filmov
tv
Find Kth Bit in Nth Binary String - Leetcode 1545 - Python
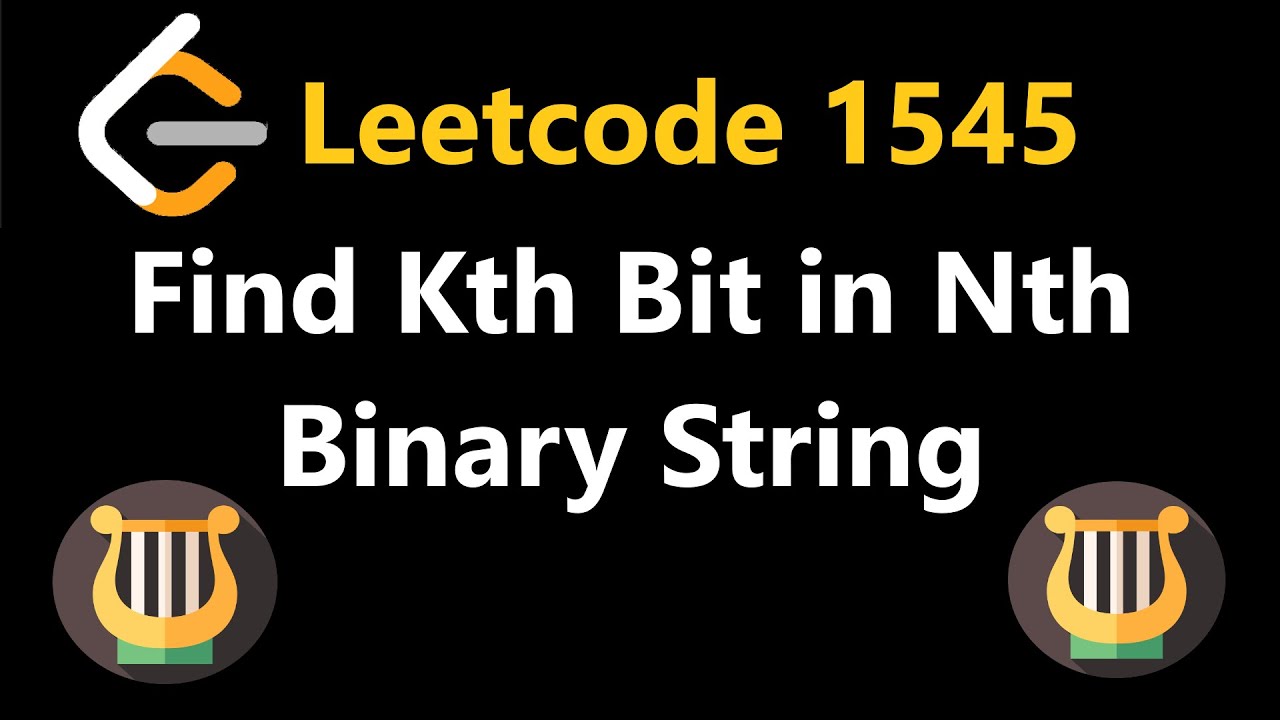
Показать описание
0:00 - Read the problem
0:30 - Drawing Explanation
14:36 - Coding Explanation
18:13 - Coding Explanation 2
leetcode 1545
#neetcode #leetcode #python
Find Kth Bit in Nth Binary String - Leetcode 1545 - Python
Find Kth Bit in Nth Binary String | 4 Methods | Leetcode 1545
Find Kth Bit in Nth Binary String | Detailed Recursion | Dry Run | Leetcode 1545 | codestorywithMIK
LeetCode | Weekly Contest 201 | Problem: Find Kth Bit in Nth Binary String
LeetCode 1545. Find Kth Bit in Nth Binary String - Interview Prep Ep 90
1545. Find Kth Bit in Nth Binary String | Leetcode Daily Challenge | DSA | Java | FAANG
Find Kth Bit in Nth Binary String | Leetcode 1545
1545. Find Kth Bit in Nth Binary String | LeetCode Daily Challenge Today POTD | Recursion | Codefod
Find Kth Bit in Nth Binary String - Leetcode (Python)
Find Kth Bit in Nth Binary String || Recursion 6 || Placement Preparation Series ||Medium ||GFG|
1545. Find Kth Bit in Nth Binary String - Day 19/31 Leetcode October Challenge
LEETCODE 1545. Find Kth Bit in Nth Binary String DETAILED EXPLANATION & Some Bit Manipulation
1545. Find Kth Bit in Nth Binary String| Brute Force| leetcode medium
Leetcode#41. 1545. Find Kth Bit in Nth Binary String. Daily 19th October.
Find Kth Bit in Nth Binary String | LeetCode 1545
LeetCode 1545 - Find Kth Bit in Nth Binary String
1545. Find Kth Bit in Nth Binary String (Leetcode Medium)
1545 Find Kth Bit in Nth Binary String
LeetCode 1545. Find Kth Bit in Nth Binary String
solving leetcode in assembly 1545. Find Kth Bit in Nth Binary String
1545. Find Kth Bit in Nth Binary String | C++ | string | amazon
Part 1 - LeetCode 1545 - Find Kth Bit in Nth Binary String
LeetCode: 1545 Find Kth Bit in Nth Binary String
Find Kth Bit in Nth Binary String | Leetcode (1545) | Recursion | Code kar Befikr
Комментарии