filmov
tv
Python Fundamentals - Python Sets
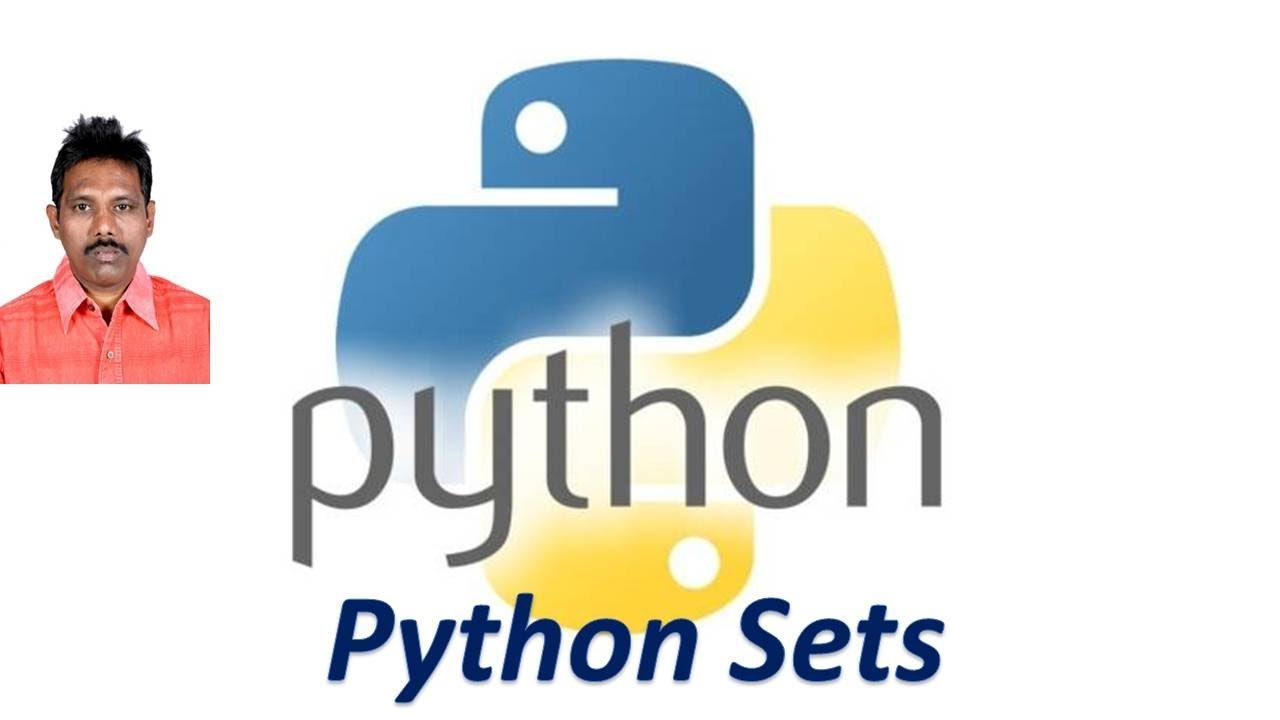
Показать описание
Python Fundamentals - Python Sets
What is Set?
Set in Python is a data structure equivalent to sets in mathematics. It may consist of various elements; the order of elements in a set is undefined.
Python set is a collection which is unordered and unindexed. In Python, sets are written with curly brackets.
Example
Create a Set:
fruits= {"apple", "banana", "cherry"}
print(fruits)
Operations on Python Sets
1) Access Items
We cannot access items in a set by referring to an index, since sets are unordered the items has no index.
But we can loop through the set item
Example 1:
Loop through the set, and print the all values
fruits = {"apple", "banana", "cherry"}
for x in fruits:
print(x)
Example 2:
Check if "banana" is present in the set:
fruits = {"apple", "banana", "cherry"}
print("banana" in fruits)
Note: It will print True/False like result
2) Change Items
Once a set is created, we cannot change its items, but we can add new items.
3) Add Items
i) To add one item to a set use the add() method.
ii) To add more than one item to a set use the update() method.
Example 1:
Add an item to a set, using the add() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
Example 2:
Add multiple items to a set, using the update() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
4) Get the Length of a Set
To determine how many items a set has, use the len() method.
Example
Get the number of items in a set:
fruits = {"apple", "banana", "cherry"}
print(len(fruits))
5) Remove Item
To remove an item in a set, use the remove(), or the discard() method.
Example 1:
Remove "banana" by using the remove() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
-----------------------------------
Example 2:
Remove "banana" by using the discard() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
Note: If the item to remove does not exist, discard() will NOT raise an error.
-------------------------
Example 3:
Remove the last item by using the pop() method:
fruits = {"apple", "banana", "cherry"}
print(x)
print(fruits)
Note: Sets are unordered, so when using the pop() method, you will not know which item that gets removed.
---------------------------------------
Example 4:
The clear() method empties the set:
fruits = {"apple", "banana", "cherry"}
print(fruits)
-----------------------------
Example 5:
The del keyword will delete the set completely:
fruits = {"apple", "banana", "cherry"}
del fruits
print(fruits)
Note: It will show error
6) Join Two Sets
There are several ways to join two or more sets in Python.
You can use the union() method that returns a new set containing all items from both sets, or the update() method that inserts all the items from one set into another:
Example 1:
The union() method returns a new set with all items from both sets:
set1 = {"a", "b" , "c"}
set2 = {1, 2, 3}
print(set3)
--------------------------
Example 2:
The update() method inserts the items in set2 into set1:
set1 = {"a", "b" , "c"}
set2 = {1, 2, 3}
print(set1)
What is Set?
Set in Python is a data structure equivalent to sets in mathematics. It may consist of various elements; the order of elements in a set is undefined.
Python set is a collection which is unordered and unindexed. In Python, sets are written with curly brackets.
Example
Create a Set:
fruits= {"apple", "banana", "cherry"}
print(fruits)
Operations on Python Sets
1) Access Items
We cannot access items in a set by referring to an index, since sets are unordered the items has no index.
But we can loop through the set item
Example 1:
Loop through the set, and print the all values
fruits = {"apple", "banana", "cherry"}
for x in fruits:
print(x)
Example 2:
Check if "banana" is present in the set:
fruits = {"apple", "banana", "cherry"}
print("banana" in fruits)
Note: It will print True/False like result
2) Change Items
Once a set is created, we cannot change its items, but we can add new items.
3) Add Items
i) To add one item to a set use the add() method.
ii) To add more than one item to a set use the update() method.
Example 1:
Add an item to a set, using the add() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
Example 2:
Add multiple items to a set, using the update() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
4) Get the Length of a Set
To determine how many items a set has, use the len() method.
Example
Get the number of items in a set:
fruits = {"apple", "banana", "cherry"}
print(len(fruits))
5) Remove Item
To remove an item in a set, use the remove(), or the discard() method.
Example 1:
Remove "banana" by using the remove() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
-----------------------------------
Example 2:
Remove "banana" by using the discard() method:
fruits = {"apple", "banana", "cherry"}
print(fruits)
Note: If the item to remove does not exist, discard() will NOT raise an error.
-------------------------
Example 3:
Remove the last item by using the pop() method:
fruits = {"apple", "banana", "cherry"}
print(x)
print(fruits)
Note: Sets are unordered, so when using the pop() method, you will not know which item that gets removed.
---------------------------------------
Example 4:
The clear() method empties the set:
fruits = {"apple", "banana", "cherry"}
print(fruits)
-----------------------------
Example 5:
The del keyword will delete the set completely:
fruits = {"apple", "banana", "cherry"}
del fruits
print(fruits)
Note: It will show error
6) Join Two Sets
There are several ways to join two or more sets in Python.
You can use the union() method that returns a new set containing all items from both sets, or the update() method that inserts all the items from one set into another:
Example 1:
The union() method returns a new set with all items from both sets:
set1 = {"a", "b" , "c"}
set2 = {1, 2, 3}
print(set3)
--------------------------
Example 2:
The update() method inserts the items in set2 into set1:
set1 = {"a", "b" , "c"}
set2 = {1, 2, 3}
print(set1)