filmov
tv
Explain JVM Architecture | Java 8 Interview Questions 2021 - J04
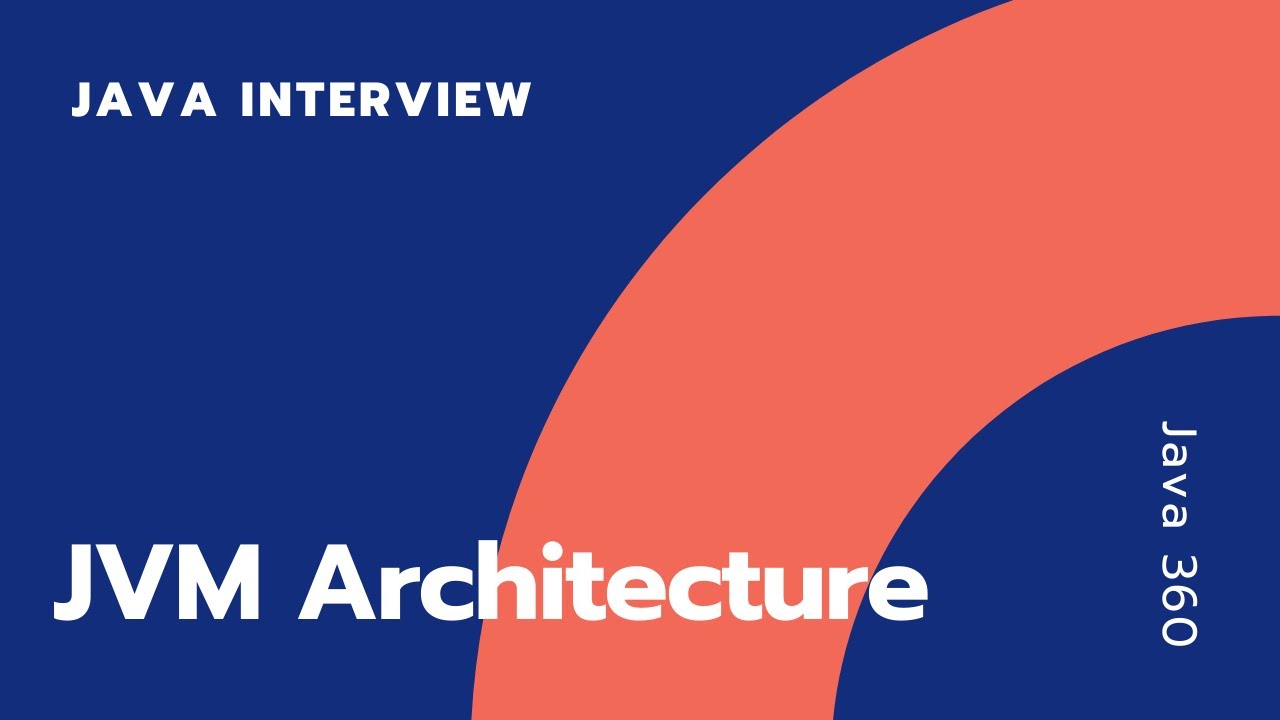
Показать описание
Explain JVM Architecture | Java 8 Interview Questions 2021 - J04
The JVM manages system memory and provides a portable execution environment for Java-based applications
A Virtual Machine is a software implementation of a physical machine The compiler compiles the Java file into a Java .class file, then that .class file is input into the JVM, which loads and executes the class file.
This is the Architecture of the JVM.
As you see in the diagram, the JVM has 3 sub systems namely, class loader sub system, runtime data area and the execution engine.
Classloader is a subsystem of the JVM which is used to load class files. It verifies class files using a bytecode verifier. A class file will only be loaded if it is valid.
The method area is also called the class area. All the class level data such as the run-time constant pool, field, and method data, and the code for methods and constructors, are stored here.
If the memory available in the method area is not sufficient for the program startup, the JVM throws an OutOfMemoryError. The method area is created on the virtual machine start-up, and there is only one method area per JVM.
For example, assume that you have the following class definition:
public class Employee {
private String name;
private int age;
public Employee(String name, int age) {
}
}
In this code example, the field level data such as name and age and the constructor details are loaded into the method area.
Lets see what happens in the Heap area. All the objects and their corresponding instance variables are stored here. This is the run-time data area from which memory for all class instances and arrays is allocated.
For example assume that you are declaring the following instance:
Employee employee = new Employee();
In this code example, an instance of Employee is created and loaded into the heap area.
The heap is created on the virtual machine start-up, and there is only one heap area per JVM.
Since the Method and Heap areas share the same memory for multiple threads, the data stored here is not thread safe.
Whenever a new thread is created in the JVM, a separate runtime stack is also created at the same time. All local variables, method calls, and partial results are stored in the stack area.
If the processing being done in a thread requires a larger stack size than what's available, the JVM throws a StackOverflowError.
For every method call, one entry is made in the stack memory which is called the Stack Frame. When the method call is complete, the Stack Frame is destroyed.
Since the Stack Area is not shared, it is thread safe.
The JVM supports multiple threads at the same time. Each thread has its own PC Register to hold the address of the currently executing JVM instruction. Once the instruction is executed, the PC register is updated with the next instruction.
The JVM contains stacks that support native methods. These methods are written in a language other than the Java, such as C and C++. For every new thread, a separate native method stack is also allocated.
Once the bytecode has been loaded into the main memory, and details are available in the runtime data area, the next step is to run the program. The Execution Engine handles this by executing the code present in each class.
However, before executing the program, the bytecode needs to be converted into machine language instructions. The JVM can use an interpreter or a JIT compiler for the execution engine.
The Garbage Collector (GC) collects and removes unreferenced objects from the heap area. It is the process of reclaiming the runtime unused memory automatically by destroying them.
Garbage collection makes Java memory efficient because because it removes the unreferenced objects from heap memory and makes free space for new objects. It involves two phases:
1. Mark - in this step, the GC identifies the unused objects in memory
2. Sweep - in this step, the GC removes the objects identified during the previous phase
The JVM manages system memory and provides a portable execution environment for Java-based applications
A Virtual Machine is a software implementation of a physical machine The compiler compiles the Java file into a Java .class file, then that .class file is input into the JVM, which loads and executes the class file.
This is the Architecture of the JVM.
As you see in the diagram, the JVM has 3 sub systems namely, class loader sub system, runtime data area and the execution engine.
Classloader is a subsystem of the JVM which is used to load class files. It verifies class files using a bytecode verifier. A class file will only be loaded if it is valid.
The method area is also called the class area. All the class level data such as the run-time constant pool, field, and method data, and the code for methods and constructors, are stored here.
If the memory available in the method area is not sufficient for the program startup, the JVM throws an OutOfMemoryError. The method area is created on the virtual machine start-up, and there is only one method area per JVM.
For example, assume that you have the following class definition:
public class Employee {
private String name;
private int age;
public Employee(String name, int age) {
}
}
In this code example, the field level data such as name and age and the constructor details are loaded into the method area.
Lets see what happens in the Heap area. All the objects and their corresponding instance variables are stored here. This is the run-time data area from which memory for all class instances and arrays is allocated.
For example assume that you are declaring the following instance:
Employee employee = new Employee();
In this code example, an instance of Employee is created and loaded into the heap area.
The heap is created on the virtual machine start-up, and there is only one heap area per JVM.
Since the Method and Heap areas share the same memory for multiple threads, the data stored here is not thread safe.
Whenever a new thread is created in the JVM, a separate runtime stack is also created at the same time. All local variables, method calls, and partial results are stored in the stack area.
If the processing being done in a thread requires a larger stack size than what's available, the JVM throws a StackOverflowError.
For every method call, one entry is made in the stack memory which is called the Stack Frame. When the method call is complete, the Stack Frame is destroyed.
Since the Stack Area is not shared, it is thread safe.
The JVM supports multiple threads at the same time. Each thread has its own PC Register to hold the address of the currently executing JVM instruction. Once the instruction is executed, the PC register is updated with the next instruction.
The JVM contains stacks that support native methods. These methods are written in a language other than the Java, such as C and C++. For every new thread, a separate native method stack is also allocated.
Once the bytecode has been loaded into the main memory, and details are available in the runtime data area, the next step is to run the program. The Execution Engine handles this by executing the code present in each class.
However, before executing the program, the bytecode needs to be converted into machine language instructions. The JVM can use an interpreter or a JIT compiler for the execution engine.
The Garbage Collector (GC) collects and removes unreferenced objects from the heap area. It is the process of reclaiming the runtime unused memory automatically by destroying them.
Garbage collection makes Java memory efficient because because it removes the unreferenced objects from heap memory and makes free space for new objects. It involves two phases:
1. Mark - in this step, the GC identifies the unused objects in memory
2. Sweep - in this step, the GC removes the objects identified during the previous phase
Комментарии