filmov
tv
Find Median from Data Stream - Heap & Priority Queue - Leetcode 295
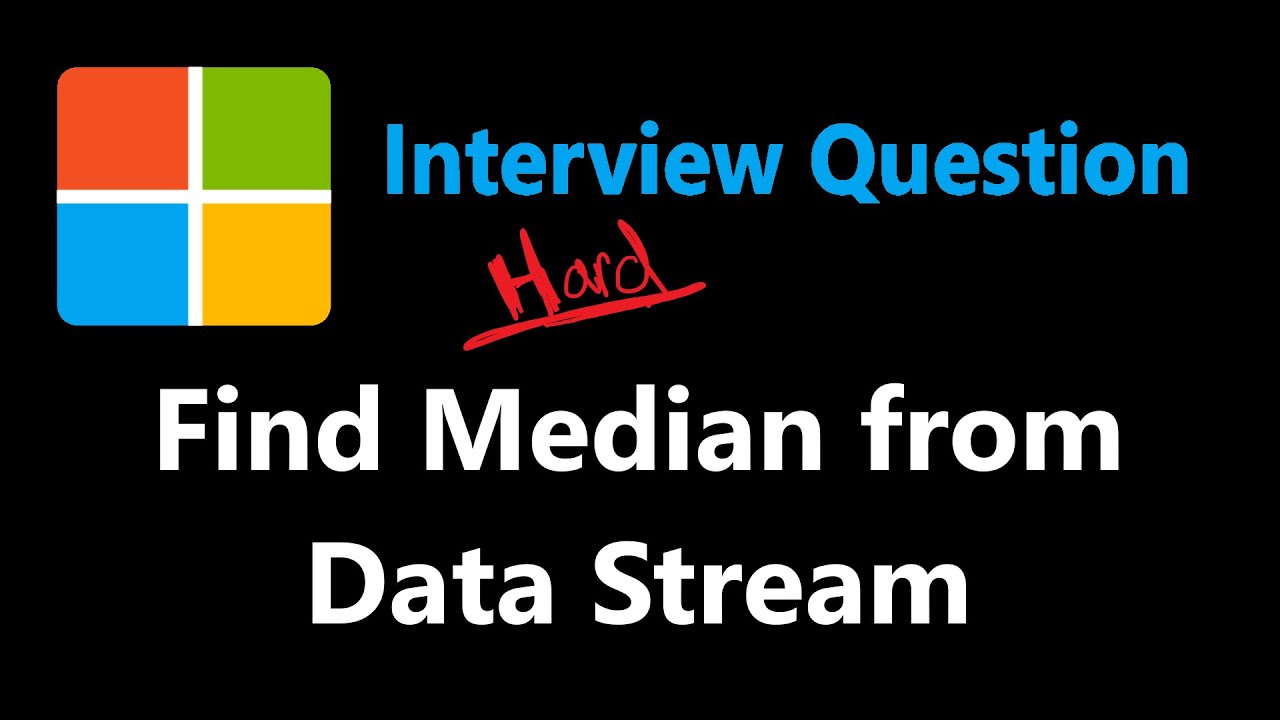
Показать описание
0:00 - Read the problem
1:39 - Drawing Brute Force
5:02 - Drawing Heap Solution
15:15 - Coding Solution
leetcode 295
#median #heap #python
Find Median from Data Stream - Heap & Priority Queue - Leetcode 295
Find Median from Data Stream
Heap - Find Median of Running stream of Integers | Google Interview Question | DSA-One Course #35
Find Median from Data Stream | Google | Amazon | Microsoft | Meta | Explanation | Live Coding
Find Median from Data Stream | Two Heap | Coding Interview
Leetcode - Find Median from Data Stream (Python)
Leetcode 295. Find Median from Data Stream Intuition + Code C++ Example
[Java] Leetcode 295. Find Median from Data Stream [Two Heaps #1]
Find Median From Data Stream - LeetCode 295 - Apple Interview
Median of stream of running integers | Heaps, Priority Queues Application | Explanation from Basics
FIND MEDIAN FROM DATA STREAM (Leetcode) - Code & Whiteboard
How to calculate Median for Grouped Data? | Formula for Median of Grouped Data
FIND MEDIAN FROM DATA STREAM | LEETCODE # 295 | PYTHON TWO HEAPS SOLUTION
Lecture 77: Heaps Hard Interview Questions || Part - 3
295. Find Median from Data Stream - Day 11/31 Leetcode July Challenge
Find Median From Data Stream
LeetCode 295. Find Median from Data Stream Explanation and Solution
Find Median from Data Stream | Leetcode 295 | C++ | Python | Heap | Hard | Approach + Code
Find Median from Data Stream
LeetCode 295. Find Median from Data Stream
Find Median from Data Stream - Design a Data Structure | The Code Mate
E006 : Find median from data stream | Top 100 liked questions | CodeNCode
LeetCode 295. Find Median from Data Stream
295. Find Median from Data Stream
Комментарии