filmov
tv
How to Return Two Things from a C or C++ Function
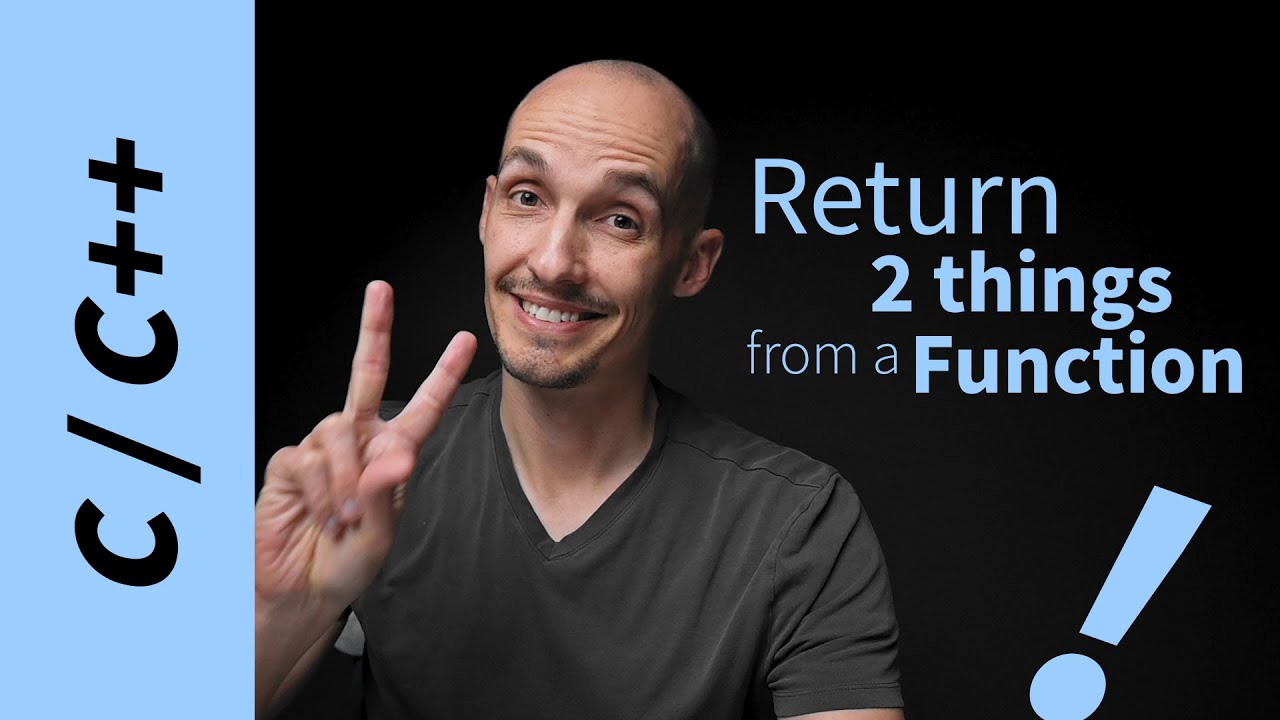
Показать описание
---
How to Return Two Things from a Function (C/C++) // New C and C++ programmers often wonder how to return multiple values from a single function. This video will give you some options. You can return values through pointer or reference arguments, and you can return structs and classes.
Related videos:
***
Welcome! I post videos that help you learn to program and become a more confident software developer. I cover beginner-to-advanced systems topics ranging from network programming, threads, processes, operating systems, embedded systems and others. My goal is to help you get under-the-hood and better understand how computers work and how you can use them to become stronger students and more capable professional developers.
About me: I'm a computer scientist, electrical engineer, researcher, and teacher. I specialize in embedded systems, mobile computing, sensor networks, and the Internet of Things. I teach systems and networking courses at Clemson University, where I also lead the PERSIST research lab.
More about me and what I do:
To Support the Channel:
+ like, subscribe, spread the word
How to Return Two Things from a Function (C/C++) // New C and C++ programmers often wonder how to return multiple values from a single function. This video will give you some options. You can return values through pointer or reference arguments, and you can return structs and classes.
Related videos:
***
Welcome! I post videos that help you learn to program and become a more confident software developer. I cover beginner-to-advanced systems topics ranging from network programming, threads, processes, operating systems, embedded systems and others. My goal is to help you get under-the-hood and better understand how computers work and how you can use them to become stronger students and more capable professional developers.
About me: I'm a computer scientist, electrical engineer, researcher, and teacher. I specialize in embedded systems, mobile computing, sensor networks, and the Internet of Things. I teach systems and networking courses at Clemson University, where I also lead the PERSIST research lab.
More about me and what I do:
To Support the Channel:
+ like, subscribe, spread the word
Комментарии