filmov
tv
Solving a JavaScript Array Reduce Interview Question
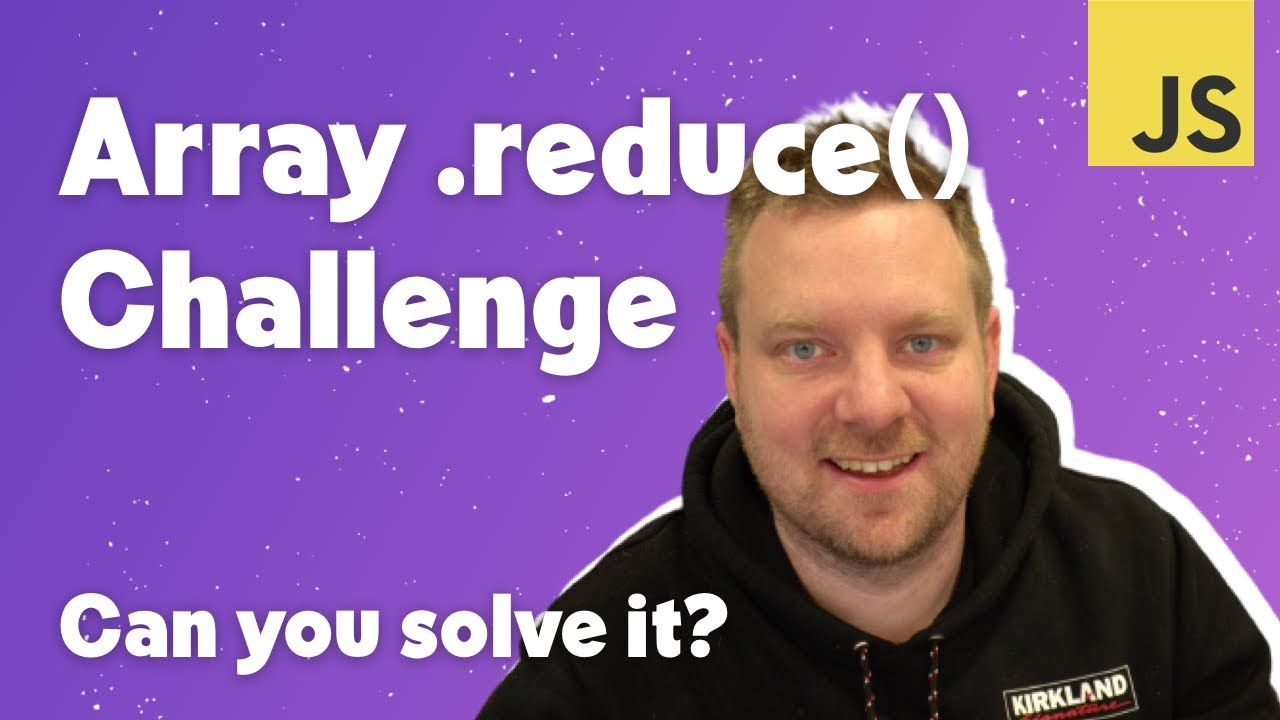
Показать описание
Solving a JavaScript Array Reduce Interview Question
Array Reduce Explained With Examples - JavaScript Tutorial
Array Reduce in 100 seconds
JavaScript Array Reduce Method Practice in 5 Minutes
5 Real Life Examples of Array Reduce in JavaScript
Reduce: Mother of all Javascript Array Methods? (Part 5)
JavaScript Arrays reduce
Javascript Array reduce Explained
Master JavaScript Array Reduce Method In 10 Minutes
Array Reduce Transformation (Transforms) - Leetcode 2626 - JavaScript 30-Day Challenge
HandsOn Coding JavaScript Array Reduce
How to use the array reduce method in Javascript
How to find max number from an array using reduce() in JS
Array Reduce Saves the Day Again - JavaScript CodeWars Challenges #3
Javascript .filter(), .map() and .reduce() on real world examples
How to group with the Array reduce method in Javascript
Javascript Array Reduce (examples with object & array)
Solving an Array Reduce Interview Question - in PHP
LeetCode 30 Days of JavaScript: Array Reduce Transformation
How To Use Reduce In JavaScript - Array.prototype.reduce
Array Iteration: 8 Methods - map, filter, reduce, some, every, find, findIndex, forEach
Array reduce explained
2626. Array Reduce Transformation - Day 6/30 Leetcode Javascript Challenge
Can you solve this tricky JavaScript Array problem?
Комментарии