filmov
tv
Simplifying JTextField KeyListener Integration in Java Swing
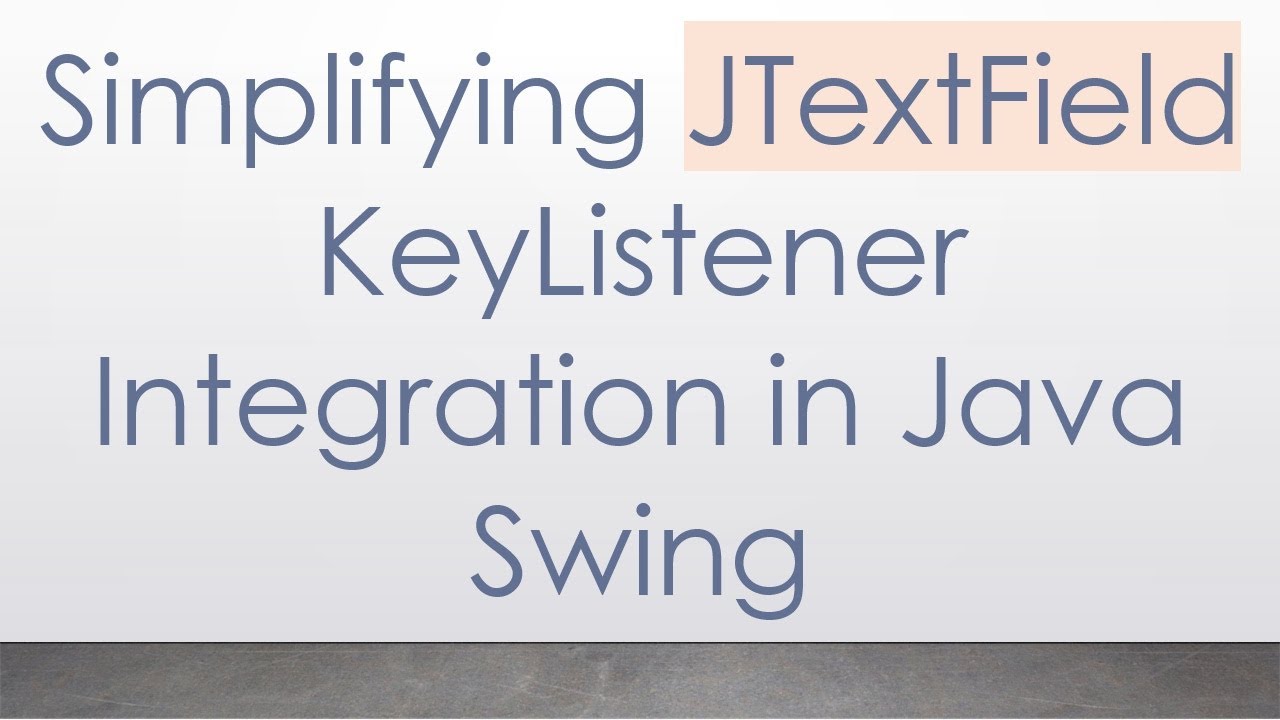
Показать описание
Learn how to efficiently add a `KeyListener` to multiple `JTextFields` in Java Swing without repetitive coding.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to provide addKeyListener() to a multiple JTextFields without writing the same code over and over again
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying JTextField KeyListener Integration in Java Swing
In the world of Java Swing, developers often find themselves faced with the same repetitive tasks over and over again. One common need is setting up KeyListeners for multiple JTextFields. If you've ever encountered the frustration of duplicating the same code for several text fields, you're not alone. You might be wondering if there's a more efficient way to achieve this without compromising on functionality. In this guide, we’ll explore an elegant solution that allows you to provide addKeyListener() to multiple JTextFields without writing the same code repeatedly.
Understanding the Problem
Imagine you have a JTextField in your application where you want to restrict users from entering invalid characters. The initial code snippet you may end up with for a single text field would look something like this:
[[See Video to Reveal this Text or Code Snippet]]
Now, if you have multiple such fields, duplicating this logic for each one can lead to messy and hard-to-maintain code. You might have even tried extending JTextField and implementing KeyListener, only to face issues with your custom class not integrating correctly with your GUI builder.
The Solution: Creating a Custom KeyListener Class
To address this repetitive coding problem, we can extract the key listener logic into a separate class. This will allow us to reuse the same logic across all text fields effortlessly. Let’s break down the steps:
Step 1: Create a Custom KeyListener Class
First, we need to create a separate class that extends KeyAdapter and takes a JTextField as a parameter. Below is how you can create this class:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Instantiate the Custom KeyListener for Each JTextField
After creating the JTextFieldKeyListener class, you can now apply it to any JTextField in your application. Here’s how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of this Approach
Code Reusability: You write the keyTyped logic just once, and you can apply it to as many text fields as you need.
Maintainability: If you need to change the logic, you only do it in one place.
Readability: Your main form’s code becomes cleaner and easier to understand.
Conclusion
In this blog, we’ve tackled the problem of assigning KeyListeners to multiple JTextFields efficiently with minimal redundancy. By encapsulating the listener's logic in its own class, we simplify both the development process and future maintenance. This approach not only saves time but also enhances the overall quality of your code.
By adopting this technique, your Java Swing applications can become more scalable and easier to manage. So go ahead and streamline your Swing projects today!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to provide addKeyListener() to a multiple JTextFields without writing the same code over and over again
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying JTextField KeyListener Integration in Java Swing
In the world of Java Swing, developers often find themselves faced with the same repetitive tasks over and over again. One common need is setting up KeyListeners for multiple JTextFields. If you've ever encountered the frustration of duplicating the same code for several text fields, you're not alone. You might be wondering if there's a more efficient way to achieve this without compromising on functionality. In this guide, we’ll explore an elegant solution that allows you to provide addKeyListener() to multiple JTextFields without writing the same code repeatedly.
Understanding the Problem
Imagine you have a JTextField in your application where you want to restrict users from entering invalid characters. The initial code snippet you may end up with for a single text field would look something like this:
[[See Video to Reveal this Text or Code Snippet]]
Now, if you have multiple such fields, duplicating this logic for each one can lead to messy and hard-to-maintain code. You might have even tried extending JTextField and implementing KeyListener, only to face issues with your custom class not integrating correctly with your GUI builder.
The Solution: Creating a Custom KeyListener Class
To address this repetitive coding problem, we can extract the key listener logic into a separate class. This will allow us to reuse the same logic across all text fields effortlessly. Let’s break down the steps:
Step 1: Create a Custom KeyListener Class
First, we need to create a separate class that extends KeyAdapter and takes a JTextField as a parameter. Below is how you can create this class:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Instantiate the Custom KeyListener for Each JTextField
After creating the JTextFieldKeyListener class, you can now apply it to any JTextField in your application. Here’s how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of this Approach
Code Reusability: You write the keyTyped logic just once, and you can apply it to as many text fields as you need.
Maintainability: If you need to change the logic, you only do it in one place.
Readability: Your main form’s code becomes cleaner and easier to understand.
Conclusion
In this blog, we’ve tackled the problem of assigning KeyListeners to multiple JTextFields efficiently with minimal redundancy. By encapsulating the listener's logic in its own class, we simplify both the development process and future maintenance. This approach not only saves time but also enhances the overall quality of your code.
By adopting this technique, your Java Swing applications can become more scalable and easier to manage. So go ahead and streamline your Swing projects today!