filmov
tv
Optimizing the Greedy Knapsack Algorithm for Better Performance in Python
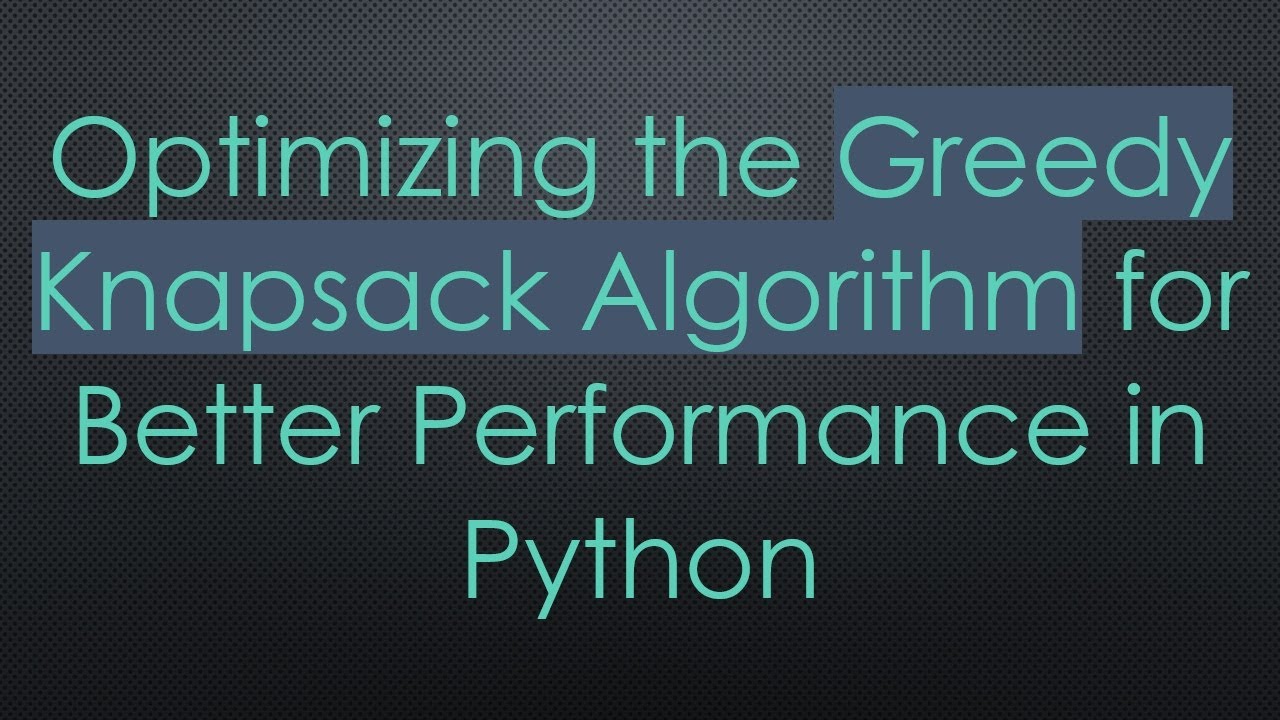
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Discover techniques to enhance the performance of the greedy knapsack algorithm in Python, improving efficiency and solution quality.
---
Optimizing the Greedy Knapsack Algorithm for Better Performance in Python
The knapsack problem is a classic optimization challenge that many computer scientists and programmers face. The objective is simple yet complex: given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is within a given limit and the total value is as large as possible. One popular approach to solving this problem is the greedy algorithm.
What is the Greedy Knapsack Algorithm?
The greedy knapsack algorithm makes a locally optimal choice at each stage with the hope of finding a global optimum. In this context, it sorts the items based on some heuristic, usually the value-to-weight ratio, and then picks items in that order until it can't pick any more without exceeding the weight limit.
Basic Greedy Algorithm in Python
[[See Video to Reveal this Text or Code Snippet]]
Enhancing the Greedy Algorithm's Performance
While the greedy algorithm is straightforward and efficient in many cases, there are several optimizations and techniques that can be applied to improve its performance and the quality of the solution.
Pre-Sorting
In the initial implementation, items are sorted by their value-to-weight ratio. This pre-sorting can be optimized by using more efficient data structures or sorting algorithms.
Limiting Item Count
If the number of items, n, is very large, it can be computationally expensive to sort all of them. Consider applying a heuristic to limit the number of items processed, such as only considering items that have a value-to-weight ratio above a certain threshold.
Using a Priority Queue
For large datasets, utilize a priority queue (heap) to manage items more efficiently while maintaining quick access to the next item with the highest value-to-weight ratio.
Improved Python Implementation
[[See Video to Reveal this Text or Code Snippet]]
Dynamic Programming (For Comparison)
Though not a greedy method, consider comparing the greedy approach with a dynamic programming solution to understand performance trade-offs.
Conclusion
Optimizing the greedy knapsack algorithm involves careful selection and management of items based on their value-to-weight ratio. By leveraging efficient sorting methods, priority queues, and possibly dynamic programming, you can significantly enhance the algorithm's performance. Understanding these tactics not only refines your algorithm but also improves your overall problem-solving skills in computational tasks.
Try these techniques with your data, and experience the difference they make in solving the knapsack problem efficiently and effectively. Happy coding!
---
Summary: Discover techniques to enhance the performance of the greedy knapsack algorithm in Python, improving efficiency and solution quality.
---
Optimizing the Greedy Knapsack Algorithm for Better Performance in Python
The knapsack problem is a classic optimization challenge that many computer scientists and programmers face. The objective is simple yet complex: given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is within a given limit and the total value is as large as possible. One popular approach to solving this problem is the greedy algorithm.
What is the Greedy Knapsack Algorithm?
The greedy knapsack algorithm makes a locally optimal choice at each stage with the hope of finding a global optimum. In this context, it sorts the items based on some heuristic, usually the value-to-weight ratio, and then picks items in that order until it can't pick any more without exceeding the weight limit.
Basic Greedy Algorithm in Python
[[See Video to Reveal this Text or Code Snippet]]
Enhancing the Greedy Algorithm's Performance
While the greedy algorithm is straightforward and efficient in many cases, there are several optimizations and techniques that can be applied to improve its performance and the quality of the solution.
Pre-Sorting
In the initial implementation, items are sorted by their value-to-weight ratio. This pre-sorting can be optimized by using more efficient data structures or sorting algorithms.
Limiting Item Count
If the number of items, n, is very large, it can be computationally expensive to sort all of them. Consider applying a heuristic to limit the number of items processed, such as only considering items that have a value-to-weight ratio above a certain threshold.
Using a Priority Queue
For large datasets, utilize a priority queue (heap) to manage items more efficiently while maintaining quick access to the next item with the highest value-to-weight ratio.
Improved Python Implementation
[[See Video to Reveal this Text or Code Snippet]]
Dynamic Programming (For Comparison)
Though not a greedy method, consider comparing the greedy approach with a dynamic programming solution to understand performance trade-offs.
Conclusion
Optimizing the greedy knapsack algorithm involves careful selection and management of items based on their value-to-weight ratio. By leveraging efficient sorting methods, priority queues, and possibly dynamic programming, you can significantly enhance the algorithm's performance. Understanding these tactics not only refines your algorithm but also improves your overall problem-solving skills in computational tasks.
Try these techniques with your data, and experience the difference they make in solving the knapsack problem efficiently and effectively. Happy coding!