filmov
tv
Loops in Java (Exercise 1)
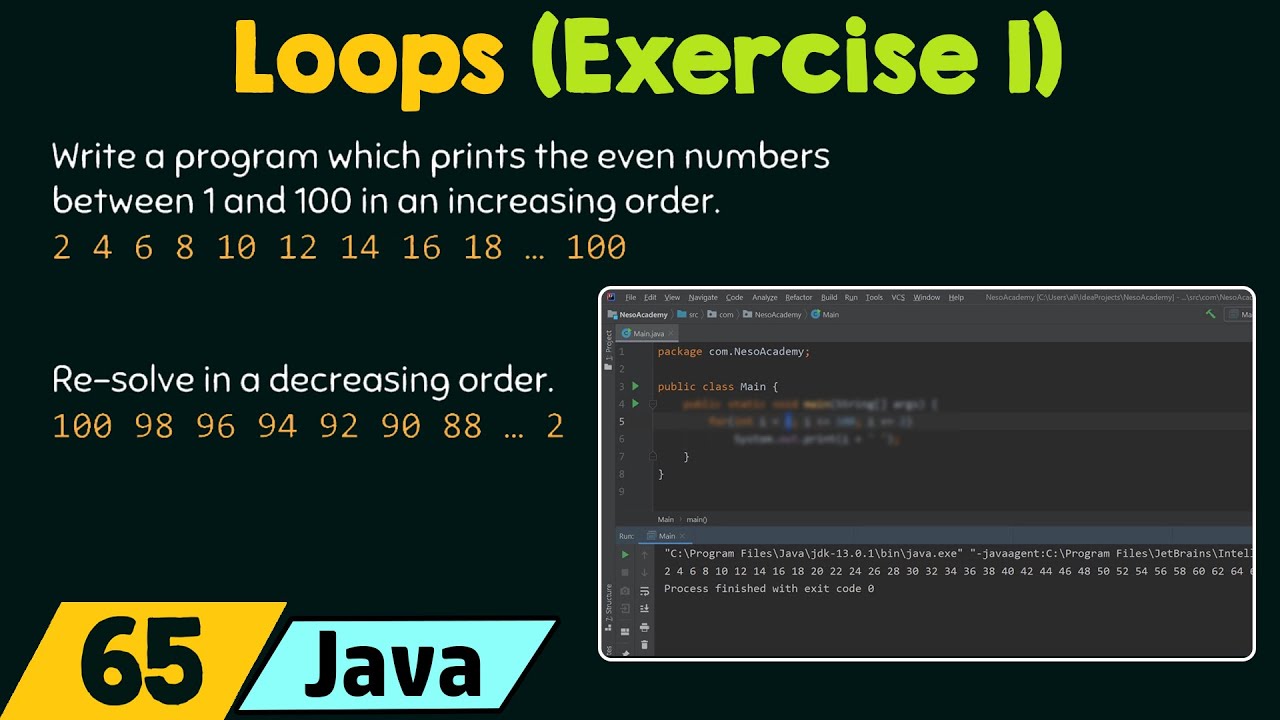
Показать описание
Java Programming: Programming Question on Loops in Java Programming
Topics Discussed:
1. Printing the even numbers between 1 to 100 with the help of loops.
2. Printing the odd numbers between 1 to 100 with the help of loops.
Music:
Axol x Alex Skrindo - You [NCS Release]
#JavaByNeso #JavaProgramming #Loops
Topics Discussed:
1. Printing the even numbers between 1 to 100 with the help of loops.
2. Printing the odd numbers between 1 to 100 with the help of loops.
Music:
Axol x Alex Skrindo - You [NCS Release]
#JavaByNeso #JavaProgramming #Loops
Loops in Java (Exercise 1)
16x - Learn Java 'for' Loops - Exercise 1
Java Exercise 1 - Basic operations in Java [Java 101]
Loops in Java (Exercise 11)
Programming 1 Java - 4.4 Method and Loops Exercise
Exercise 1 Week 3 - Java Tutorial Multiple Strings While Loop
Programming 1 Java - 4.4 Method and Loops Exercise
Programming 1 Java - 3.8 Nested Loops Exercise 1
Loops in Java (Exercise 12)
Loops in Java (Exercise 7)
Java Lecture 10: An exercise on Java for-loops with if-else inside
#014 [JAVA] - Questions and Exercises in Loops
Nested Loops in Java
Loops in Java (Exercise 6)
Loops in Java (Exercise 4)
Loops in Java (Exercise 13)
Learn Java Programming - Exercise 11x - Java Do-While Loop
❌ DON'T use a for loop like this for multiple Lists in Python!!!
07x - Learn Java - Exercise 1 - Compiling Java Code
The For Loop in Java
While Loop Java Tutorial #37
Loops in Java (Exercise 2)
Loops in Java (Exercise 5)
Loops in Java (Exercise 3)
Комментарии