blocks of JavaScript, and there are different ways to define them: function declarations and function expressions. Although both methods create functions, they behave differently in important ways.
Function Declarations
A function declaration defines a named function and is hoisted to the top of its containing scope. This means that the function can be used before it is declared in the code. Here is an example of a function declaration:
[[See Video to Reveal this Text or Code Snippet]]
In the example above, the greet function can be called from anywhere within its scope, even before the actual function code appears. This is due to hoisting, a behavior in JavaScript where function declarations are moved to the top of their scope before code execution.
Function Expressions
A function expression involves creating a function and assigning it to a variable. Unlike function declarations, function expressions are not hoisted, which means the function cannot be called before it has been defined in the code. Below is an example of a function expression:
[[See Video to Reveal this Text or Code Snippet]]
In this case, if you try to call greet before the assignment, you will get an error because the variable greet is not defined until that point in the code is executed.
Anonymous vs. Named Function Expressions
Function expressions can either be anonymous or named. The example above is an anonymous function expression because the function has no name. Here is how a named function expression looks:
[[See Video to Reveal this Text or Code Snippet]]
Using a named function expression can make debugging easier because the function name will appear in stack traces.
Key Differences
Hoisting: Function declarations are hoisted to the top of their scope, allowing them to be used before they are defined. Function expressions are not hoisted.
Syntax: Function declarations define a named function, while function expressions involve creating a function that is typically assigned to a variable.
Usage: Function declarations are more suitable for defining utility functions that need to be available throughout the code. Function expressions are ideal for creating functions that are used within more localized scopes.
Summary
Understanding the differences between function declarations and function expressions in JavaScript is crucial for organizing your code efficiently and avoiding common pitfalls. Function declarations offer the benefit of hoisting, making them accessible throughout the entire scope. In contrast, function expressions provide more flexible options, such as anonymous functions and names used for debugging.
Knowing when and how to use each can greatly enhance the readability, maintainability, and behavior of your JavaScript code. Happy coding!
---
Understanding the Difference Between Function Declarations and Function Expressions in JavaScript
When working with JavaScript, understanding how to declare functions is essential for effective coding. Functions are one of the core building blocks of JavaScript, and there are different ways to define them: function declarations and function expressions. Although both methods create functions, they behave differently in important ways.
Function Declarations
A function declaration defines a named function and is hoisted to the top of its containing scope. This means that the function can be used before it is declared in the code. Here is an example of a function declaration:
[[See Video to Reveal this Text or Code Snippet]]
In the example above, the greet function can be called from anywhere within its scope, even before the actual function code appears. This is due to hoisting, a behavior in JavaScript where function declarations are moved to the top of their scope before code execution.
Function Expressions
A function expression involves creating a function and assigning it to a variable. Unlike function declarations, function expressions are not hoisted, which means the function cannot be called before it has been defined in the code. Below is an example of a function expression:
[[See Video to Reveal this Text or Code Snippet]]
In this case, if you try to call greet before the assignment, you will get an error because the variable greet is not defined until that point in the code is executed.
Anonymous vs. Named Function Expressions
Function expressions can either be anonymous or named. The example above is an anonymous function expression because the function has no name. Here is how a named function expression looks:
[[See Video to Reveal this Text or Code Snippet]]
Using a named function expression can make debugging easier because the function name will appear in stack traces.
Key Differences
Hoisting: Function declarations are hoisted to the top of their scope, allowing them to be used before they are defined. Function expressions are not hoisted.
Syntax: Function declarations define a named function, while function expressions involve creating a function that is typically assigned to a variable.
Usage: Function declarations are more suitable for defining utility functions that need to be available throughout the code. Function expressions are ideal for creating functions that are used within more localized scopes.
Summary
Understanding the differences between function declarations and function expressions in JavaScript is crucial for organizing your code efficiently and avoiding common pitfalls. Function declarations offer the benefit of hoisting, making them accessible throughout the entire scope. In contrast, function expressions provide more flexible options, such as anonymous functions and names used for debugging.
Knowing when and how to use each can greatly enhance the readability, maintainability, and behavior of your JavaScript code. Happy coding!