filmov
tv
Evaluate Reverse Polish Notation - Leetcode 150 - Python
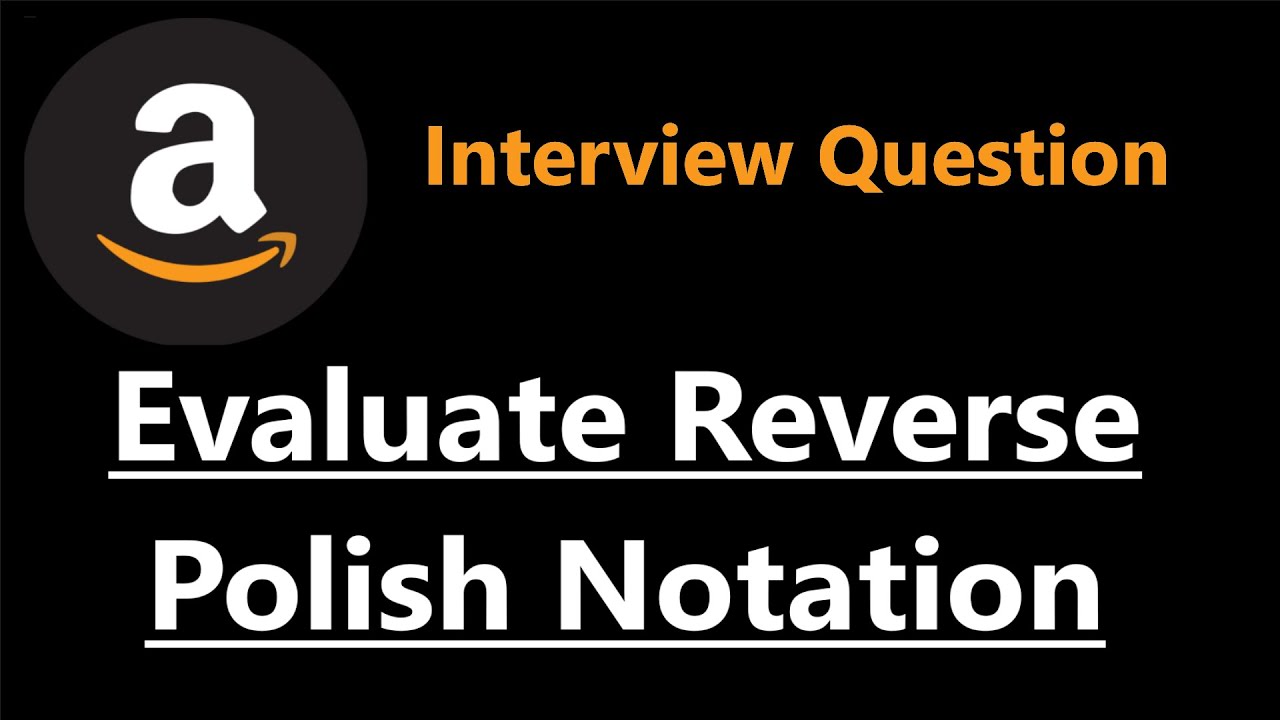
Показать описание
0:00 - Read the problem
1:25 - Drawing Explanation
5:47 - Coding Explanation
leetcode 150
#amazon #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Evaluate Reverse Polish Notation - Leetcode 150 - Python
Evaluate Reverse Polish Notation (RPN) - Leetcode 150 - Stacks (Python)
Reverse Polish Notation: Types of Mathematical Notations & Using A Stack To Solve RPN Expression...
This is one of my Favorite Coding Questions! | Evaluate Reverse Polish Notation - Leetcode 150
Leetcode - Evaluate Reverse Polish Notation (Python)
Evaluate Reverse Polish Notation | 2 Approaches | Leetcode 150
Leetcode 150. Evaluate Reverse Polish Notation || Code + Explanation + Example Walkthrough
Leetcode 150 - Evaluate Reverse Polish Notation
38. AQA A Level (7517) SLR5 - 4.3.3 Reverse Polish notation Part 1
Evaluate Reverse Polish Notation | Решение на Python | LeetCode 150
LeetCode Interview Problem - Reverse Polish Notation
150. Evaluate Reverse Polish Notation | Stack
Evaluate Reverse Polish Notation | Leetcode 150 | Live coding session
Evaluate Reverse Polish Notation | Leetcode Example Stack in Data structure & Algorithms Hello W...
EVALUATE REVERSE POLISH NOTATION | LEETCODE # 150 | PYTHON STACK SOLUTION
What is Reverse Polish Notation (AKA Postfix Notation)? Why is it Important?
Evaluate Reverse Polish Notation - LeetCode 150 - Python
Evaluate Reverse Polish Notation @ Алгосики для самых маленьких, s1e4 / Стеки...
Evaluate Reverse Polish Notation | Leetcode 150 | Stack
150. Evaluate Reverse Polish Notation || Java || Leetcode || Hindi
Leetcode Çözümleri - Evaluate Reverse Polish Notation
Leetcode | 150. Evaluate Reverse Polish Notation | Medium | Java Solution
Reverse Polish Notation - Evaluating RPN expressions
Evaluate A Reverse Polish Notation Equation With JavaScript
Комментарии