filmov
tv
Solving the NullReferenceException When Adding Objects to a List in Unity C#
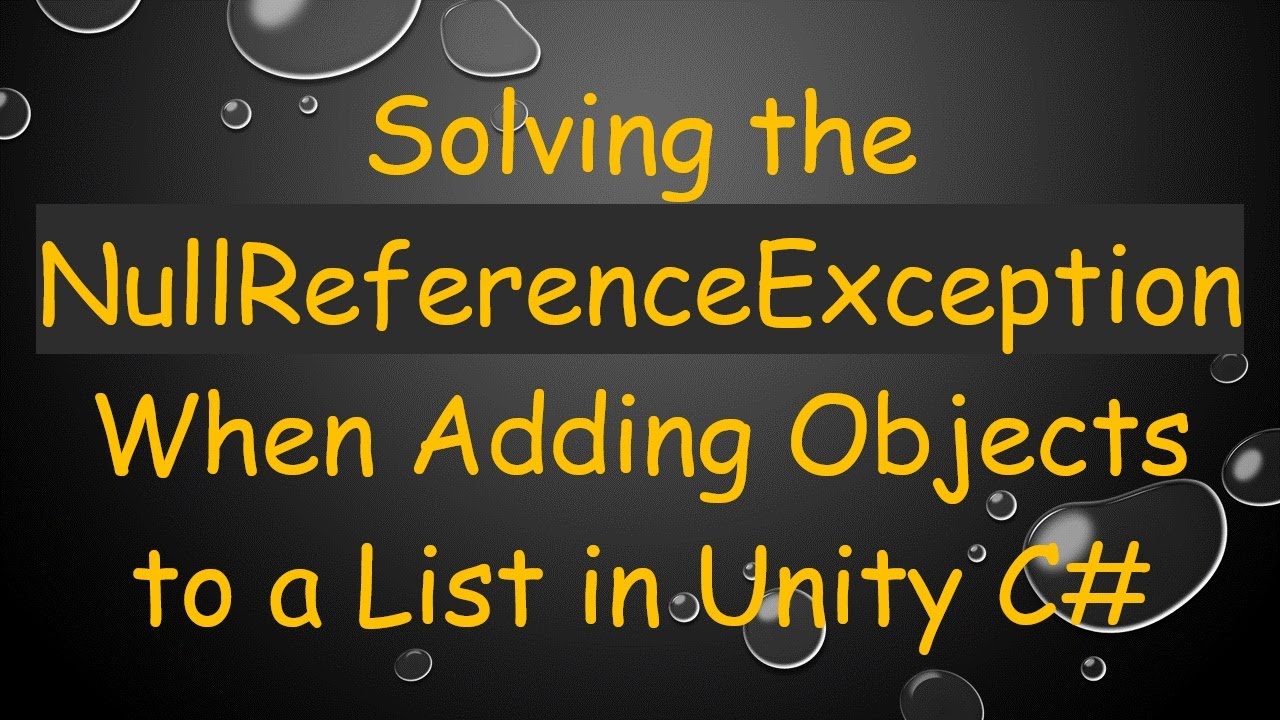
Показать описание
A comprehensive guide on fixing the `NullReferenceException` in Unity when trying to add objects to a List in C#. Learn how to initialize your List correctly for smooth game development.
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: unity C# null reference exception when trying to add object to list
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the NullReferenceException When Adding Objects to a List in Unity C#
If you're a newcomer to Unity and C#, encountering a NullReferenceException can be quite the hurdle. One common scenario arises when you're trying to add an object to a list, but for some reason, your code indicates that the list doesn't exist. In this guide, we'll explore a specific case where a developer is trying to add an object named newTask to a list called builtTasks, but receives a NullReferenceException.
The Problem
In the provided code snippet, the error occurs on the line where the new task is being added to the builtTasks list:
[[See Video to Reveal this Text or Code Snippet]]
This line throws a NullReferenceException. Upon inspecting the code, it's clear that the check for builtTasks reveals it is, in fact, null. When you attempt to call the Add method on a null object, the runtime throws this exception because it doesn't know what to do with a method call on a null reference.
Understanding the Code Structure
To better understand and fix the issue, let’s analyze the relevant code sections:
Class Definitions
The main class looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Here, both builtTasks and runningTasks are defined as static lists but are not initialized. This means they start as null.
Start Method
In the Start() method, the following check is made:
[[See Video to Reveal this Text or Code Snippet]]
When this condition is true, it indicates that the code expects builtTasks to exist but hasn't been explicitly created yet.
The Solution: Initializing the List
To fix the issue, you need to initiate your builtTasks list before trying to use it. This can be done in two possible ways:
Option 1: Initialize During Declaration
You can initialize builtTasks directly during its declaration:
[[See Video to Reveal this Text or Code Snippet]]
This way, as soon as the class is loaded, the list will be ready to use.
Option 2: Initialize Within the Start Method
Alternatively, you can initialize the list inside the Start() method:
[[See Video to Reveal this Text or Code Snippet]]
Both methods will effectively eliminate the NullReferenceException as you’ll have a valid list to work with.
Best Practices
While addressing this issue, consider the following best practices:
Use [SerializeField]: Instead of making fields public, use [SerializeField] to expose them to Unity's inspector while keeping them private. This promotes encapsulation and indicates that Unity handles these fields.
[[See Video to Reveal this Text or Code Snippet]]
Avoid Static Lists: Static members do not have the same lifecycle as instance members. If possible, review your architecture to avoid using static lists unless necessary.
Conclusion
In summary, encountering a NullReferenceException for a list in Unity is typically due to the list not being initialized before use. Always ensure your lists are properly instantiated to avoid these runtime errors. By following the outlined steps, you should be able to manage your lists efficiently and keep your game development process smooth.
If you have any questions or need further clarification, feel free to ask in the comments below!
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: unity C# null reference exception when trying to add object to list
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the NullReferenceException When Adding Objects to a List in Unity C#
If you're a newcomer to Unity and C#, encountering a NullReferenceException can be quite the hurdle. One common scenario arises when you're trying to add an object to a list, but for some reason, your code indicates that the list doesn't exist. In this guide, we'll explore a specific case where a developer is trying to add an object named newTask to a list called builtTasks, but receives a NullReferenceException.
The Problem
In the provided code snippet, the error occurs on the line where the new task is being added to the builtTasks list:
[[See Video to Reveal this Text or Code Snippet]]
This line throws a NullReferenceException. Upon inspecting the code, it's clear that the check for builtTasks reveals it is, in fact, null. When you attempt to call the Add method on a null object, the runtime throws this exception because it doesn't know what to do with a method call on a null reference.
Understanding the Code Structure
To better understand and fix the issue, let’s analyze the relevant code sections:
Class Definitions
The main class looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Here, both builtTasks and runningTasks are defined as static lists but are not initialized. This means they start as null.
Start Method
In the Start() method, the following check is made:
[[See Video to Reveal this Text or Code Snippet]]
When this condition is true, it indicates that the code expects builtTasks to exist but hasn't been explicitly created yet.
The Solution: Initializing the List
To fix the issue, you need to initiate your builtTasks list before trying to use it. This can be done in two possible ways:
Option 1: Initialize During Declaration
You can initialize builtTasks directly during its declaration:
[[See Video to Reveal this Text or Code Snippet]]
This way, as soon as the class is loaded, the list will be ready to use.
Option 2: Initialize Within the Start Method
Alternatively, you can initialize the list inside the Start() method:
[[See Video to Reveal this Text or Code Snippet]]
Both methods will effectively eliminate the NullReferenceException as you’ll have a valid list to work with.
Best Practices
While addressing this issue, consider the following best practices:
Use [SerializeField]: Instead of making fields public, use [SerializeField] to expose them to Unity's inspector while keeping them private. This promotes encapsulation and indicates that Unity handles these fields.
[[See Video to Reveal this Text or Code Snippet]]
Avoid Static Lists: Static members do not have the same lifecycle as instance members. If possible, review your architecture to avoid using static lists unless necessary.
Conclusion
In summary, encountering a NullReferenceException for a list in Unity is typically due to the list not being initialized before use. Always ensure your lists are properly instantiated to avoid these runtime errors. By following the outlined steps, you should be able to manage your lists efficiently and keep your game development process smooth.
If you have any questions or need further clarification, feel free to ask in the comments below!