filmov
tv
Solving the: Variable Not Changing from its Initial Value in JavaScript
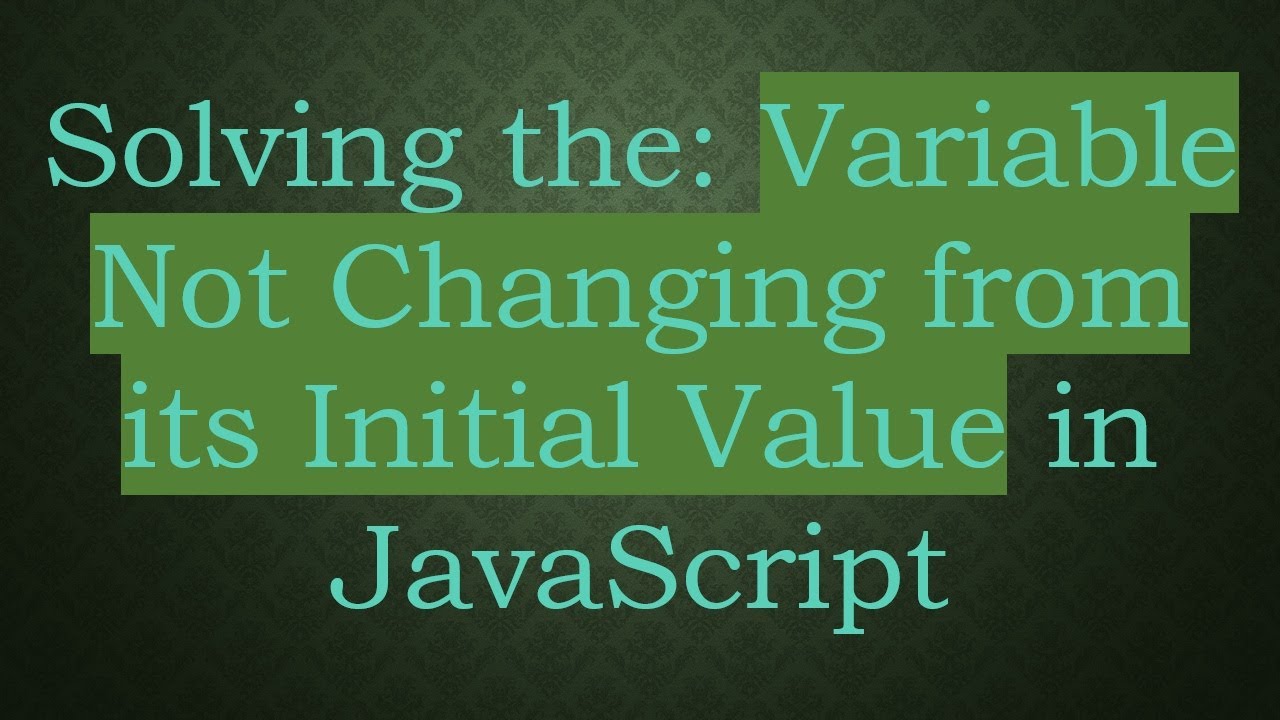
Показать описание
Uncover the reason behind a JavaScript variable not updating and learn how to solve it with simple code adjustments. Perfect for developers looking for practical solutions to common issues!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Variable not changing from its initial value when it outputs in the result
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the: Variable Not Changing from its Initial Value in JavaScript
If you've encountered the frustrating issue where a variable in JavaScript isn’t updating as expected, you're not alone. This can be particularly baffling if you’ve tried multiple fixes but still see the same initial value in your output. In this guide, we'll walk through the problem and reveal how to effectively solve it.
The Problem
In a sample code snippet, a developer struggles with a variable named odEv that is intended to change based on whether a number is odd or even. Despite expectations, the variable retains its initial placeholder value 'T' when output is generated. Here's a brief overview of the situation:
The developer declares odEv globally and attempts to set its value in a function named workingOdd().
When buttons are pressed to simulate a selection, the output always displays that initial value.
Why is This Happening?
The crux of the issue lies in variable scope. In JavaScript, variables can be declared globally or locally. When a variable is declared within a function using var, let, or const, it becomes local to that function. Hence, any changes made to this local variable won't affect the global variable of the same name.
Based on the developer's implementation:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To solve this problem, the developer needs to ensure that they are working with the global variable odEv instead of shadowing it with a local declaration. Here’s how to implement this fix:
Step-by-Step Solution
Remove Local Declaration: Eliminate the var odEv; line from the workingOdd function definition.
From this:
[[See Video to Reveal this Text or Code Snippet]]
To this:
[[See Video to Reveal this Text or Code Snippet]]
Test the Changes: After making this adjustment, test the output again by pressing the buttons. You should now see the odEv variable reflecting the correct 'Ev' or 'Od' value based on whether the input number is even or odd.
Conclusion
Variable scope can often catch developers off guard, especially when juggling global and local variables. By simply removing the local declaration of odEv in your function, you can ensure that the global variable is updated correctly.
Next time you face a similarly puzzling issue in your JavaScript code, remember to check your variable scopes! If you have any further questions or need more assistance, feel free to reach out in the comments below.
Stay tuned for more insights and tips on navigating coding challenges!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Variable not changing from its initial value when it outputs in the result
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the: Variable Not Changing from its Initial Value in JavaScript
If you've encountered the frustrating issue where a variable in JavaScript isn’t updating as expected, you're not alone. This can be particularly baffling if you’ve tried multiple fixes but still see the same initial value in your output. In this guide, we'll walk through the problem and reveal how to effectively solve it.
The Problem
In a sample code snippet, a developer struggles with a variable named odEv that is intended to change based on whether a number is odd or even. Despite expectations, the variable retains its initial placeholder value 'T' when output is generated. Here's a brief overview of the situation:
The developer declares odEv globally and attempts to set its value in a function named workingOdd().
When buttons are pressed to simulate a selection, the output always displays that initial value.
Why is This Happening?
The crux of the issue lies in variable scope. In JavaScript, variables can be declared globally or locally. When a variable is declared within a function using var, let, or const, it becomes local to that function. Hence, any changes made to this local variable won't affect the global variable of the same name.
Based on the developer's implementation:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To solve this problem, the developer needs to ensure that they are working with the global variable odEv instead of shadowing it with a local declaration. Here’s how to implement this fix:
Step-by-Step Solution
Remove Local Declaration: Eliminate the var odEv; line from the workingOdd function definition.
From this:
[[See Video to Reveal this Text or Code Snippet]]
To this:
[[See Video to Reveal this Text or Code Snippet]]
Test the Changes: After making this adjustment, test the output again by pressing the buttons. You should now see the odEv variable reflecting the correct 'Ev' or 'Od' value based on whether the input number is even or odd.
Conclusion
Variable scope can often catch developers off guard, especially when juggling global and local variables. By simply removing the local declaration of odEv in your function, you can ensure that the global variable is updated correctly.
Next time you face a similarly puzzling issue in your JavaScript code, remember to check your variable scopes! If you have any further questions or need more assistance, feel free to reach out in the comments below.
Stay tuned for more insights and tips on navigating coding challenges!