filmov
tv
Majority Element | GeeksforGeeks
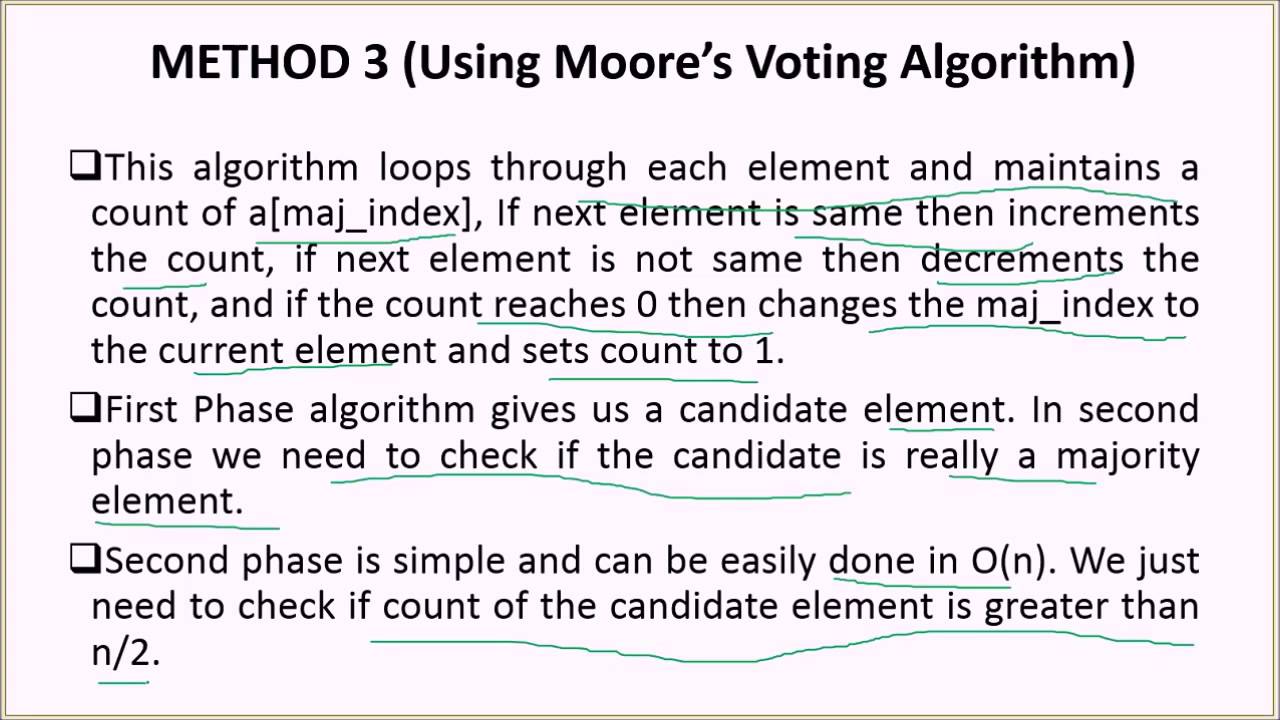
Показать описание
This video is contributed by Harshit Jain.
Majority Element | GeeksforGeeks
Solving Arrays Questions | Majority element | GeeksForGeeks | Nishant Chahar Ep-17
Majority Element | Problem of the Day-1/09/21 | Siddharth Hazra | GeeksforGeeks Practice
Majority Element _ geeksforgeeks
GeeksforGeeks Cheatcode Book - Easy Problems-Topic:Array ||Q2: Majority Element || Geeks Girl
Majority element - Geeks for Geeks, LeetCode
Majority Element - GeeksforGeeks - Must Do Interview Preparation - Arrays - Java
Majority Element | GeeksforGeeks | Solution | Java
Majority Element | Leetcode | C++ | Java | Brute-Better-Optimal | Moore's Voting Algorithm
Majority Element (LeetCode 169) | Full solution with 4 different methods | Interview Essential
Majority element | Leetcode #169
Majority Element
Majority Element in an array (Algorithm)
Majority Element || GeeksForGeeks || LeetCode 169 || 2 Approach Discussion || 21 Feb.
Majority Element Hashmap Solution || LeetCode 169 || Google Software Engineering Interview Question
Majority Element || GeeksForGeeks || LeetCode 169 || Efficient Approach Discussion || 21 Feb.
DSA Placement Series: Mastering Majority Element & Count N/K Occurrence | GeeksforGeeks |Code Cr...
Majority Element | DSA | MAANG | Java | Hindi
MAJORITY ELEMENT: Arrays [L - 1.9] | Java Programming | Programming Questions | Placements
Majority Elements in an Array | Moore's Voting Algorithm | DSA @GeeksforGeeksVideos @LoveBabbar...
Find Majority Element in an Array
Majority Element problem - Python code | Frequently asked coding interview and exam question
Majority Element Range Queries Tutorial
3 Tips I’ve learned after 2000 hours of Leetcode
Комментарии